#!/bin/bash function count() { local insert=0 local delete=0 while read line ;do current=`echo $line| awk -F',' '{printf $2}' | awk '{printf $1}'` if [[ -n $current ]]; then insert=`expr $insert + $current` fi current=`echo $line | sed -n 's/.*, //p' | awk '{printf $1}'` if [[ -n $current ]]; then delete=`expr $delete + $current` fi done < .tmp.count echo "$insert insertions, $delete deletions" } function countAll() { git log --author=wxy --shortstat --pretty=format:"" | sed /^$/d >.tmp.count count; rm .tmp.count } function countToday() { local current=`date +%s`; local begin=`date +%Y-%m-%d |xargs date +%s -d`; local minutes=$(($current - $begin)); git log --author=wxy --since="$minutes seconds ago" --shortstat --pretty=format:"" | sed /^$/d >.tmp.count count; rm .tmp.count } function countOneDay() { git log --author=wxy --since="1 days ago" --shortstat --pretty=format:"" | sed /^$/d >.tmp.count count; rm .tmp.count } if [[ ! -n $1 ]] || [[ $1 = "all" ]] ; then countAll; elif [[ $1 = "oneday" ]]; then countOneDay; elif [[ $1 = "today" ]]; then countToday; else echo "args: all | oneday | today"; fi git log --graph --date=yyyymmdd --pretty=format:'%ae%cd%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr)%Creset' --abbrev-commit --date=relative git log --graph --date=yyyymmdd --pretty=format:'%ae%cd%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr)%Creset' --abbrev-commit --date=relative git log --pretty=format:'%ci %an %s' > work.txt
git工作量统计
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
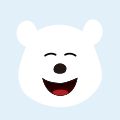
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
mysql 查询数据库响应时长
mysql 查询数据库响应时长的几种方法介绍。
MySQL sql 执行时间 -
ES复合查询工作量评估
ES复合查询工作量评估
elasticsearch should must not 字段 解决方案 -
测试工作量的评估
尝试着列一下有多少个测试点,这样能够大约摸看看能有多少个测试用例。然后,自己看看大概要规划几轮测试。这样根据你现在的人员经验就大致上知道写
测试工作量的评估 怎么评估测试工作量 软件测试工作量评估 怎么好软件测试工作量评估 测试工作量评估 -
如何估算测试工作量功能点 开发模式 数据