using System;
using System.Linq;
namespace ConsoleApplication21
{
public class Product
{
public int ProductID { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
public string Category { set; get; }
}
class Program
{
static void Main(string[] args)
{
Product[] products = {
new Product {Name = "西瓜", Category = "水果", Price = 2.3M},
new Product {Name = "苹果", Category = "水果", Price = 4.9M},
new Product {Name = "空心菜", Category = "蔬菜", Price = 2.2M},
new Product {Name = "地瓜", Category = "蔬菜", Price = 1.9M}
};
var results = from product in products
orderby product.Price descending
select new { product.Name, product.Price };
var res2 = products.Where(r=>r.Name=="地").Count();
Console.WriteLine(res2);
var res1 = products.Single(g => g.Name == "地瓜");
Console.WriteLine("商品:{0},价钱:{1}", res1.Name, res1.Price);
var res = products.Sum(p => p.Price);
/*
//打印价钱最高的三个商品
int count = 0;
foreach (var p in results)
{
Console.WriteLine("商品:{0},价钱:{1}", p.Name, p.Price);
if (++count == 3) break;
}
Console.ReadKey();
*/
}
}
}
C# 使用Linq
原创
©著作权归作者所有:来自51CTO博客作者RandTsui的原创作品,请联系作者获取转载授权,否则将追究法律责任
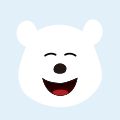
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
C# Linq Distinct的使用
,所以当我们要用某个成员属性做重复数据的判断时,就必需绕一下路,这边稍微将处理的方法做个整理并记录一下
c# Distinct 数据 泛型 ide -
C# LINQ to XML
LINQ to XML 为创建 XML 元素提供了一种称为“函数构造”的有效方式。函数构造是指在单个语句中创建 XML 树的能力。 启用
linq to xml xml xml文件 子节点 -
LINQ 查询简介 (C#)
查询是一种从数据源检索数据的表达式。 查询通常用专门的查询语言来表示。 随着时间的推移,人们已经为各
Linq C# lambader 数据源 数据 SQL -
C#遍历目录和文件(使用Linq)
语言越来越强大,程序员越来越轻松。
搜索 当前目录 模式匹配 子目录 html