using System;
namespace ConsoleApplication2
{
class Program
{
/*
public static void Main()
{
int value = -16;
Byte[] bytes = BitConverter.GetBytes(value);
for (int i = 0; i < bytes.Length; i++)
{
Console.WriteLine(bytes[i]);
}
// Convert bytes back to Int32.
int intValue = BitConverter.ToInt32(bytes, 0);
Console.WriteLine("{0} = {1}: {2}",
value, intValue,
value.Equals(intValue) ? "Round-trips" : "Does not round-trip");
// Convert bytes to UInt32.
uint uintValue = BitConverter.ToUInt32(bytes, 0);
Console.WriteLine("{0} = {1}: {2}", value, uintValue,
value.Equals(uintValue) ? "Round-trips" : "Does not round-trip");
}
*/
public static void DisplayArray(short[] arr)
{
for (int loopX = arr.Length - 1; loopX >= 0; loopX--)
Console.Write(" {0:X4}", arr[loopX]);
Console.WriteLine();
}
public static void Main()
{
// This array is to be modified and displayed.
short[] arr = { 258, 259, 260, 261, 262, 263, 264,
265, 266, 267, 268, 269, 270, 271 };
Console.WriteLine("This example of the Buffer class " +
"methods generates the following output.\n" +
"Note: The array is displayed from right to left.\n");
Console.WriteLine("Initial values of array:\n");
// Display the initial array values and ByteLength.
DisplayArray(arr);
Console.WriteLine("\nBuffer.ByteLength( arr ): {0}",
Buffer.ByteLength(arr));
// Copy a region of the array; set a byte within the array.
Console.WriteLine("\nCall these methods: \n" +
" Buffer.BlockCopy( arr, 5, arr, 16, 9 ),\n" +
" Buffer.SetByte( arr, 7, 170 ).\n");
Buffer.BlockCopy(arr, 5, arr, 16, 9);
Buffer.SetByte(arr, 7, 170);
// Display the array and a byte within the array.
Console.WriteLine("Final values of array:\n");
DisplayArray(arr);
Console.WriteLine("\nBuffer.GetByte( arr, 26 ): {0}",
Buffer.GetByte(arr, 26));
}
}
}
C# Console.Write格式化、Buffer、BitConverter用处不大
原创
©著作权归作者所有:来自51CTO博客作者RandTsui的原创作品,请联系作者获取转载授权,否则将追究法律责任
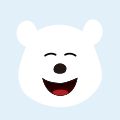
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
C# xml格式化
Collections.Generic...
C# xml格式化 属性值 xml 字符串