3.2.1快速排序
原创
©著作权归作者所有:来自51CTO博客作者塞上江南o的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:sql server多行插入
下一篇:pandas 布尔索引
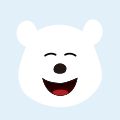
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
排序算法之计数排序的优化
排序算法之计数排序的优化
数组 计数排序 最小值 -
快速排序python代码 python 快速排序
简介 快速排序(Quick Sort)是对冒泡排序的一种改进,其基本思想:选一基准元素,依次将剩余元素中小于该基准元素的值放置其左侧,大于等于该基准元素的值放置其右侧;然后,取基准元素的前半部分和后半部分分别进行同样的处理;以此类推,直至各子序列剩余一个元素时,即排序完成(类比二叉树的思想)。 算法实现步骤首先设定一个分界值(pivot),通过该分界值将数组分成左右两部分。
快速排序python代码 python 快速排序 python快速排序 冒泡和快速排序的时间复杂度 实现快速排序的算法