import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage>
with SingleTickerProviderStateMixin {
AnimationController controller;
@override
void initState() {
super.initState();
controller = AnimationController(
duration: Duration(seconds: 1),
vsync: this,
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Padding(
padding: const EdgeInsets.all(8.0),
child: AnimatedTextField(
controller: controller,
),
),
),
floatingActionButton: FloatingActionButton(
child: Icon(Icons.opacity),
onPressed: () {
var status = controller.status;
if (status == AnimationStatus.completed) {
controller.reverse();
} else if (status == AnimationStatus.dismissed) {
controller.forward();
}
},
),
);
}
}
class AnimatedTextField extends StatefulWidget {
final AnimationController controller;
final Animation<double> opacity;
final Animation<double> left;
AnimatedTextField({
Key key,
@required this.controller,
}) : opacity = Tween<double>(
begin: 1.0,
end: 0.0,
).animate(
CurvedAnimation(
parent: controller,
curve: Interval(
0.0,
0.5,
curve: Curves.easeInOut,
),
),
),
left = Tween<double>(
begin: 20.0,
end: 0.0,
).animate(
CurvedAnimation(
parent: controller,
curve: Interval(
0.0,
1.0,
curve: Curves.easeIn,
),
),
),
super(key: key);
@override
_AnimatedTextFieldState createState() => _AnimatedTextFieldState();
}
class _AnimatedTextFieldState extends State<AnimatedTextField> {
TextEditingController _textEditingController =
TextEditingController(text: 'hello fluttr');
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: widget.controller,
builder: (context, child) {
var theme = Theme.of(context);
var o = widget.opacity.value;
var _child = o > 0.0
? TextField(
controller: _textEditingController,
style: theme.textTheme.body2,
decoration: InputDecoration(
focusedBorder: UnderlineInputBorder(
borderSide: BorderSide(color: Color.fromRGBO(0, 0, 0, 1)),
),
enabledBorder: UnderlineInputBorder(
borderSide: BorderSide(color: Color.fromRGBO(0, 0, 0, o)),
),
),
)
: Opacity(
opacity: (o - 1).abs(),
child: Align(
alignment: Alignment.centerLeft,
child: Text(
_textEditingController.text,
style: theme.textTheme.body2,
),
),
);
return Padding(
padding: EdgeInsets.only(
left: widget.left.value,
top: 20,
right: 20,
bottom: 20,
),
child: _child,
);
},
);
}
}
Flutter 将TextField平滑过渡到Text
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:perl 打印简单的help文档
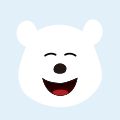
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
mysql向mariadb平滑过渡
简单介绍了mariadb
mysql 存储引擎 oracle ide centos -
从EIGRP到OSPF的平滑过渡
EIGRP到OSPF的过渡。
职场 OSPF EIGRP 休闲 -
集成 Sermant,ServiceStage 带你实现应用上下线平滑过渡
为了保证应用正确上下线、流量不丢失,ServiceStage 基于 Sermant 提供了一套优雅上下线的方案,包括预热、延迟下线等,避免了请求超时、连接拒绝、流量丢失等问题的发生。
微服务 人工智能 字节码 ServiceStage Sermant -
JavaScript实现缩略图到全宽图像页的平滑过渡
要实现缩略图到全宽图像页的平滑过渡效果,您可以使用JavaScript和CSS过渡效果来实现。下面是一个简单的示例:HTML:<div clas
javascript 开发语言 ecmascript 前端 过渡效果 -
flutter TextField类
1、类属性意思 const TextField({ Key key, this.controller, //编辑框的控制器,跟文本框
flutter TextField 输入框 android 获取焦点