#include <iostream>
#include<malloc.h>
#include<cstdio>
using namespace std;
typedef char elemtype;
typedef struct lnode{
elemtype data;
struct lnode *next;
}linknode;
void createlistf(linknode* &l,elemtype a[],int n)
{
linknode *s;
l=(linknode*)malloc(sizeof(linknode));
l->next=NULL;
for(int i=0;i<n;i++)
{
s=(linknode*)malloc(sizeof(linknode));
s->data=a[i];
s->next=l->next;
l->next=s;
}
}
void createlistr(linknode* &l,elemtype a[],int n)
{
linknode *s,*r;
l=(linknode*)malloc(sizeof(linknode));
l->next=NULL;
r=l;
for(int i=0;i<n;i++)
{
s=(linknode*)malloc(sizeof(linknode));
s->data=a[i];
r->next=s;
r=s;
}
r->next=NULL;
}
void initlist(linknode *&l)
{
l=(linknode*)malloc(sizeof(linknode));
l->next=NULL;
}
void destroylist(linknode *&l)
{
linknode *pre=l,*p=pre->next;
while(p!=NULL)
{
free(pre);
pre=p;
p=pre->next;
}
free(pre);
}
bool listempty(linknode *l)
{
return(l->next==NULL);
}
int listlength(linknode *l)
{
int i=0;
linknode *p=l;
while(p->next!=NULL)
{
i++;
p=p->next;
}
return i;
}
void displist(linknode *l)
{
linknode *p=l->next;
while(p!=NULL)
{
printf("%c ",p->data);
p=p->next;
}
cout<<endl;
}
bool getelem(linknode *l,int i,elemtype &e)
{
int j=0;
linknode *p=l;
if(i<=0) return false;
while(j<i&&p!=NULL)
{
j++;
p=p->next;
}
if(p==NULL)
return false;
else
{
e=p->data;
return true;
}
}
int locateelem(linknode *l,elemtype e)
{
int i=1;
linknode *p=l->next;
while(p!=NULL&&p->data!=e)
{
p=p->next;
i++;
}
if(p==NULL)
return 0;
else
return i;
}
bool listinsert(linknode* &l,int i,elemtype e)
{
int j=0;
linknode *p=l,*s;
if(i<=0) return false;
while(j<i-1&&p!=NULL)
{
j++;
p=p->next;
}
if(p==NULL)
return false;
else
{
s=(linknode*)malloc(sizeof(linknode));
s->data=e;
s->next=p->next;
p->next=s;
return true;
}
}
bool listdelete(linknode * &l,int i,elemtype e)
{
int j=0;
linknode *p=l,*q;
if(i<=0) return false;
while(j<i-1&&p!=NULL)
{
j++;
p=p->next;
}
if(p==NULL)
{
return false;
}
else
{
q=p->next;
if(q==NULL)
return false;
e=q->data;
p->next=q->next;
free(q);
return true;
}
}
int main() {
linknode *h;
elemtype e;
cout<<"单链表基本运算如下"<<endl;
cout<<"初始化"<<endl;
initlist(h);
cout<<"尾插法"<<endl;
listinsert(h,1,'a');
listinsert(h,2,'b');
listinsert(h,3,'c');
listinsert(h,4,'d');
listinsert(h,5,'e');
cout<<"输出单链表"<<endl;
displist(h);
cout<<"长度"<<endl;
int j=listlength(h);
cout<<j<<endl;
printf("单链表为%s\n",(listempty(h)?"空":"非空"));
getelem(h,3,e);
cout<<"第三个元素是:"<<e<<endl;
int weizhi=locateelem(h,'a');
cout<<"元素a的位置是"<<weizhi<<endl;
cout<<"在第4个位置上插入f元素"<<endl;
listinsert(h,4,'f');\
displist(h);
cout<<"删除第三个元素"<<endl;
listdelete(h,3,e);
displist(h);
cout<<"释放"<<endl;
destroylist(h);
return 0;
}
线性表——单链表的各种操作
原创
©著作权归作者所有:来自51CTO博客作者李响Superb的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:堆排序最坏时间复杂度
下一篇:线性表——顺序表操作详解
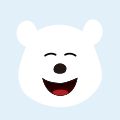
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】详细剖析线性表
【数据结构】第二章——线性表(9)总结了线性表的基本知识点,并对循序表与链表进行了比较
数据结构 C语言 线性表 顺序表 链表 -
1.链式存储的线性表——C语言实现
本文是作者学习数据结构过程中在单链表基本运算代码实现时遇到问题并解决问题后的结果
单链表 基本运算 存储结构 代码实现 -
链表(线性表)
Home Web Board ProblemSet Standing
#include 链表 结点