terminatedThreadCount++;
break;
default:
break;
}
} else {
terminatedThreadCount++;
}
}
// 新建线程数
map.put(“jvm.thread.new.count”, newThreadCount);
// 运行线程数
map.put(“jvm.thread.runnable.count”, runnableThreadCount);
// 阻塞线程数
map.put(“jvm.thread.blocked.count”, blockedThreadCount);
// 等待线程数
map.put(“jvm.thread.waiting.count”, waitThreadCount);
// 等待超时线程数
map.put(“jvm.thread.time_waiting.count”, timeWaitThreadCount);
// 死亡线程数
map.put(“jvm.thread.terminated.count”, terminatedThreadCount);
long[] ids = threadBean.findDeadlockedThreads();
map.put(“jvm.thread.deadlock.count”, ids == null ? 0 : ids.length);
获取系统cpu信息
=========
直接使用oshi的系统api
JSONObject cpuInfo = new JSONObject();
CentralProcessor processor = hardware.getProcessor();
// CPU信息
long[] prevTicks = processor.getSystemCpuLoadTicks();
Util.sleep(OSHI_WAIT_SECOND);
long[] ticks = processor.getSystemCpuLoadTicks();
long nice = ticks[CentralProcessor.TickType.NICE.getIndex()] - prevTicks[CentralProcessor.TickType.NICE.getIndex()];
long irq = ticks[CentralProcessor.TickType.IRQ.getIndex()] - prevTicks[CentralProcessor.TickType.IRQ.getIndex()];
long softirq = ticks[CentralProcessor.TickType.SOFTIRQ.getIndex()] - prevTicks[CentralProcessor.TickType.SOFTIRQ.getIndex()];
long steal = ticks[CentralProcessor.TickType.STEAL.getIndex()] - prevTicks[CentralProcessor.TickType.STEAL.getIndex()];
long cSys = ticks[CentralProcessor.TickType.SYSTEM.getIndex()] - prevTicks[CentralProcessor.TickType.SYSTEM.getIndex()];
long user = ticks[CentralProcessor.TickType.USER.getIndex()] - prevTicks[CentralProcessor.TickType.USER.getIndex()];
long iowait = ticks[CentralProcessor.TickType.IOWAIT.getIndex()] - prevTicks[CentralProcessor.TickType.IOWAIT.getIndex()];
long idle = ticks[CentralProcessor.TickType.IDLE.getIndex()] - prevTicks[CentralProcessor.TickType.IDLE.getIndex()];
long totalCpu = user + nice + cSys + idle + iowait + irq + softirq + steal;
//cpu核数
cpuInfo.put(“cpuNum”, processor.getLogicalProcessorCount());
//cpu系统使用率
cpuInfo.put(“cSys”, new DecimalFormat(“#.##%”).format(cSys * 1.0 / totalCpu));
//cpu用户使用率
cpuInfo.put(“user”, new DecimalFormat(“#.##%”).format(user * 1.0 / totalCpu));
//cpu当前等待率
cpuInfo.put(“iowait”, new DecimalFormat(“#.##%”).format(iowait * 1.0 / totalCpu));
//cpu当前使用率
cpuInfo.put(“idle”, new DecimalFormat(“#.##%”).format(1.0 - (idle * 1.0 / totalCpu)));
System.out.println(cpuInfo);
获取系统内存信息(非jvm)
==============
package io.github.quickmsg.metric.category;
import lombok.extern.slf4j.Slf4j;
import oshi.SystemInfo;
import oshi.hardware.*;
import oshi.software.os.FileSystem;
import oshi.software.os.NetworkParams;
import oshi.software.os.OSFileStore;
import oshi.software.os.OperatingSystem;
import oshi.util.FormatUtil;
import java.util.Arrays;
import java.util.List;
/**
• 系统数据
•
• @author luxurong
*/
@Slf4j
public class SysMetric {
public static void main(String[] args) {
// Options: ERROR > WARN > INFO > DEBUG > TRACE
log.info(“Initializing System…”);
SystemInfo si = new SystemInfo();
HardwareAbstractionLayer hal = si.getHardware();
OperatingSystem os = si.getOperatingSystem();
System.out.println(os);
log.info(“Checking computer system…”);
printComputerSystem(hal.getComputerSystem());
log.info(“Checking Processor…”);
printProcessor(hal.getProcessor());
log.info(“Checking Memory…”);
printMemory(hal.getMemory());
log.info(“Checking CPU…”);
printCpu(hal.getProcessor());
log.info(“Checking Sensors…”);
printSensors(hal.getSensors());
log.info(“Checking Power sources…”);
printPowerSources(hal.getPowerSources());
log.info(“Checking Disks…”);
printDisks(hal.getDiskStores());
log.info(“Checking File System…”);
printFileSystem(os.getFileSystem());
log.info(“Checking Network interfaces…”);
printNetworkInterfaces(hal.getNetworkIFs());
log.info(“Checking Network parameterss…”);
printNetworkParameters(os.getNetworkParams());
// hardware: displays
log.info(“Checking Displays…”);
printDisplays(hal.getDisplays());
// hardware: USB devices
log.info(“Checking USB Devices…”);
printUsbDevices(hal.getUsbDevices(true));
}
private static void printComputerSystem(final ComputerSystem computerSystem) {
System.out.println("manufacturer: " + computerSystem.getManufacturer());
System.out.println("model: " + computerSystem.getModel());
System.out.println("serialnumber: " + computerSystem.getSerialNumber());
final Firmware firmware = computerSystem.getFirmware();
System.out.println(“firmware:”);
System.out.println(" manufacturer: " + firmware.getManufacturer());
System.out.println(" name: " + firmware.getName());
System.out.println(" description: " + firmware.getDescription());
System.out.println(" version: " + firmware.getVersion());
final Baseboard baseboard = computerSystem.getBaseboard();
System.out.println(“baseboard:”);
System.out.println(" manufacturer: " + baseboard.getManufacturer());
System.out.println(" model: " + baseboard.getModel());
System.out.println(" version: " + baseboard.getVersion());
System.out.println(" serialnumber: " + baseboard.getSerialNumber());
}
private static void printProcessor(CentralProcessor processor) {
System.out.println(processor);
System.out.println(" " + processor.getPhysicalPackageCount() + " physical CPU package(s)");
System.out.println(" " + processor.getPhysicalProcessorCount() + " physical CPU core(s)");
System.out.println("Identifier: " + processor.getProcessorIdentifier());
System.out.println("ProcessorID: " + processor.getProcessorIdentifier());
}
private static void printMemory(GlobalMemory memory) {
System.out.println("Memory: " + FormatUtil.formatBytes(memory.getAvailable()) + “/”
• FormatUtil.formatBytes(memory.getTotal()));
System.out.println("Swap used: " + FormatUtil.formatBytes(memory.getVirtualMemory().getSwapUsed()) + “/”
• FormatUtil.formatBytes(memory.getVirtualMemory().getSwapTotal()));
}
private static void printCpu(CentralProcessor processor) {
System.out.println(
"Context Switches/Interrupts: " + processor.getContextSwitches() + " / " + processor.getInterrupts());
long[] prevTicks = processor.getSystemCpuLoadTicks();
System.out.println(“CPU, IOWait, and IRQ ticks @ 0 sec:” + Arrays.toString(prevTicks));
// Wait a second…
long[] ticks = processor.getSystemCpuLoadTicks();
System.out.println(“CPU, IOWait, and IRQ ticks @ 1 sec:” + Arrays.toString(ticks));
long user = ticks[CentralProcessor.TickType.USER.getIndex()] - prevTicks[CentralProcessor.TickType.USER.getIndex()];
long nice = ticks[CentralProcessor.TickType.NICE.getIndex()] - prevTicks[CentralProcessor.TickType.NICE.getIndex()];
long sys = ticks[CentralProcessor.TickType.SYSTEM.getIndex()] - prevTicks[CentralProcessor.TickType.SYSTEM.getIndex()];
long idle = ticks[CentralProcessor.TickType.IDLE.getIndex()] - prevTicks[CentralProcessor.TickType.IDLE.getIndex()];
long iowait = ticks[CentralProcessor.TickType.IOWAIT.getIndex()] - prevTicks[CentralProcessor.TickType.IOWAIT.getIndex()];
long irq = ticks[CentralProcessor.TickType.IRQ.getIndex()] - prevTicks[CentralProcessor.TickType.IRQ.getIndex()];
long softirq = ticks[CentralProcessor.TickType.SOFTIRQ.getIndex()] - prevTicks[CentralProcessor.TickType.SOFTIRQ.getIndex()];
long steal = ticks[CentralProcessor.TickType.STEAL.getIndex()] - prevTicks[CentralProcessor.TickType.STEAL.getIndex()];
long totalCpu = user + nice + sys + idle + iowait + irq + softirq + steal;
System.out.format(
“User: %.1f%% Nice: %.1f%% System: %.1f%% Idle: %.1f%% IOwait: %.1f%% IRQ: %.1f%% SoftIRQ: %.1f%% Steal: %.1f%%%n”,
100d * user / totalCpu, 100d * nice / totalCpu, 100d * sys / totalCpu, 100d * idle / totalCpu,
100d * iowait / totalCpu, 100d * irq / totalCpu, 100d * softirq / totalCpu, 100d * steal / totalCpu);
double[] loadAverage = processor.getSystemLoadAverage(3);
System.out.println(“CPU load averages:” + (loadAverage[0] < 0 ? " N/A" : String.format(" %.2f", loadAverage[0]))
• (loadAverage[1] < 0 ? " N/A" : String.format(" %.2f", loadAverage[1]))
• (loadAverage[2] < 0 ? " N/A" : String.format(" %.2f", loadAverage[2])));
}
private static void printSensors(Sensors sensors) {
System.out.println(“Sensors:”);
System.out.format(" CPU Temperature: %.1f°C%n", sensors.getCpuTemperature());
System.out.println(" Fan Speeds: " + Arrays.toString(sensors.getFanSpeeds()));
System.out.format(" CPU Voltage: %.1fV%n", sensors.getCpuVoltage());
}
private static void printPowerSources(List powerSources) {
StringBuilder sb = new StringBuilder("Power: ");
if (powerSources.size() == 0) {
sb.append(“Unknown”);
} else {
double timeRemaining = powerSources.get(0).getTimeRemainingInstant();
if (timeRemaining < -1d) {
sb.append(“Charging”);
} else if (timeRemaining < 0d) {
sb.append(“Calculating time remaining”);
} else {
sb.append(String.format(“%d:%02d remaining”, (int) (timeRemaining / 3600),
(int) (timeRemaining / 60) % 60));
}
}
for (PowerSource pSource : powerSources) {
sb.append(String.format(“%n %s @ %.1f%%”, pSource.getName(), pSource.getTimeRemainingInstant() * 100d));
}
System.out.println(sb.toString());
}
private static void printDisks(List diskStores) {
System.out.println(“Disks:”);
for (HWDiskStore disk : diskStores) {
boolean readwrite = disk.getReads() > 0 || disk.getWrites() > 0;
System.out.format(" %s: (model: %s - S/N: %s) size: %s, reads: %s (%s), writes: %s (%s), xfer: %s ms%n",
disk.getName(), disk.getModel(), disk.getSerial(),
disk.getSize() > 0 ? FormatUtil.formatBytesDecimal(disk.getSize()) : “?”,
java jts Geometry 获取点
转载下一篇:Java C语言属于什么
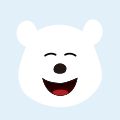
-
java获取当月最大日
java获取当月最大日
System java 字段 -
java递归获取树形结构数据
java递归获取树形结构数据
List 递归 java -
JTS(Geometry)
空间数据模型(1)、JTS Geometry model (2)、ISO Geometry model (Geometry Plugin and JTS Wrapper Plugin)GeoTools has tw
wrapper delegates class null string -
JTS Geometry Operations(一)
Geometry 空间分析方法几何图形操作包在operation包内,包含buffer、distance、linemerge、overlap、polygonize、pre
distance 图形 buffer matrix class -
JTS(Geometry)工具类
空间数据模型(1)、JTS Geometry model(2)、ISO Geometry model (Geometry Plugin and JTS Wrapper Plu
geotools Math System java -
JTS Geometry Operations(二)
一些高级操作, Buffer,LineMerger,Polygonization,UnionLine,凹壳分析,Overlays(1)、Buffer,返回的结果是一个Polygon或者
string buffer class list distance -
JTS Geometry之间的关系
几何信息和拓扑关系是地理信息系统中描述地理要素的空间位置和空间关系的不可缺少的基本信息。其中几何信息主要涉及几
equals string 图形 buffer class -
JTS Geometry关系判断和分析
关系判断Geometry之间的关系有如下几种: 相等(Equals):
jts System List java -
GeoTools应用-JTS(Geometry之间的关系)
几何信息和拓扑关系是地理信息系统中描述地理要素的空间位置和空间关系的不可缺少的基本信息
System 地理信息系统 java -
airsim硬件在环python控制
本章节主要介绍如何搭建涂鸦 Zigbee ZSU 模组 SDK 开发环境。IAR安装前往 IAR 官网下载 IAR Embedded Workbench IDE(IAR for Arm),下载安装完成后打开IAR如图所示:注意:必须使用 ARM 8.40.1或更高版本正版 IAR ,强烈建议使用 8.40.1 版本。Python安装涂鸦 Zigbee SDK 应用工程编译依赖于 Python 脚本
airsim硬件在环python控制 ide vscode visual studio code Python