static std::string w2c(std::wstring str)
{
int nlength = str.length();
int nbytes = WideCharToMultiByte(0,0,str.c_str(),nlength,NULL,0,NULL,NULL);
if(nbytes == 0) return "";
char*buff = new char[nbytes+1];
WideCharToMultiByte(0,0,str.c_str(),nlength,buff,nbytes,NULL,NULL);
buff[nbytes] = '\0';
std::string ret_str = std::string(buff);
delete [] buff;
return ret_str;
}
static std::wstring c2w(std::string str)
{
if(str.length() == 0) return std::wstring();
int nu = str.length();
size_t n =(size_t)MultiByteToWideChar(CP_ACP,0,str.c_str(),nu,NULL,0);
wchar_t*wbuff = new wchar_t[n+1];
MultiByteToWideChar(CP_ACP,0,str.c_str(),(int)nu,wbuff,(int)n);
wbuff[n] = 0;
std::wstring wstr_ret = std::wstring(wbuff);
delete []wbuff;
return wstr_ret;
}
win32 wstring <-> string
转载文章标签 ide javascript 文章分类 代码人生
本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
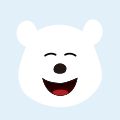
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
STM32的OLED
OLED显示,便于后期调试代码
IIC OLED