Android中使用ProgressBar实现进度条
精选
原创
©著作权归作者所有:来自51CTO博客作者mp624183768的原创作品,请联系作者获取转载授权,否则将追究法律责任
Demo展示图片
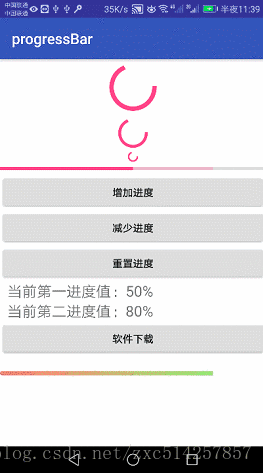
布局代码
//(layout)activity_main.xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="com.test.progressbar.MainActivity">
<ProgressBar
android:id="@+id/progressBarLarge"
style="?android:attr/progressBarStyleLarge"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<ProgressBar
android:id="@+id/progressBarNormal"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<ProgressBar
android:id="@+id/progressBarSmall"
style="?android:attr/progressBarStyleSmall"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<ProgressBar
android:max="100"
android:progress="50"
android:secondaryProgress="80"
android:id="@+id/progressBarHorizontal"
style="?android:attr/progressBarStyleHorizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<!--注:max指最大进度值
progress指第一进度值 如:播放进度
secondProgress指第二进度值 如:缓冲进度 -->
<Button
android:id="@+id/add"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="增加进度"/>
<Button
android:id="@+id/reduce"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="减少进度"/>
<Button
android:id="@+id/reset"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="重置进度"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:text="当前第一进度值:"
android:textSize="20sp"
android:layout_marginLeft="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/firstProgressTv"
android:textSize="20sp"
android:text="50%"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:text="当前第二进度值:"
android:textSize="20sp"
android:layout_marginLeft="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/secondProgressTv"
android:textSize="20sp"
android:text="80%"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="软件下载"
android:onClick="showDialog"/>
<ProgressBar
android:id="@+id/customProgressBar"
style="?android:attr/progressBarStyleHorizontal"
android:progressDrawable="@drawable/progressbar"
android:layout_marginTop="20dp"
android:layout_width="match_parent"
android:layout_height="6dp"/>
<!--通过覆盖原progressBar的progressDrawable实现自定义的目的-->
</LinearLayout>
-------------------------------------------------------------------
//(drawable)progressbar.xml
<layer-list xmlns:android="http://schemas.android.com/apk/res/android" >
<item android:id="@android:id/background">
<shape>
<corners android:radius="5dip" />
<gradient
android:angle="0"
android:centerColor="#fff"
android:centerY="0.75"
android:endColor="#fff"
android:startColor="#fff" />
</shape>
</item>
<item android:id="@android:id/secondaryProgress">
<clip>
<shape>
<corners android:radius="5dip" />
<gradient
android:angle="0"
android:centerColor="#80ffb600"
android:centerY="0.75"
android:endColor="#a0ffcb00"
android:startColor="#80ffd300" />
</shape>
</clip>
</item>
<item android:id="@android:id/progress">
<clip>
<shape>
<corners android:radius="5dip" />
<gradient
android:angle="0"
android:endColor="#8000ff00"
android:startColor="#80ff0000" />
</shape>
</clip>
</item>
</layer-list>
Activity代码
//MainActivity
import android.app.ProgressDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private ProgressBar mProgressBarHorizontal;
private Button mAdd;
private Button mReduce;
private Button mReset;
private TextView mFirstProgressTv;
private TextView mSecondProgressTv;
private int increase = 10;
private int decrease = -10;
private int originalFirstPro = 50;
private int originalSecondPro = 80;
private Context mContext = MainActivity.this;
private ProgressBar mCustomProgressBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
initData();
}
private void initView(){
mProgressBarHorizontal = (ProgressBar) findViewById(R.id.progressBarHorizontal);
mAdd = (Button) findViewById(R.id.add);
mReduce = (Button) findViewById(R.id.reduce);
mReset = (Button) findViewById(R.id.reset);
mFirstProgressTv = (TextView) findViewById(R.id.firstProgressTv);
mSecondProgressTv = (TextView) findViewById(R.id.secondProgressTv);
mCustomProgressBar = (ProgressBar) findViewById(R.id.customProgressBar);
}
private void initData(){
mAdd.setOnClickListener(this);
mReduce.setOnClickListener(this);
mReset.setOnClickListener(this);
// 自定义彩色进度条
mCustomProgressBar.incrementProgressBy(80);
}
@Override
public void onClick(View view) {
int progress;
int secondaryProgress;
int max;
switch (view.getId()){
case R.id.add:
mProgressBarHorizontal.incrementProgressBy(increase);
mProgressBarHorizontal.incrementSecondaryProgressBy(increase);
break;
case R.id.reduce:
mProgressBarHorizontal.incrementProgressBy(decrease);
mProgressBarHorizontal.incrementSecondaryProgressBy(decrease);
break;
case R.id.reset:
mProgressBarHorizontal.setProgress(originalFirstPro);
mProgressBarHorizontal.setSecondaryProgress(originalSecondPro);
break;
}
progress = mProgressBarHorizontal.getProgress();
secondaryProgress = mProgressBarHorizontal.getSecondaryProgress();
max = mProgressBarHorizontal.getMax();
mFirstProgressTv.setText( (int)( progress / (float)max*100) + "%");
mSecondProgressTv.setText( (int)(secondaryProgress / (float)max *100) + "%");
}
public void showDialog(View view){
ProgressDialog dialog = new ProgressDialog(mContext);
// 设置对话框参数
dialog.setIcon(R.mipmap.ic_launcher);
dialog.setTitle("软件下载");
dialog.setMessage("软件下载进度:");
dialog.setCancelable(false);
// 设置进度条参数
dialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
dialog.setMax(100);
dialog.incrementProgressBy(20);
dialog.setIndeterminate(false); // 填false表示是明确显示进度的 填true表示不是明确显示进度的
dialog.setButton(DialogInterface.BUTTON_POSITIVE, "确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(mContext , "确定" , Toast.LENGTH_SHORT).show();
}
});
dialog.setButton(DialogInterface.BUTTON_NEGATIVE, "取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(mContext , "取消" , Toast.LENGTH_SHORT).show();
}
});
dialog.show();
}
}