#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_N 26
#define GETCODE(a) (a - 'a')
typedef struct Node {
char *flag;
struct Node *next[MAX_N], *tail;
} Node;
typedef struct Queue {
Node **d;
int head, file;
} Queue;
int size_cnt;
Queue *initQueue(int x) {
Queue *p = (Queue *)malloc(sizeof(Queue));
p->d = (Node **)malloc(sizeof(Node *) * x);
p->head = p->file = 0;
return p;
}
int empty(Queue *q) {
return q->file ^ q->head;
}
Node* font(Queue *q) {
if (empty(q)) return q->d[q->head];
return 0;
}
void push(Queue *q, Node *root) {
if (!q) return ;
q->d[q->file++] = root;
return ;
}
void initBudeTail(Node *root, Queue *q) {
for (int i = 0; i < MAX_N; i++) root->next[i] && root->next[i] != root && (root->next[i]->tail = root, push(q, root->next[i]), 1) || (root->next[i] = root);
return ;
}
void pop(Queue *q) {
q->head++;
return ;
}
void clearQueue(Queue *q) {
free(q->d);
free(q);
return ;
}
Node *getNewNode() {
++size_cnt;
Node *p = (Node *)malloc(sizeof(Node));
p->tail = 0;
p->flag = NULL;
memset(p->next, 0, sizeof(Node *) * MAX_N);
return p;
}
void __clear(Queue *t) {
Node *p;
while (empty(t)) {
p = font(t);
p->flag && (free(p->flag), 0);
free(p), pop(t);
}
return ;
}
void clear_node(Queue *q) {
if (!empty(q)) return ;
Node *p;
Queue *t = initQueue(size_cnt);
while (empty(q)) {
p = font(q);
for (int i = 0; i < MAX_N; i++) p->next[i] != p->tail->next[i] && (push(q, p->next[i]), 0);
pop(q), push(t, p);
}
__clear(t);
clearQueue(t);
return ;
}
void clear(Node *root) {
if (!root) return ;
Queue *q = initQueue(size_cnt);
initBudeTail(root, q);
clear_node(q);
clearQueue(q);
free(root);
return ;
}
void insert(Node *root, const char *s) {
if (!root) return ;
for (int i = 0; s[i]; i++) {
root->next[GETCODE(s[i])] || (root->next[GETCODE(s[i])] = getNewNode());
root = root->next[GETCODE(s[i])];
}
root->flag = strdup(s);
return ;
}
void buildTail(Node *root) {
Queue *q = initQueue(size_cnt);
initBudeTail(root, q);
while (empty(q)) {
Node *p = font(q), *t;
for (int i = 0; i < MAX_N; i++) {
if (!p->next[i]) {
p->next[i] = p->tail->next[i];
continue;
}
p->next[i]->tail = p->tail->next[i];
push(q, p->next[i]);
}
pop(q);
}
clearQueue(q);
return ;
}
int match_ac(Node *root, const char *s) {
int len = 0;
Node * p = root, *q;
for (int i = 0; s[i]; i++) {
p = p->next[GETCODE(s[i])];
q = p;
while (q != root) {
q->flag && printf("OK: %s\n", q->flag) && ++len;
q = q->tail;
}
}
return len;
}
int main() {
int m, n;
scanf("%d", &m);
char s[100] = {0};
Node *root = getNewNode();
for (int i = 0; i < m; i++) {
scanf("%s", s);
insert(root, s);
}
buildTail(root);
scanf("%s", s);
printf("find %s == %d\n", s, match_ac(root, s));
clear(root);
return 0;
}
AC自动机, 多模匹配, 字符串匹配
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
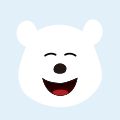
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】详细介绍串的简单模式匹配——朴素模式匹配算法
【数据结构】第四章——串详细介绍串的朴素模式匹配算法……
数据结构 模式匹配 C语言 字符串匹配 -
C++ 模板匹配matchTemplate
C++ 模板匹配matchTemplate
opencv 模版匹配 -
python凸包检测怎么抑制凸包数量
一.概念: 凸包(Convex Hull)是一个计算几何(图形学)中的概念。 在一个实数向量空间V中,对于给定集合X,所有包含X的凸集的交集S被称为X的凸包。 X的凸包可以用X内所有点(X1,...Xn)的线性组合来构造.
python凸包检测怎么抑制凸包数量 #include 极角排序 i++