/** 生成总和固定的随机数组
* @param total -- 必填:数据总和
* @param size -- 非必填,不指定时传 null:数据条目(要生成多少条数据)
* @param min -- 非必填:随机数的最小值
* @param max -- 非必填:随机数的最大值
* this.isEmpty() 封装的判断数据是否为空的方法
* this.getRandomNumber() 封装的生成随机整数的方法
* this.AddFun() 封装的加法函数
* this.SubFun() 封装的减法函数
* this.MulFun() 封装的乘法函数
* this.DivFun() 封装的除法函数
*/
function getRandom(total, size, min, max) {
let quantity = size; // 要生成多少条数据
let numArr = []; // 生成的数组
let minNum = !this.isEmpty(min) ? min : 0; // 未指定最小值默认为0,否则使用指定值
let maxNum = !this.isEmpty(max) ? max : total; // 未指定最大值默认使用数据总和,否则使用指定值
// 生成保留两位小数的随机数
function calcRandomNum(min, max) {
return parseFloat((Math.random() * (max - min + 1) + min).toFixed(2));
}
// 指定数据条目
if (!this.isEmpty(quantity)) {
let totalNum = total; // 要生成的数据总和
let average = this.DivFun(total, quantity); // 获取平均数
let length = quantity; // 控制循环的变量
let tempArr = [];
while (length > 1) {
let rnd = calcRandomNum(minNum, average); // 生成一个最大不超过平均数的随机数
totalNum = this.SubFun(totalNum, rnd); // 从数据总和中移除已生成数据
// numArr.push(rnd); // 将已生成数据存入结果数组
tempArr.push(rnd);
length--; // 控制循环
}
// 为防止最后一条数据与其他数据差值过大,将最后一条数据再进行拆分,追加到已生成的数据中
let lastNum = totalNum; // 存储最后的一条数据
let lastAverage = this.DivFun(lastNum, quantity - 1); // 计算最后一条数据在已生成数量下的平均数
if (totalNum > 0) {
for (let i = 0; i < tempArr.length; i++) {
let addNum = calcRandomNum(0, lastAverage); // 生成最大值不超过平均数的随机数
tempArr[i] = this.AddFun(tempArr[i], addNum); // 向已生成数据中追加生成的随机数
totalNum = this.SubFun(totalNum, addNum); // 在最后一条数据中删除生成的随机数
}
}
numArr = numArr.concat(tempArr); // 数据整合
numArr.push(totalNum); // 数据整合
let position = this.getRandomNumber(0, quantity - 2); // 随机数组中除最后一位的位置
let last = numArr.splice(-1)[0]; // 存储最后一位的值
let randItem = numArr[position]; // 存储随机出来的位置的值
numArr[position] = last; // 数值替换
numArr[quantity - 1] = randItem; // 数值替换
} else {
// 未指定数据条目
let totalNum = total; // 要生成的数据总和
for (let i = 0; i < totalNum; i++) {
let rnd = 0;
// 最大值小于需随机的数据和时,生成随机数
if (maxNum < totalNum) {
rnd = parseFloat(
(Math.random() * (maxNum - minNum + 1) + minNum).toFixed(2)
);
} else {
// 最大值大于等于需随机的数据和时,随机数的值为数据总和
rnd = totalNum;
}
// 生成随机数
totalNum = this.SubFun(totalNum, rnd); // 从数据总和中移除已生成数据
numArr.push(rnd); // 将已生成数据存入结果数组
}
}
return numArr;
},
JS生成固定和的随机数组(可指定/不指定数组长度)
原创
©著作权归作者所有:来自51CTO博客作者Double杨的原创作品,请联系作者获取转载授权,否则将追究法律责任
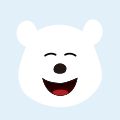
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
文心一言智能体创建教程——快速生成一个自己的专属智能体
文心一言智能体创建教程——快速生成一个自己的专属智能体
人工智能 沉浸感 决策制定