jQuery 是一个 JavaScript 库
4.0jquey基础
转载在<head></head>标签中引入jquery库
<script type="text/javascript" src="js/jquery-1.11.3.min.js"></script>
所有的jquery代码都在
<script type="text/javascript"> 对于html5可以省略type="text/javascript"
$(document).ready(function(){
});
</script> 中实现
//例如点击所有p标签元素后隐藏 $("p")代表所有的p元素
<script type="text/javascript">
$(document).ready(function(){
$("p").click(function(){
$(this).hide();
});
});
</script>
//jquery设置、获取值 $("#start_Time")代表id=start_Time的元素
$("#start_Time").val(ToData());
var start_Time = $("#start_Time").val();
var div=$("div");
//通过id获取表单
$('#searchCondition')[0];
//重载页面 刷新
window.location.reload();
window.parent.location.reload();
//设置css样式
$("#lui_grid_arrival").css("display","none");
//点击按钮实现样式转换
$("button").click(function(){
$("p").css("background-color","red");
});
//点击按钮返回元素样式
$("button").click(function(){
alert("Background-color="+$("p").css("background-color"));
});
//常用
$("p").css("background-color","yellow");
$("p").css({"background-color":"yellow","font-size":"200%"});
//设置元素 属性
alert($("#w3s").attr("href"));
$("button").click(function(){//button事件 测试
$("#w3s").attr({
});
});
//添加/删除一个或多个类
$("h1,h2,p").addClass("blue");
$("div").addClass("important");
$("#div1").addClass("important blue");
$("h1,h2,p").removeClass("blue");
$("h1,h2,p").toggleClass("blue");
创建元素
var txt3=document.createElement("big"); // 通过 DOM 创建元素
txt3.innerHTML="jQuery!";
删除元素
$("button").click(function(){
$("#div1").remove();
});
$("#div1").empty();//清空元素内容
$("p").remove(".italic");//删除class="italic"的内容
调整div尺寸
$("#div1").width(320).height(320);
<div id="div1" style="height:100px;width:100px;padding:10px;margin:3px;border:1px solid blue;background-color:lightblue;"></div>
//动作完成后的回调函数(隐藏完成后弹出提示)
$("button").click(function(){//放在点击事件中 测试
$("p").hide(1000,function(){
alert("The paragraph is now hidden");
});
});
//多方法同时操作元素
$("button").click(function(){//放在点击事件中 测试
//$("#p1").css("color","red").slideUp(2000).slideDown(2000);
$("#p1").css("color","red")
.slideUp(3000)
.slideDown(3000);
});
//text()/html()/val()方法的区别
<p id="test">这是段落中的<b>粗体</b>文本。</p>
<p>姓名:<input type="text" id="test" value="米老鼠"></p>
alert("Text: " + $("#test").text());
alert("HTML: " + $("#test").html());
alert("Value: " + $("#test").val();
<p id="test1">这是段落。</p>
<p id="test2">这是另一个段落。</p>
<p>Input field: <input type="text" id="test3" value="Mickey Mouse"></p>
<button id="btn1">设置文本</button>
<button id="btn2">设置 HTML</button>
<button id="btn3">设置值</button>
$("#btn1").click(function(){
$("#test1").text("Hello world!");
});
$("#btn2").click(function(){
$("#test2").html("<b>Hello world!</b>");
});
$("#btn3").click(function(){
$("#test3").val("Dolly Duck");
});
//元素的宽高和边距
<div id="div1" style="height:100px;width:300px;padding:10px;margin:3px;border:1px solid blue;background-color:lightblue;"></div>
<button>显示 div 的尺寸</button>
$("button").click(function(){
var txt="";
//width() 方法设置或返回元素的宽度(不包括内边距、边框或外边距)
//height() 方法设置或返回元素的高度(不包括内边距、边框或外边距)
txt+="Width of div: " + $("#div1").width() + "</br>";
txt+="Height of div: " + $("#div1").height();
//innerWidth() 方法返回元素的宽度(包括内边距)
//innerHeight() 方法返回元素的高度(包括内边距)
txt+="Inner width of div: " + $("#div1").innerWidth() + "</br>";
txt+="Inner height of div: " + $("#div1").innerHeight();
//outerWidth() 方法返回元素的宽度(包括内边距和边框)
//outerHeight() 方法返回元素的高度(包括内边距和边框)
txt+="Outer width of div: " + $("#div1").outerWidth() + "</br>";
txt+="Outer height of div: " + $("#div1").outerHeight();
//outerWidth(true) 方法返回元素的宽度(包括内边距、边框和外边距)
//outerHeight(true) 方法返回元素的高度(包括内边距、边框和外边距)
txt+="Outer width of div (margin included): " + $("#div1").outerWidth(true) + "</br>";
txt+="Outer height of div (margin included): " + $("#div1").outerHeight(true);
$("#div1").html(txt);
});
//显示文档和窗口的尺寸
<script type="text/javascript">
$(document).ready(function(){
$("button").click(function(){
var txt="";
txt+="Document width/height: " + $(document).width();
txt+="x" + $(document).height() + "\n";
txt+="Window width/height: " + $(window).width();
txt+="x" + $(window).height();
alert(txt);
});
});
</script>
//父亲 /儿子
<div><p>Hello</p></div>
<div class="selected"><p>Hello Again</p></div>
<script>
$("p").parent(".selected").css("background", "yellow");
</script>
//最近父元素
$('li.item-a').parent().css('background-color', 'red');
//所有上级父元素
$('li.item-a').parents().css('background-color', 'red');
<div>
<span>Hello</span>
<p class="selected">Hello Again</p>
<div class="selected">And Again</div>
<p>And One Last Time</p>
</div>
<script>$("div").children(".selected").css("color", "blue");</script>
//遍历元素
$("button").click(function(){
$("li").each(function(){
alert($(this).text());
});
});
<button>输出每个列表项的值</button>
<ul>
<li>Coffee</li>
<li>Milk</li>
<li>Soda</li>
</ul>
//过滤器
$("div").css("background", "#c8ebcc")
.filter(".middle")
.css("border-color", "red");
<div></div>
<div class="middle"></div>
<div class="middle"></div>
$("p").find("span").css('color','red');
<p><span>Hello</span>, how are you?</p>
<p>Me? I'm <span>good</span>.</p>
var $allListElements = $('li');
$('li.item-ii').find( $allListElements ).css('background-color', 'red');
//捕捉元素 当点击到li时设置样式
<ul>
<li>list <strong>item 1</strong></li>
<li><span>list item 2</span></li>
<li>list item 3</li>
</ul>
<script>
$("ul").click(function(event) {
var $target = $(event.target);
if ( $target.is("li") ) {
$target.css("background-color", "red");
}
});
</script>
//常用方法 筛选
//find() 在div的子元素中找到所有p元素 包括div元素本身
$("div").find("p").andSelf().addClass("border");
$("div").find("p").addClass("background");
first()方法 第一个元素
<p><span>Look:</span> <span>This is some text in a paragraph.</span> <span>This is a note about it.</span></p>
<script>$("p span").first().addClass('highlight');</script>
$('li').first().css('background-color', 'red');
<ul>
<li>list item 1</li>
<li>list item 2</li>
<li>list item 3</li>
</ul>
last()方法
<p><span>Look:</span> <span>This is some text in a paragraph.</span> <span>This is a note about it.</span></p>
<script>$("p span").last().addClass('highlight');</script>
$('li').last().css('background-color', 'red');
//$("p").next(".selected").css("background", "yellow");
$("p.selected").css("background", "yellow");
//prev() next()
<ul>
<li>list item 1</li>
<li>list item 2</li>
<li class="third-item">list item 3</li>
<li>list item 4</li>
<li>list item 5</li>
</ul>
<script>
$('li.third-item').prev().css('background-color', 'red');
$('li.third-item').next().css('background-color', 'red');
</script>
//还可以筛选带样式的元素
<div><span>Hello</span></div>
<p class="selected">Hello Again</p>
<p>And Again</p>
<script>
$("p").prev(".selected").css("background", "yellow");
</script>
//nextAll() class="third-item"的所有并列后续元素 包括$("li.third-item")本身
$("li.third-item").nextAll().andSelf().css("background-color", "red");
//prevAll()
<div></div>
<div></div>
<div></div>
<div></div>
<script>$("div:last").prevAll().addClass("before");</script>
end() 结束最近的选中 $("p").find("span").end()代表span的选中回到p
<p><span>Hello</span>, how are you?</p>
<script>$("p").find("span").end().css("border", "2px red solid");</script>
<body>
<ul class="first">
<li class="foo">list item 1</li>
<li>list item 2</li>
<li class="bar">list item 3</li>
</ul>
<ul class="second">
<li class="foo">list item 1</li>
<li>list item 2</li>
<li class="bar">list item 3</li>
</ul>
<script>$('ul.first').find('.foo').css('background-color', 'red')
.end().find('.bar').css('background-color', 'green');</script>
</body>
eq() 方法 按索引查,一般是并列元素
<script>$("body").find("div").eq(2).addClass("blue");</script>
<script>$('li').eq(2).css('background-color', 'red');</script>
<script>$('li').eq(-2).css('background-color', 'red');</script>
<script>$('li').eq(5).css('background-color', 'red');</script>
本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:4.2 jquery事件
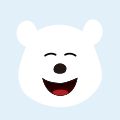
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
jQuey基础思维导图梳理1
jQuey基础思维导图梳理
jQuery -
RedHatAS4.0-网络基础
RedHatAS4.0-网络基础
linux RedHat 网络基础 系统 RedHatAS4.0教程 -
jquey写的简单图片轮转
通俗易懂的jquery幻灯片轮转代码
HTML css jquery 幻灯片轮转 大气 -
jquey datatables checkbox 列排序
jquey datatable checkbox 列排序,icheck的选择框排序如果你的checkbox是icheck的,请参考:
javascript 前端 开发语言 初始化 换页 -
Jquey拖拽控件Draggable用法
本例主要目的是使用Jquey的Draggable控件........
asp.net js html jquery javascript 拖拽 -
Solr 4.0 基础教程
本文只是Solr 4.0的基础教程,本人不经常写东西,写的不好请见谅,欢迎到群233413850进行讨论学习。 转载请标明原文地址:http://my.oschina.net/zhanyu/blo...
solr xml tomcat apache lucene -
android 渲染html链接
本篇文章意在讲解Flutter测量、布局和渲染和android的区别,了解了布局的渲染自己在flutter的自定义控件中才能如鱼得水,首先先从整个渲染的入口Mian.dart的main方法的runApp方法void runApp(Widget app) { WidgetsFlutterBinding.ensureInitialized() ..scheduleAttachRootWid
android 渲染html链接 flutter Flutter渲染流程 Flutter源码讲解 Flutter测量布局详解