<?php
namespace Cart;
/**
* 顾客类
* @package Cart
*/
class Customer {
// 顾客id
private $customer_id;
// 名字
private $firstname;
// 姓氏
private $lastname;
// 分组id
private $customer_group_id;
//邮箱
private $email;
// 电话
private $telephone;
// 通讯
private $newsletter;
// 地址id
private $address_id;
public function __construct($registry) {
// 注册配置类
$this->config = $registry->get('config');
// 注册数据库类
$this->db = $registry->get('db');
// 注册请求类
$this->request = $registry->get('request');
// 注册session类
$this->session = $registry->get('session');
if (isset($this->session->data['customer_id'])) {
// 查询顾客信息
$customer_query = $this->db->query("SELECT * FROM " . DB_PREFIX . "customer WHERE customer_id = '" . (int)$this->session->data['customer_id'] . "' AND status = '1'");
if ($customer_query->num_rows) {
$this->customer_id = $customer_query->row['customer_id'];
$this->firstname = $customer_query->row['firstname'];
$this->lastname = $customer_query->row['lastname'];
$this->customer_group_id = $customer_query->row['customer_group_id'];
$this->email = $customer_query->row['email'];
$this->telephone = $customer_query->row['telephone'];
$this->newsletter = $customer_query->row['newsletter'];
$this->address_id = $customer_query->row['address_id'];
$this->db->query("UPDATE " . DB_PREFIX . "customer SET language_id = '" . (int)$this->config->get('config_language_id') . "', ip = '" . $this->db->escape($this->request->server['REMOTE_ADDR']) . "' WHERE customer_id = '" . (int)$this->customer_id . "'");
} else {
$this->logout();
}
}
}
// 登陆
public function login($email, $password, $override = false) {
$customer_query = $this->db->query("SELECT * FROM " . DB_PREFIX . "customer WHERE LOWER(email) = '" . $this->db->escape(utf8_strtolower($email)) . "' AND status = '1'");
if ($customer_query->num_rows) {
if (!$override) {
// 验证密码和散列值是否匹配
if (password_verify($password, $customer_query->row['password'])) {
// 重新加密
if (password_needs_rehash($customer_query->row['password'], PASSWORD_DEFAULT)) {
// 对密码加密
$new_password_hashed = password_hash($password, PASSWORD_DEFAULT);
}
} elseif ($customer_query->row['password'] == sha1($customer_query->row['salt'] . sha1($customer_query->row['salt'] . sha1($password))) || $customer_query->row['password'] == md5($password)) {
$new_password_hashed = password_hash($password, PASSWORD_DEFAULT);
} else {
return false;
}
}
$this->session->data['customer_id'] = $customer_query->row['customer_id'];
$this->customer_id = $customer_query->row['customer_id'];
$this->firstname = $customer_query->row['firstname'];
$this->lastname = $customer_query->row['lastname'];
$this->customer_group_id = $customer_query->row['customer_group_id'];
$this->email = $customer_query->row['email'];
$this->telephone = $customer_query->row['telephone'];
$this->newsletter = $customer_query->row['newsletter'];
$this->address_id = $customer_query->row['address_id'];
$this->db->query("UPDATE " . DB_PREFIX . "customer SET " . ((isset($new_password_hashed)) ? "salt = '', password = '" . $this->db->escape($new_password_hashed) . "', " : "") . "language_id = '" . (int)$this->config->get('config_language_id') . "', ip = '" . $this->db->escape($this->request->server['REMOTE_ADDR']) . "' WHERE customer_id = '" . (int)$this->customer_id . "'");
return true;
} else {
return false;
}
}
// 退出
public function logout() {
// 清除session
unset($this->session->data['customer_id']);
$this->customer_id = '';
$this->firstname = '';
$this->lastname = '';
$this->customer_group_id = '';
$this->email = '';
$this->telephone = '';
$this->newsletter = '';
$this->address_id = '';
}
// 是否登录
public function isLogged() {
return $this->customer_id;
}
// 获取顾客id
public function getId() {
return $this->customer_id;
}
// 获取名字
public function getFirstName() {
return $this->firstname;
}
// 获取姓氏
public function getLastName() {
return $this->lastname;
}
// 获取分组id
public function getGroupId() {
return $this->customer_group_id;
}
// 获取邮箱
public function getEmail() {
return $this->email;
}
// 获取电话
public function getTelephone() {
return $this->telephone;
}
// 获取通讯信息
public function getNewsletter() {
return $this->newsletter;
}
public function getAddressId() {
return $this->address_id;
}
// 交易总额
public function getBalance() {
$query = $this->db->query("SELECT SUM(amount) AS total FROM " . DB_PREFIX . "customer_transaction WHERE customer_id = '" . (int)$this->customer_id . "'");
return $query->row['total'];
}
// 增送积分
public function getRewardPoints() {
$query = $this->db->query("SELECT SUM(points) AS total FROM " . DB_PREFIX . "customer_reward WHERE customer_id = '" . (int)$this->customer_id . "'");
return $query->row['total'];
}
}
【opencart3源码分析】顾客类customer.php
原创文章标签 opencart源码分析 opencart顾客类 ide php 数据库类 文章分类 代码人生
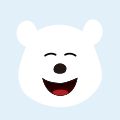
-
iOS 接入 FaceBook
1. 创建本地工程 路径:/Users/XXX/Documents/OC-PrivatePods2. 在码云(https://gitee.com)上创建一个自己的远程私有索引库,用来存放私有框架的详细描述信息.podspec文件 3.&n
iOS 接入 FaceBook 私有库 CocoaPods 码云 iOS