import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
/**
* XML格式字符串转换为Map
* @作者 廖正瀚
* @日期 2017年12月1日
* @param xml
* @param charset
* @return
* @throws DocumentException
* @throws UnsupportedEncodingException
*/
public static Map<String, String> xmlToMap(String xml, String charset) throws UnsupportedEncodingException, DocumentException{
Map<String, String> respMap = new HashMap<String, String>();
SAXReader reader = new SAXReader();
Document doc = reader.read(new ByteArrayInputStream(xml.getBytes(charset)));
Element root = doc.getRootElement();
xmlToMap(root, respMap);
return respMap;
}
public static Map<String, String> xmlToMap(Element tmpElement, Map<String, String> respMap){
if (tmpElement.isTextOnly()) {
respMap.put(tmpElement.getName(), tmpElement.getText());
return respMap;
}
@SuppressWarnings("unchecked")
Iterator<Element> eItor = tmpElement.elementIterator();
while (eItor.hasNext()) {
Element element = eItor.next();
xmlToMap(element, respMap);
}
return respMap;
}
java转xml字符串 xml字符串转map
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
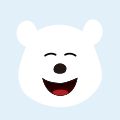
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
java json字符串转xml对象 java json字符串转map
json字符串转map,JSON字符串不能直接转化为map对象,要想取得map中的键对应的值需要别的方式java中JSON可以有两种格式,一种是对象格式的,另一种是数组对象,
java json字符串转xml对象 java json格式字符串转为map JSON json java