ImageView currentImage;
int dpScale;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(id.toolbar);
setSupportActionBar(toolbar);
DisplayMetrics metrics = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(metrics);
dpScale = (int)metrics.density;
FloatingActionButton fab = (FloatingActionButton) findViewById(id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Snackbar.make(view, “Replace with your own action”, Snackbar.LENGTH_LONG)
.setAction(“Action”, null).show();
}
});
//动态绘制64个带不同图片的网格
addImageView();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.Game_difficult) {
return true;
}
return super.onOptionsItemSelected(item);
}
public void addImageView(){
gridView = (GridLayout)findViewById(id.gridLayout);
for(int i = 0; i < 64; i++){
imageView = new ImageView(this);
//为每一个网格设置一个ID,然后给每一个网格附随机的图片,网格与图片之间是相互分离的
imageView.setId(i);
//随机产生一个数,然后通过这个数去获取图片
int random = getRandom();
switch (random){
case 1:
imageView.setBackgroundResource(drawable.icon_1);
break;
case 2:
imageView.setBackgroundResource(drawable.icon_2);
break;
case 3:
imageView.setBackgroundResource(drawable.icon_3);
break;
case 4:
imageView.setBackgroundResource(drawable.icon_4);
break;
case 5:
imageView.setBackgroundResource(drawable.icon_5);
break;
case 6:
imageView.setBackgroundResource(drawable.icon_6);
break;
case 7:
imageView.setBackgroundResource(drawable.icon_7);
break;
case 8:
imageView.setBackgroundResource(drawable.icon_8);
break;
}
ViewGroup.LayoutParams params = new GridLayout.LayoutParams();
imageView.setLayoutParams(new LinearLayout.LayoutParams(125,125));
imageView.setClickable(true);
//为每一个imageView设置监听事件
imageView.setOnClickListener(this);
gridView.addView(imageView);
}
}
public int getRandom(){
Random random = new Random();//定义随机类
int result = random.nextInt(8);
return result+1;
}
@RequiresApi(api = Build.VERSION_CODES.JELLY_BEAN)
@Override
public void onClick(View v) {
handleImageView((ImageView)v);
}
public void handleImageView(ImageView imageView){
imageView.setImageResource(drawable.background_border);
currentImage = imageView;
}
}
2. background_border.xml
android 顶部imageview溢出
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:yarn怎么扩充资源
下一篇:vpc 容器 宿主机
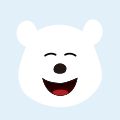
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
CSS溢出——WEB开发系列20
在网页设计中,“溢出”是一个常见且重要的概念。它涉及到如何处理那些超出预定范围的内容,以确保网页的布局和视觉效果达到预期。
html css web 前端 css3 -
java循环创建对象内存溢出怎么解决
本文简要介绍了Java循环创建对象内存溢出如何解决。
java 内存溢出 对象引用 -
android imageview形状 android imageview属性
最近在图片上犯了迷糊,在礼拜天抽出时间把属性一个一个试了一遍,在这里和大家分享一下ImageView的属性: android:src 用于显示图片 android:maxWidth="" 最大宽度 android:minHeight="" 最小高度 android
android imageview形状 imageview android xml 居中显示