<?php namespace Laravel;
// /laravel/laravel.php
/**
* Bootstrap the core framework components like the IoC container and
* the configuration class, and the class auto-loader. Once this file
* has run, the framework is essentially ready for use.
* 启动核心框架组件,像IOC容器和配置类,以及自动加载类。一旦这个文件运行,框架就基本可以使用了
*/
require 'core.php';
/**
* Register the PHP exception handler. The framework throws exceptions
* on every error that cannot be handled. All of those exceptions will
* be sent through this closure for processing.
* 注册异常处理器。当任何不能处理的错误发生时框架就抛出异常,所有这些异常将被发送到闭包中处理
*/
// set_exception_handler() 自定义异常处理函数
set_exception_handler(function($e)
{
// 调用错误类函数exception处理异常
Error::exception($e);
});
/**
* Register the PHP error handler. All PHP errors will fall into this
* handler which will convert the error into an ErrorException object
* and pass the exception into the exception handler.
* 注册PHP错误处理器。所有的PHP错误都将放入这个处理器,将错误转为ErrorException对象,然后将这个异常传递给处理器进行处理
*/
set_error_handler(function($code, $error, $file, $line)
{
// 处理原生的PHP错误
Error::native($code, $error, $file, $line);
});
/**
* Register the shutdown handler. This function will be called at the
* end of the PHP script or on a fatal PHP error. If a PHP error has
* occured, we will convert it to an ErrorException and pass it
* to the common exception handler for the framework.
* 注册关闭处理器。这个函数将在PHP脚本运行结束或者发证致命的PHP错误时被调用。
* 如果PHP错误发生了,将会把它转为一个ErrorException,然后将它作为参数传递给框架的公共异常处理器
*/
// register_shutdown_function() 注册一个在PHP中止时执行的函数
register_shutdown_function(function()
{
Error::shutdown();
});
/**
* Setting the PHP error reporting level to -1 essentially forces
* PHP to report every error, and it is guranteed to show every
* error on future versions of PHP.
*
* If error detail is turned off, we will turn off all PHP error
* reporting and display since the framework will be displaying
* a generic message and we do not want any sensitive details
* about the exception leaking into the views.
*
*/
// 报告所有PHP错误
error_reporting(-1);
// init_set()为配置项设置值, display_errors 是否显示错误 在application/config/errors中配置display, 默认关闭
ini_set('display_errors', Config::get('error.display'));
/**
* Determine if we need to set the application key to a very random
* string so we can provide a zero configuration installation but
* still ensure that the key is set to something random. It is
* possible to disable this feature.
* 判断是否需要给应用设置一个随机的字符串作为应用key,这样我们可以零配置安装,而且还能确保key是随机的。未来也可能不可用。
*/
// 在application/config/application.php中配置auto_key
$auto_key = Config::get('application.auto_key');
// 若auto_key为true 且key为空,则自动生成key
if ($auto_key and Config::get('application.key') == '')
{
// ob_start() 打开输出控制缓冲
ob_start() and with(new CLI\Tasks\Key)->generate();
// ob_end_clean() 清空缓冲区并关闭输出缓冲
ob_end_clean();
}
/**
* Even though "Magic Quotes" are deprecated in PHP 5.3, they may
* still be enabled on the server. To account for this, we will
* strip slashes on all input arrays if magic quotes are turned
* on for the server environment.
* 即使Magic Quotes 在php5.3被废弃了,它们可能还能使用,未来解决这个问题,
* 如果服务器环境magic quotes开启的话,我们在所有输入数组添加斜杠
*/
// php5.3之后magic_quotes() 返回false
if (magic_quotes())
{
$magics = array(&$_GET, &$_POST, &$_COOKIE, &$_REQUEST);
foreach ($magics as &$magic)
{
// array_strop_slashed() 函数在helpers.php中
// 遍历数组,删除斜杠
$magic = array_strip_slashes($magic);
}
}
/**
* Load the session using the session manager. The payload will
* be set on a static property of the Session class for easy
* access throughout the framework and application.
* 用session管理器加载session,
* 设置成Session类的静态属性上,为了框架和应用更容易使用它
*/
if (Config::get('session.driver') !== '')
{
Session::start(Config::get('session.driver'));
Session::load(Cookie::get(Config::get('session.cookie')));
}
/**
* Gather the input to the application based on the global input
* variables for the current request. The input will be gathered
* based on the current request method and will be set on the
* Input manager class' static $input property.
* 定义全局input遍历手机应用的输入。
* 当前的请求方法将被设置在Input管理类的静态input属性
*/
$input = array();
switch (Request::method())
{
case 'GET':
$input = $_GET;
break;
case 'POST':
$input = $_POST;
break;
case 'PUT':
case 'DELETE':
if (Request::spoofed())
{
$input = $_POST;
}
else
{
// file_get_contents() 将整个文件读入一个字符串
// parse_str() 将字符串解析成多个变量
parse_str(file_get_contents('php://input'), $input);
if (magic_quotes()) $input = array_strip_slashes($input);
}
}
/**
* The spoofed request method is removed from the input so it is not
* unexpectedly included in Input::all() or Input::get(). Leaving it
* in the input array could cause unexpected results if an Eloquent
* model is filled with the input.
* 伪造的请求方法将被从inout中移除,被包含在Input:all()或者Input::get()并不意外。
* 如果一个Eloquent模型被填充到input中,放在input数组中可能造成意外的结果。
*/
// 释放伪造的请求方法
unset($input[Request::spoofer]);
Input::$input = $input;
/**
* Load the "application" bundle. Though the application folder is
* not typically considered a bundle, it is started like one and
* essentially serves as the "default" bundle.
* 加载application
* 尽管通常不将application文件夹视为绑定,实际上充当一个默认的绑定进行启动
*/
Bundle::start(DEFAULT_BUNDLE);
/**
* Auto-start any bundles configured to start on every request.
* This is especially useful for debug bundles or bundles that
* are used throughout the application.
* 对于每一个请求将自动启动配置中的绑定
* 这对于调试绑定或整个应用程序中使用的绑定特别有用。
*/
foreach (Bundle::$bundles as $bundle => $config)
{
if ($config['auto']) Bundle::start($bundle);
}
/**
* Register the "catch-all" route that handles 404 responses for
* routes that can not be matched to any other route within the
* application. We'll just raise the 404 event.
* 注册catch-all路由,用来匹配应用中无法匹配的任何路由,从而进行404响应
* 只触发404事件
*/
Routing\Router::register('*', '(:all)', function()
{
return Event::first('404');
});
/**
* If the requset URI has too many segments, we will bomb out of
* the request. This is too avoid potential DDoS attacks against
* the framework by overloading the controller lookup method
* with thousands of segments.
* 如果请求URI中分段过多,我们将终止请求
* 这也避免了通过数千个网段重载控制器查找方法进行DDos攻击
*/
$uri = URI::current();
// URL分段大于15抛出异常
if (count(URI::$segments) > 15)
{
throw new \Exception("Invalid request. Too many URI segments.");
}
/**
* Route the request to the proper route in the application. If a
* route is found, the route will be called via the request class
* static property. If no route is found, the 404 response will
* be returned to the browser.
* 将请求路由到应用中正确的路由
* 如果一个路由被找到了,这个路由将被通过请求类的静态属性调用
* 如果路由没有找到,将给浏览器返回404响应
*/
Request::$route = Routing\Router::route(Request::method(), $uri);
$response = Request::$route->call();
/**
* Close the session and write the active payload to persistent
* storage. The session cookie will also be written and if the
* driver is a sweeper, session garbage collection might be
* performed depending on the "sweepage" probability.
* 关闭会话,然后将活动的有效负载写入持久性存储。
* 会话cookie也将被写入,如果驱动程序是扫描程序,则可能会根据“溢出”概率执行会话垃圾回收。
*/
if (Config::get('session.driver') !== '')
{
Session::save();
}
/**
* Send all of the cookies to the browser. The cookies are
* stored in a "jar" until the end of a request, primarily
* to make testing the cookie functionality of the site
* much easier since the jar can be inspected.
* 发送所有的cookie到浏览器。
* Cookies被存储在jar中,直到请求结束,主要是为了测试网站的cookie功能比jar更容易被侦测到
*/
Cookie::send();
/**
* Send the final response to the browser and fire the
* final event indicating that the processing for the
* current request is completed.
* 发送最终的响应到浏览器,而且触发最终的事件来表明当前处理中的请求已经完成
*/
$response->send();
Event::fire('laravel.done');
【Laravel3.0.0源码阅读分析】启动文件larave.php
原创深漂小码哥 ©著作权
文章标签 PHP Laravel源码分析 文章分类 后端开发
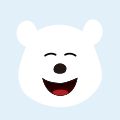
-
Apache Doris 聚合函数源码阅读与解析|源码解读系列
Apache Doris Active Contributor 隐形通过本文记录下对源码的理解,以方便新人快速上手源码开发。
Apache Doris 数据库 大数据 数据分析 数据仓库