1 import java.util.*;2 import java.text.*;3 import java.util.Calendar;4
5 public classVeDate {6 /**7 * 获取现在时间8 *9 * @return 返回时间类型 yyyy-MM-dd HH:mm:ss10 */
11 public staticDate getNowDate() {12 Date currentTime = newDate();13 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");14 String dateString =formatter.format(currentTime);15 ParsePosition pos = new ParsePosition(8);16 Date currentTime_2 =formatter.parse(dateString, pos);17 returncurrentTime_2;18 }19
20 /**21 * 获取现在时间22 *23 * @return返回短时间格式 yyyy-MM-dd24 */
25 public staticDate getNowDateShort() {26 Date currentTime = newDate();27 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");28 String dateString =formatter.format(currentTime);29 ParsePosition pos = new ParsePosition(8);30 Date currentTime_2 =formatter.parse(dateString, pos);31 returncurrentTime_2;32 }33
34 /**35 * 获取现在时间36 *37 * @return返回字符串格式 yyyy-MM-dd HH:mm:ss38 */
39 public staticString getStringDate() {40 Date currentTime = newDate();41 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");42 String dateString =formatter.format(currentTime);43 returndateString;44 }45
46 /**47 * 获取现在时间48 *49 * @return 返回短时间字符串格式yyyy-MM-dd50 */
51 public staticString getStringDateShort() {52 Date currentTime = newDate();53 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");54 String dateString =formatter.format(currentTime);55 returndateString;56 }57
58 /**59 * 获取时间 小时:分;秒 HH:mm:ss60 *61 * @return62 */
63 public staticString getTimeShort() {64 SimpleDateFormat formatter = new SimpleDateFormat("HH:mm:ss");65 Date currentTime = newDate();66 String dateString =formatter.format(currentTime);67 returndateString;68 }69
70 /**71 * 将长时间格式字符串转换为时间 yyyy-MM-dd HH:mm:ss72 *73 * @param strDate74 * @return75 */
76 public staticDate strToDateLong(String strDate) {77 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");78 ParsePosition pos = new ParsePosition(0);79 Date strtodate =formatter.parse(strDate, pos);80 returnstrtodate;81 }82
83 /**84 * 将长时间格式时间转换为字符串 yyyy-MM-dd HH:mm:ss85 *86 * @param dateDate87 * @return88 */
89 public staticString dateToStrLong(java.util.Date dateDate) {90 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");91 String dateString =formatter.format(dateDate);92 returndateString;93 }94
95 /**96 * 将短时间格式时间转换为字符串 yyyy-MM-dd97 *98 * @param dateDate99 * @param k100 * @return101 */
102 public staticString dateToStr(java.util.Date dateDate) {103 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");104 String dateString =formatter.format(dateDate);105 returndateString;106 }107
108 /**109 * 将短时间格式字符串转换为时间 yyyy-MM-dd110 *111 * @param strDate112 * @return113 */
114 public staticDate strToDate(String strDate) {115 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");116 ParsePosition pos = new ParsePosition(0);117 Date strtodate =formatter.parse(strDate, pos);118 returnstrtodate;119 }120
121 /**122 * 得到现在时间123 *124 * @return125 */
126 public staticDate getNow() {127 Date currentTime = newDate();128 returncurrentTime;129 }130
131 /**132 * 提取一个月中的最后一天133 *134 * @param day135 * @return136 */
137 public static Date getLastDate(longday) {138 Date date = newDate();139 long date_3_hm = date.getTime() - 3600000 * 34 *day;140 Date date_3_hm_date = newDate(date_3_hm);141 returndate_3_hm_date;142 }143
144 /**145 * 得到现在时间146 *147 * @return 字符串 yyyyMMdd HHmmss148 */
149 public staticString getStringToday() {150 Date currentTime = newDate();151 SimpleDateFormat formatter = new SimpleDateFormat("yyyyMMdd HHmmss");152 String dateString =formatter.format(currentTime);153 returndateString;154 }155
156 /**157 * 得到现在小时158 */
159 public staticString getHour() {160 Date currentTime = newDate();161 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");162 String dateString =formatter.format(currentTime);163 String hour;164 hour = dateString.substring(11, 13);165 returnhour;166 }167
168 /**169 * 得到现在分钟170 *171 * @return172 */
173 public staticString getTime() {174 Date currentTime = newDate();175 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");176 String dateString =formatter.format(currentTime);177 String min;178 min = dateString.substring(14, 16);179 returnmin;180 }181
182 /**183 * 根据用户传入的时间表示格式,返回当前时间的格式 如果是yyyyMMdd,注意字母y不能大写。184 *185 * @param sformat186 * yyyyMMddhhmmss187 * @return188 */
189 public staticString getUserDate(String sformat) {190 Date currentTime = newDate();191 SimpleDateFormat formatter = newSimpleDateFormat(sformat);192 String dateString =formatter.format(currentTime);193 returndateString;194 }195
196 /**197 * 二个小时时间间的差值,必须保证二个时间都是"HH:MM"的格式,返回字符型的分钟198 */
199 public staticString getTwoHour(String st1, String st2) {200 String[] kk = null;201 String[] jj = null;202 kk = st1.split(":");203 jj = st2.split(":");204 if (Integer.parseInt(kk[0]) < Integer.parseInt(jj[0]))205 return "0";206 else{207 double y = Double.parseDouble(kk[0]) + Double.parseDouble(kk[1]) / 60;208 double u = Double.parseDouble(jj[0]) + Double.parseDouble(jj[1]) / 60;209 if ((y - u) > 0)210 return y - u + "";211 else
212 return "0";213 }214 }215
216 /**217 * 得到二个日期间的间隔天数218 */
219 public staticString getTwoDay(String sj1, String sj2) {220 SimpleDateFormat myFormatter = new SimpleDateFormat("yyyy-MM-dd");221 long day = 0;222 try{223 java.util.Date date =myFormatter.parse(sj1);224 java.util.Date mydate =myFormatter.parse(sj2);225 day = (date.getTime() - mydate.getTime()) / (24 * 60 * 60 * 1000);226 } catch(Exception e) {227 return "";228 }229 return day + "";230 }231
232 /**233 * 时间前推或后推分钟,其中JJ表示分钟.234 */
235 public staticString getPreTime(String sj1, String jj) {236 SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");237 String mydate1 = "";238 try{239 Date date1 =format.parse(sj1);240 long Time = (date1.getTime() / 1000) + Integer.parseInt(jj) * 60;241 date1.setTime(Time * 1000);242 mydate1 =format.format(date1);243 } catch(Exception e) {244 }245 returnmydate1;246 }247
248 /**249 * 得到一个时间延后或前移几天的时间,nowdate为时间,delay为前移或后延的天数250 */
251 public staticString getNextDay(String nowdate, String delay) {252 try{253 SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");254 String mdate = "";255 Date d =strToDate(nowdate);256 long myTime = (d.getTime() / 1000) + Integer.parseInt(delay) * 24 * 60 * 60;257 d.setTime(myTime * 1000);258 mdate =format.format(d);259 returnmdate;260 }catch(Exception e){261 return "";262 }263 }264
265 /**266 * 判断是否润年267 *268 * @param ddate269 * @return270 */
271 public staticboolean isLeapYear(String ddate) {272
273 /**274 * 详细设计: 1.被400整除是闰年,否则: 2.不能被4整除则不是闰年 3.能被4整除同时不能被100整除则是闰年275 * 3.能被4整除同时能被100整除则不是闰年276 */
277 Date d =strToDate(ddate);278 GregorianCalendar gc =(GregorianCalendar) Calendar.getInstance();279 gc.setTime(d);280 int year = gc.get(Calendar.YEAR);281 if ((year % 400) == 0)282 return true;283 else if ((year % 4) == 0) {284 if ((year % 100) == 0)285 return false;286 else
287 return true;288 } else
289 return false;290 }291
292 /**293 * 返回美国时间格式 26 Apr 2006294 *295 * @param str296 * @return297 */
298 public staticString getEDate(String str) {299 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");300 ParsePosition pos = new ParsePosition(0);301 Date strtodate =formatter.parse(str, pos);302 String j =strtodate.toString();303 String[] k = j.split(" ");304 return k[2] + k[1].toUpperCase() + k[5].substring(2, 4);305 }306
307 /**308 * 获取一个月的最后一天309 *310 * @param dat311 * @return312 */
313 public static String getEndDateOfMonth(String dat) {//yyyy-MM-dd
314 String str = dat.substring(0, 8);315 String month = dat.substring(5, 7);316 int mon =Integer.parseInt(month);317 if (mon == 1 || mon == 3 || mon == 5 || mon == 7 || mon == 8 || mon == 10 || mon == 12) {318 str += "31";319 } else if (mon == 4 || mon == 6 || mon == 9 || mon == 11) {320 str += "30";321 } else{322 if(isLeapYear(dat)) {323 str += "29";324 } else{325 str += "28";326 }327 }328 returnstr;329 }330
331 /**332 * 判断二个时间是否在同一个周333 *334 * @param date1335 * @param date2336 * @return337 */
338 public staticboolean isSameWeekDates(Date date1, Date date2) {339 Calendar cal1 =Calendar.getInstance();340 Calendar cal2 =Calendar.getInstance();341 cal1.setTime(date1);342 cal2.setTime(date2);343 int subYear = cal1.get(Calendar.YEAR) - cal2.get(Calendar.YEAR);344 if (0 ==subYear) {345 if (cal1.get(Calendar.WEEK_OF_YEAR) == cal2.get(Calendar.WEEK_OF_YEAR))346 return true;347 } else if (1 == subYear && 11 == cal2.get(Calendar.MONTH)) {348 //如果12月的最后一周横跨来年第一周的话则最后一周即算做来年的第一周
349 if (cal1.get(Calendar.WEEK_OF_YEAR) == cal2.get(Calendar.WEEK_OF_YEAR))350 return true;351 } else if (-1 == subYear && 11 == cal1.get(Calendar.MONTH)) {352 if (cal1.get(Calendar.WEEK_OF_YEAR) == cal2.get(Calendar.WEEK_OF_YEAR))353 return true;354 }355 return false;356 }357
358 /**359 * 产生周序列,即得到当前时间所在的年度是第几周360 *361 * @return362 */
363 public staticString getSeqWeek() {364 Calendar c =Calendar.getInstance(Locale.CHINA);365 String week = Integer.toString(c.get(Calendar.WEEK_OF_YEAR));366 if (week.length() == 1)367 week = "0" +week;368 String year = Integer.toString(c.get(Calendar.YEAR));369 return year +week;370 }371
372 /**373 * 获得一个日期所在的周的星期几的日期,如要找出2002年2月3日所在周的星期一是几号374 *375 * @param sdate376 * @param num377 * @return378 */
379 public staticString getWeek(String sdate, String num) {380 //再转换为时间
381 Date dd =VeDate.strToDate(sdate);382 Calendar c =Calendar.getInstance();383 c.setTime(dd);384 if (num.equals("1")) //返回星期一所在的日期
385 c.set(Calendar.DAY_OF_WEEK, Calendar.MONDAY);386 else if (num.equals("2")) //返回星期二所在的日期
387 c.set(Calendar.DAY_OF_WEEK, Calendar.TUESDAY);388 else if (num.equals("3")) //返回星期三所在的日期
389 c.set(Calendar.DAY_OF_WEEK, Calendar.WEDNESDAY);390 else if (num.equals("4")) //返回星期四所在的日期
391 c.set(Calendar.DAY_OF_WEEK, Calendar.THURSDAY);392 else if (num.equals("5")) //返回星期五所在的日期
393 c.set(Calendar.DAY_OF_WEEK, Calendar.FRIDAY);394 else if (num.equals("6")) //返回星期六所在的日期
395 c.set(Calendar.DAY_OF_WEEK, Calendar.SATURDAY);396 else if (num.equals("0")) //返回星期日所在的日期
397 c.set(Calendar.DAY_OF_WEEK, Calendar.SUNDAY);398 return new SimpleDateFormat("yyyy-MM-dd").format(c.getTime());399 }400
401 /**402 * 根据一个日期,返回是星期几的字符串403 *404 * @param sdate405 * @return406 */
407 public staticString getWeek(String sdate) {408 //再转换为时间
409 Date date =VeDate.strToDate(sdate);410 Calendar c =Calendar.getInstance();411 c.setTime(date);412 //int hour=c.get(Calendar.DAY_OF_WEEK);413 //hour中存的就是星期几了,其范围 1~7414 //1=星期日 7=星期六,其他类推
415 return new SimpleDateFormat("EEEE").format(c.getTime());416 }417 public staticString getWeekStr(String sdate){418 String str = "";419 str =VeDate.getWeek(sdate);420 if("1".equals(str)){421 str = "星期日";422 }else if("2".equals(str)){423 str = "星期一";424 }else if("3".equals(str)){425 str = "星期二";426 }else if("4".equals(str)){427 str = "星期三";428 }else if("5".equals(str)){429 str = "星期四";430 }else if("6".equals(str)){431 str = "星期五";432 }else if("7".equals(str)){433 str = "星期六";434 }435 returnstr;436 }437
438 /**439 * 两个时间之间的天数440 *441 * @param date1442 * @param date2443 * @return444 */
445 public static longgetDays(String date1, String date2) {446 if (date1 == null || date1.equals(""))447 return 0;448 if (date2 == null || date2.equals(""))449 return 0;450 //转换为标准时间
451 SimpleDateFormat myFormatter = new SimpleDateFormat("yyyy-MM-dd");452 java.util.Date date = null;453 java.util.Date mydate = null;454 try{455 date =myFormatter.parse(date1);456 mydate =myFormatter.parse(date2);457 } catch(Exception e) {458 }459 long day = (date.getTime() - mydate.getTime()) / (24 * 60 * 60 * 1000);460 returnday;461 }462
463 /**464 * 形成如下的日历 , 根据传入的一个时间返回一个结构 星期日 星期一 星期二 星期三 星期四 星期五 星期六 下面是当月的各个时间465 * 此函数返回该日历第一行星期日所在的日期466 *467 * @param sdate468 * @return469 */
470 public staticString getNowMonth(String sdate) {471 //取该时间所在月的一号
472 sdate = sdate.substring(0, 8) + "01";473
474 //得到这个月的1号是星期几
475 Date date =VeDate.strToDate(sdate);476 Calendar c =Calendar.getInstance();477 c.setTime(date);478 int u = c.get(Calendar.DAY_OF_WEEK);479 String newday = VeDate.getNextDay(sdate, (1 - u) + "");480 returnnewday;481 }482
483 /**484 * 取得数据库主键 生成格式为yyyymmddhhmmss+k位随机数485 *486 * @param k487 * 表示是取几位随机数,可以自己定488 */
489
490 public static String getNo(intk) {491
492 return getUserDate("yyyyMMddhhmmss") +getRandom(k);493 }494
495 /**496 * 返回一个随机数497 *498 * @param i499 * @return500 */
501 public static String getRandom(inti) {502 Random jjj = newRandom();503 //int suiJiShu = jjj.nextInt(9);
504 if (i == 0)505 return "";506 String jj = "";507 for (int k = 0; k < i; k++) {508 jj = jj + jjj.nextInt(9);509 }510 returnjj;511 }512
513 /**514 *515 * @param args516 */
517 public staticboolean RightDate(String date) {518
519 SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");520 ;521 if (date == null)522 return false;523 if (date.length() > 10) {524 sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");525 } else{526 sdf = new SimpleDateFormat("yyyy-MM-dd");527 }528 try{529 sdf.parse(date);530 } catch(ParseException pe) {531 return false;532 }533 return true;534 }535
536 /***************************************************************************537 * //nd=1表示返回的值中包含年度 //yf=1表示返回的值中包含月份 //rq=1表示返回的值中包含日期 //format表示返回的格式 1538 * 以年月日中文返回 2 以横线-返回 // 3 以斜线/返回 4 以缩写不带其它符号形式返回 // 5 以点号.返回539 **************************************************************************/
540 public staticString getStringDateMonth(String sdate, String nd, String yf, String rq, String format) {541 Date currentTime = newDate();542 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");543 String dateString =formatter.format(currentTime);544 String s_nd = dateString.substring(0, 4); //年份
545 String s_yf = dateString.substring(5, 7); //月份
546 String s_rq = dateString.substring(8, 10); //日期
547 String sreturn = "";548 roc.util.MyChar mc = newroc.util.MyChar();549 if (sdate == null || sdate.equals("") || !mc.Isdate(sdate)) { //处理空值情况
550 if (nd.equals("1")) {551 sreturn =s_nd;552 //处理间隔符
553 if (format.equals("1"))554 sreturn = sreturn + "年";555 else if (format.equals("2"))556 sreturn = sreturn + "-";557 else if (format.equals("3"))558 sreturn = sreturn + "/";559 else if (format.equals("5"))560 sreturn = sreturn + ".";561 }562 //处理月份
563 if (yf.equals("1")) {564 sreturn = sreturn +s_yf;565 if (format.equals("1"))566 sreturn = sreturn + "月";567 else if (format.equals("2"))568 sreturn = sreturn + "-";569 else if (format.equals("3"))570 sreturn = sreturn + "/";571 else if (format.equals("5"))572 sreturn = sreturn + ".";573 }574 //处理日期
575 if (rq.equals("1")) {576 sreturn = sreturn +s_rq;577 if (format.equals("1"))578 sreturn = sreturn + "日";579 }580 } else{581 //不是空值,也是一个合法的日期值,则先将其转换为标准的时间格式
582 sdate =roc.util.RocDate.getOKDate(sdate);583 s_nd = sdate.substring(0, 4); //年份
584 s_yf = sdate.substring(5, 7); //月份
585 s_rq = sdate.substring(8, 10); //日期
586 if (nd.equals("1")) {587 sreturn =s_nd;588 //处理间隔符
589 if (format.equals("1"))590 sreturn = sreturn + "年";591 else if (format.equals("2"))592 sreturn = sreturn + "-";593 else if (format.equals("3"))594 sreturn = sreturn + "/";595 else if (format.equals("5"))596 sreturn = sreturn + ".";597 }598 //处理月份
599 if (yf.equals("1")) {600 sreturn = sreturn +s_yf;601 if (format.equals("1"))602 sreturn = sreturn + "月";603 else if (format.equals("2"))604 sreturn = sreturn + "-";605 else if (format.equals("3"))606 sreturn = sreturn + "/";607 else if (format.equals("5"))608 sreturn = sreturn + ".";609 }610 //处理日期
611 if (rq.equals("1")) {612 sreturn = sreturn +s_rq;613 if (format.equals("1"))614 sreturn = sreturn + "日";615 }616 }617 returnsreturn;618 }619
620 public static String getNextMonthDay(String sdate, intm) {621 sdate =getOKDate(sdate);622 int year = Integer.parseInt(sdate.substring(0, 4));623 int month = Integer.parseInt(sdate.substring(5, 7));624 month = month +m;625 if (month < 0) {626 month = month + 12;627 year = year - 1;628 } else if (month > 12) {629 month = month - 12;630 year = year + 1;631 }632 String smonth = "";633 if (month < 10)634 smonth = "0" +month;635 else
636 smonth = "" +month;637 return year + "-" + smonth + "-10";638 }639
640 public staticString getOKDate(String sdate) {641 if (sdate == null || sdate.equals(""))642 returngetStringDateShort();643
644 if (!VeStr.Isdate(sdate)) {645 sdate =getStringDateShort();646 }647 //将“/”转换为“-”
648 sdate = VeStr.Replace(sdate, "/", "-");649 //如果只有8位长度,则要进行转换
650 if (sdate.length() == 8)651 sdate = sdate.substring(0, 4) + "-" + sdate.substring(4, 6) + "-" + sdate.substring(6, 8);652 SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");653 ParsePosition pos = new ParsePosition(0);654 Date strtodate =formatter.parse(sdate, pos);655 String dateString =formatter.format(strtodate);656 returndateString;657 }658
659 public static voidmain(String[] args) throws Exception {660 try{661 //System.out.print(Integer.valueOf(getTwoDay("2006-11-03 12:22:10", "2006-11-02 11:22:09")));
662 } catch(Exception e) {663 throw newException();664 }665 //System.out.println("sss");
666 }
java object时间格式转时间 java时间日期格式转换
转载文章标签 java object时间格式转时间 java转换时间类型 java 时间格式 整除 文章分类 Java 后端开发
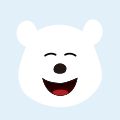
-
java时间日期格式 java的时间格式
SimpleDateFormat日期和时间格式由日期和时间模式字符串指定。
java 日期格式 java System 字符串 -
flume采集hbase
一,Flume的描述1、Flume的概念Flume是分布式的日志收集系统,它将各个服务器中的数据收集起来并送到指定的地方去,比如说送到HDFS,Kafka,MySql;简单来说flume就是收集日志的。 2、Event的概念 Flume中event的相关概念:Flume的核心是把数据从数据源(source)收集过来,在将收集到的数据送到指定的目的地(sink)。为
flume采集hbase 分布式的采集文件系统 Flume的原理和架构 Flume的基本使用 数据