Java IO实战操作(三)
转载
/**
* IO管道处理集合
*/
public void OutFile() throws FileNotFoundException {
/**
* 使用PrintStream进行输出
*/
PrintStream print = new PrintStream(new FileOutputStream(new File("d:"
+ File.separator + "hello.txt")));
print.println(true);
print.println("Rollen");
print.close();
/** *****格式化输出******** */
String name = "Rollen";
int age = 20;
print.printf("姓名:%s. 年龄:%d.", name, age);
print.close();
/** * 使用OutputStream向屏幕上输出内容 * */
OutputStream out = System.out;
try {
out.write("hello".getBytes());
} catch (Exception e) {
e.printStackTrace();
}
try {
out.close();
} catch (Exception e) {
e.printStackTrace();
}
/** * 为System.out.println()重定向输出 * */
// 此刻直接输出到屏幕
System.out.println("hello");
File file = new File("d:" + File.separator + "hello.txt");
try {
System.setOut(new PrintStream(new FileOutputStream(file)));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
System.out.println("这些内容在文件中才能看到哦!");
/** * System.err重定向 这个例子也提示我们可以使用这种方法保存错误信息 * */
System.err.println("这些在控制台输出");
try {
System.setErr(new PrintStream(new FileOutputStream(file)));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
System.err.println("这些在文件中才能看到哦!");
/** * System.in重定向 前提是文件中提前要有值* */
if (!file.exists()) {
return;
} else {
try {
System.setIn(new FileInputStream(file));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
byte[] bytes = new byte[1024];
int len = 0;
try {
len = System.in.read(bytes);
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("读入的内容为:" + new String(bytes, 0, len));
}
/** * 使用缓冲区从键盘上读入内容 * */
BufferedReader buf = new BufferedReader(
new InputStreamReader(System.in));
String str = null;
System.out.println("请输入内容");
try {
str = buf.readLine();
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("你输入的内容是:" + str);
}
/**
* 消息发送类
*/
class Send implements Runnable {
private PipedOutputStream out = null;
public Send() {
out = new PipedOutputStream();
}
public PipedOutputStream getOut() {
return this.out;
}
public void run() {
String message = "hello , Rollen";
try {
out.write(message.getBytes());
} catch (Exception e) {
e.printStackTrace();
}
try {
out.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
/** * 接受消息类 * */
class Recive implements Runnable {
private PipedInputStream input = null;
public Recive() {
this.input = new PipedInputStream();
}
public PipedInputStream getInput() {
return this.input;
}
public void run() {
byte[] b = new byte[1000];
int len = 0;
try {
len = this.input.read(b);
} catch (Exception e) {
e.printStackTrace();
}
try {
input.close();
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("接受的内容为 " + (new String(b, 0, len)));
}
/** Scanner读取数据 */
public void OutFile() {
File file = new File("d:" + File.separator + "hello.txt");
Scanner sca = null;
try {
sca = new Scanner(file);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
String str = sca.next();
System.out.println("从文件中读取的内容是:" + str);
}
/**
* 数据操作流DataOutputStream、DataInputStream类
* @throws IOException
*/
public void DataOutFile() throws IOException {
File file = new File("d:" + File.separator + "hello.txt");
char[] ch = { 'A', 'B', 'C' };
DataOutputStream out = null;
out = new DataOutputStream(new FileOutputStream(file));
for (char temp : ch) {
out.writeChar(temp);
}
out.close();
}
/**
* DataInputStream读取数据
* @throws IOException
*/
public void DataInputStreamFile() throws IOException {
File file = new File("d:" + File.separator + "hello.txt");
DataInputStream input = new DataInputStream(new FileInputStream(file));
char[] ch = new char[10];
int count = 0;
char temp;
while ((temp = input.readChar()) != 'C') {
ch[count++] = temp;
}
System.out.println(ch);
}
/**
* 合并流 SequenceInputStream合并文件 合并hello1/hello2到hello中
* @throws IOException
*/
public void SequenceInputStreamFile() throws IOException {
File file1 = new File("d:" + File.separator + "hello1.txt");
File file2 = new File("d:" + File.separator + "hello2.txt");
File file3 = new File("d:" + File.separator + "hello.txt");
InputStream input1 = new FileInputStream(file1);
InputStream input2 = new FileInputStream(file2);
OutputStream output = new FileOutputStream(file3);
// 合并流
SequenceInputStream sis = new SequenceInputStream(input1, input2);
int temp = 0;
while ((temp = sis.read()) != -1) {
output.write(temp);
}
input1.close();
input2.close();
output.close();
sis.close();
}
本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:matlab绘制树
下一篇:Java IO实战操作(四)
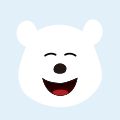
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java IO - 源码: InputStream
本文主要从JDK 11 源码角度分析InputStream。
Java IO/NIO/AIO -
Java NIO - IO多路复用详解
本文主要对IO多路复用,Ractor模型以及Java NIO对其的支持。
Java IO/NIO/AIO -
java中的IO操作总结(三)
说实话,其实我并不是很喜欢Java这门语言,尽管它很强大,有很
java io 输入输出 java System API