#define _CRT_SECURE_NO_WARNINGS
#include<bits/stdc++.h>
#define ull unsigned long long
using namespace std;
const int INF = 0x3f3f3f3f,M=27*27*27-1;
int count(int x, bool st) {
int a[9];
for (int i = 0; i < 9; i++) {
a[i] = x % 3;
x /= 3;
}
int res = 0;
int des;
if (st)des = 1;
else des = 2;
if (a[0] == a[1] && a[1] == a[2] && a[0] == des)res++;
if (a[3] == a[4] && a[4] == a[5] && a[3] == des)res++;
if (a[6] == a[7] && a[7] == a[8] && a[6] == des)res++;
if (a[0] == a[3] && a[3] == a[6] && a[0] == des)res++;
if (a[1] == a[4] && a[4] == a[7] && a[1] == des)res++;
if (a[2] == a[5] && a[5] == a[8] && a[2] == des)res++;
if (a[0] == a[4] && a[4] == a[8] && a[0] == des)res++;
if (a[2] == a[4] && a[4] == a[6] && a[2] == des)res++;
return res;
}
int g_n_0(int x) {
for (int i = 0; i < 9; i++) {
if (x % 3 == 0)return i;
x /= 3;
}
return -1;
}
int dp[20010][3];
int tot0[20000];
int pow3[20];
int t, n, m;
int main() {
memset(tot0, 0, sizeof tot0);
for (int i = 1; i <= 20000; i++)
dp[i][1] = -INF, dp[i][0] = INF;
pow3[0] = 1;
for (int i = 1; i <= 9; i++)pow3[i] = 3 * pow3[i - 1];
for (int i = 1; i <= M; i++)
if (g_n_0(i) == -1)dp[i][0] = dp[i][1] = count(i, 1) - count(i, 0);
int a = 1;
for (int i = 0; i <= M; i++) {
int x = i;
for (int j = 0; j < 9; j++) {
if (x % 3 == 0)tot0[i]++;
x /= 3;
}
}
for (int tot = 1; tot <= 9; tot++)
for (int i = 0; i <= M; i++) {
if (tot0[i] != tot)continue;
for (int j = 0; j <= 1; j++) {
int x = i;
if (j) {
for (int z = 0; z < 9; z++) {
if (x % 3 == 0)dp[i][j] = max(dp[i][j], dp[i + pow3[z]][0]);
x /= 3;
}
}
else {
for (int z = 0; z < 9; z++) {
if (x % 3 == 0)dp[i][j] = min(dp[i][j], dp[i + 2 * pow3[z]][1]);
x /= 3;
}
}
}
}
int t;
cin >> t;
while (t--) {
bool st;
cin >> st;
string s;
int str=0;
for (int i = 0; i < 3; i++) {
cin >> s;
for (int j = 0; j < 3; j++) {
if (s[j] == '.')str = 3 * str;
if (s[j] == 'O')str = 3 * str + 1;
if (s[j] == 'X')str = 3 * str + 2;
}
}
cout << dp[str][st] << endl;
}
}
三进制状压dp
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
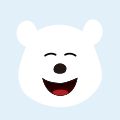
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Movie[状压DP]
题目描述:小石头喜欢看电影,选择有 N 部电影可供选择,每一部电影会在一天的不同时段播放。他希望
状压dp 数据 i++ #define -
HDU 3001 Travelling (状压DP,3进制)
题意: 给出n 2 #include 3 #include 4 #include 5 #includ
状压DP #include i++ #define 进制