import pygame, sys
import os
import random
import cv2
import mediapipe as mp
import pyautogui
screenWidth, screenHeight = pyautogui.size()
mp_drawing = mp.solutions.drawing_utils
mp_hands = mp.solutions.hands
hands = mp_hands.Hands(
static_image_mode=False,
max_num_hands=2,
min_detection_confidence=0.75,
min_tracking_confidence=0.75)
player_lives = 3 # keep track of lives
score = 0 # keeps track of score
fruits = ['melon', 'orange', 'pomegranate', 'guava', 'bomb'] # entities in the game
# initialize pygame and create window
WIDTH = 800
HEIGHT = 500
FPS = 12 # controls how often the gameDisplay should refresh. In our case, it will refresh every 1/12th second
pygame.init()
pygame.display.set_caption('水果忍者 -- Python代码大全')
gameDisplay = pygame.display.set_mode((WIDTH, HEIGHT)) # setting game display size
clock = pygame.time.Clock()
# Define colors
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
background = pygame.image.load('back.jpg') # game background
font = pygame.font.Font(os.path.join(os.getcwd(), 'comic.ttf'), 42)
score_text = font.render('Score : ' + str(score), True, (255, 255, 255)) # score display
lives_icon = pygame.image.load('images/white_lives.png') # images that shows remaining lives
cap = cv2.VideoCapture(0)
# Generalized structure of the fruit Dictionary
def generate_random_fruits(fruit):
fruit_path = "images/" + fruit + ".png"
data[fruit] = {
'img': pygame.image.load(fruit_path),
'x': random.randint(100, 500), # where the fruit should be positioned on x-coordinate
'y': 800,
'speed_x': random.randint(-10, 10),
# how fast the fruit should move in x direction. Controls the diagonal movement of fruits
'speed_y': random.randint(-80, -60), # control the speed of fruits in y-directionn ( UP )
'throw': False,
# determines if the generated coordinate of the fruits is outside the gameDisplay or not. If outside, then it will be discarded
't': 0, # manages the
'hit': False,
}
if random.random() >= 0.75: # Return the next random floating point number in the range [0.0, 1.0) to keep the fruits inside the gameDisplay
data[fruit]['throw'] = True
else:
data[fruit]['throw'] = False
# Dictionary to hold the data the random fruit generation
data = {}
for fruit in fruits:
generate_random_fruits(fruit)
def hide_cross_lives(x, y):
gameDisplay.blit(pygame.image.load("images/red_lives.png"), (x, y))
# Generic method to draw fonts on the screen
font_name = pygame.font.match_font('comic.ttf')
def draw_text(display, text, size, x, y):
font = pygame.font.Font(font_name, size)
text_surface = font.render(text, True, WHITE)
text_rect = text_surface.get_rect()
text_rect.midtop = (x, y)
gameDisplay.blit(text_surface, text_rect)
# draw players lives
def draw_lives(display, x, y, lives, image):
for i in range(lives):
img = pygame.image.load(image)
img_rect = img.get_rect() # gets the (x,y) coordinates of the cross icons (lives on the the top rightmost side)
img_rect.x = int(x + 35 * i) # sets the next cross icon 35pixels awt from the previous one
img_rect.y = y # takes care of how many pixels the cross icon should be positioned from top of the screen
display.blit(img, img_rect)
# show game over display & front display
def show_gameover_screen():
gameDisplay.blit(background, (0, 0))
draw_text(gameDisplay, "FRUIT NINJA!", 90, WIDTH / 2, HEIGHT / 4)
if not game_over:
draw_text(gameDisplay, "Score : " + str(score), 50, WIDTH / 2, HEIGHT / 2)
draw_text(gameDisplay, "Press a key to begin!", 64, WIDTH / 2, HEIGHT * 3 / 4)
pygame.display.flip()
waiting = True
while waiting:
clock.tick(FPS)
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
if event.type == pygame.KEYUP:
waiting = False
# Game Loop
first_round = True
game_over = True # terminates the game While loop if more than 3-Bombs are cut
game_running = True # used to manage the game loop
while game_running:
ret, frame = cap.read()
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 因为摄像头是镜像的,所以将摄像头水平翻转
# 不是镜像的可以不翻转
frame = cv2.flip(frame, 1)
results = hands.process(frame)
frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR)
# print("""dddddddd111111""")
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
# 识别状态
hand_dict = {i: v for i, v in enumerate(hand_landmarks.landmark)}
# finger_table = shibie_wuzhi_zhuangtai(hand_dict)
# history_list.append(hand_dict)
if hand_dict:
currentMouseX = int(hand_dict[8].x * screenWidth)
currentMouseY = int(hand_dict[8].y * screenHeight)
pyautogui.moveTo(currentMouseX, currentMouseY, duration=0, tween=pyautogui.linear)
if game_over:
if first_round:
show_gameover_screen()
first_round = False
game_over = False
player_lives = 3
draw_lives(gameDisplay, 690, 5, player_lives, 'images/red_lives.png')
score = 0
for event in pygame.event.get():
# checking for closing window
if event.type == pygame.QUIT:
game_running = False
gameDisplay.blit(background, (0, 0))
gameDisplay.blit(score_text, (0, 0))
draw_lives(gameDisplay, 690, 5, player_lives, 'images/red_lives.png')
for key, value in data.items():
if value['throw']:
value['x'] += value['speed_x'] # moving the fruits in x-coordinates
value['y'] += value['speed_y'] # moving the fruits in y-coordinate
value['speed_y'] += (1 * value['t']) # increasing y-corrdinate
value['t'] += 1 # increasing speed_y for next loop
if value['y'] <= 800:
gameDisplay.blit(value['img'],
(value['x'], value['y'])) # displaying the fruit inside screen dynamically
else:
generate_random_fruits(key)
current_position = pygame.mouse.get_pos() # gets the current coordinate (x, y) in pixels of the mouse
print(current_position)
if not value['hit'] and current_position[0] > value['x'] and current_position[0] < value['x'] + 60 \
and current_position[1] > value['y'] and current_position[1] < value['y'] + 60:
if key == 'bomb':
player_lives -= 1
if player_lives == 0:
hide_cross_lives(690, 15)
elif player_lives == 1:
hide_cross_lives(725, 15)
elif player_lives == 2:
hide_cross_lives(760, 15)
# if the user clicks bombs for three time, GAME OVER message should be displayed and the window should be reset
if player_lives < 0:
show_gameover_screen()
game_over = True
half_fruit_path = "images/explosion.png"
else:
half_fruit_path = "images/" + "half_" + key + ".png"
value['img'] = pygame.image.load(half_fruit_path)
value['speed_x'] += 10
if key != 'bomb':
score += 1
score_text = font.render('Score : ' + str(score), True, (255, 255, 255))
value['hit'] = True
else:
generate_random_fruits(key)
pygame.display.update()
clock.tick(
FPS) # keep loop running at the right speed (manages the frame/second. The loop should update afer every 1/12th pf the sec
pygame.quit()
我的第一款隔空操作游戏算是手残了
原创
©著作权归作者所有:来自51CTO博客作者东方佑的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:4k动漫壁纸28
下一篇:隔空操作之简单的模拟三种行为
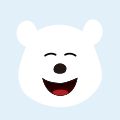
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
我做的第一款鸿蒙demo!
之前看到“粒子消散”的特效组件,于是就产生想法!
ide 斜率 全局变量 播放音频 随机变化 -
有三AI终于摊牌了,第一款产品上市!
当扑克遇到了AI
深度学习 开源框架 图像分类 百度 tensorflow -
Spring Boot实战:我们的第一款开源软件
让收藏更简单,让收藏更智慧!
Spring Boot -
华为第一款台式机正式上线!
此前,有关华为高性能智慧电脑台式机的爆料消息屡屡传出,网上也有消息称华为有可能会采用鲲鹏主板以及华为黄...
物联网 openstack vm windows junit