using System.IO;
using System.Threading.Tasks;
using UnityEngine;
/// <summary>压缩图片</summary>
public class ImageResizer
{
/// <summary>
/// 压缩并保存图片
/// </summary>
/// <param name="inputPath">图片源</param>
/// <param name="outputPath">修改后保存地址</param>
/// <param name="maxResolution">最大高度</param>
public void CompressAndSave(string inputPath, string outputPath,int maxResolution = 500)
{
Texture2D originalTexture = LoadTexture(inputPath);
if (originalTexture != null)
{
// 压缩图片并保存到指定路径
Texture2D compressedTexture = CompressTexture(originalTexture, maxResolution);
SaveTexture(compressedTexture, outputPath);
}
}
// 从文件加载纹理
private Texture2D LoadTexture(string path)
{
Texture2D texture = null;
try
{
byte[] fileData = File.ReadAllBytes(path);
texture = new Texture2D(2, 2, TextureFormat.RGBA32, false);
texture.LoadImage(fileData);
}
catch (IOException e)
{
Debug.LogError("Failed to load texture: " + e.Message);
}
return texture;
}
// 压缩纹理到目标大小
private Texture2D CompressTexture(Texture2D texture, int targetWidth)
{
// 计算目标高度,保持宽高比不变
int targetHeight = Mathf.RoundToInt((float)texture.height / texture.width * targetWidth);
Texture2D compressedTexture = new Texture2D(targetWidth, targetHeight, TextureFormat.RGBA32, false);
RenderTexture renderTexture = RenderTexture.GetTemporary(targetWidth, targetHeight);
Graphics.Blit(texture, renderTexture);
RenderTexture.active = renderTexture;
compressedTexture.ReadPixels(new Rect(0, 0, targetWidth, targetHeight), 0, 0);
RenderTexture.ReleaseTemporary(renderTexture);
return compressedTexture;
}
// 将纹理保存为文件
private void SaveTexture(Texture2D texture, string path)
{
byte[] bytes = texture.EncodeToPNG();
File.WriteAllBytes(path, bytes);
}
}
Unity等比压缩图片
原创
©著作权归作者所有:来自51CTO博客作者DaLiangChen的原创作品,请联系作者获取转载授权,否则将追究法律责任
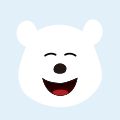
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
php等比例压缩图片
网上摘抄,还没做测试
php 系统函数 -
java等比压缩图片工具类
java等比压缩图片工具类
Image java System -
压缩图片
d
d