package teat;
import java.util.*;
import java.util.Map.Entry;
/**
* @author linjitai
*/
public class ThreeIterator {
public static void main(String[] args) {
Map<String, String> map = new HashMap<>();
map.put("001", "宝马");
map.put("002", "奔驰");
map.put("003", "奥迪");
firstIterator(map);
System.out.println("===");
twoIterator(map);
System.out.println("===");
threeItertor(map);
}
/**
* entry
* @param map
* @return
*/
public static void firstIterator(Map<String, String> map) {
for (Entry<String, String> entry : map.entrySet()) {
String keys = entry.getKey();
String values = entry.getValue();
System.out.println("key = "+ keys + ";value = "+ values);
}
}
/**
* keySet
* @param map
*/
public static void twoIterator(Map<String, String> map) {
for (String keys : map.keySet()) {
String values = map.get(keys);
System.out.println("key = "+ keys + ";value = "+ values);
}
}
/**
*
* @param map
*/
public static void threeItertor(Map<String, String> map) {
Iterator<String> keys = map.keySet().iterator();
while (keys.hasNext()) {
String key = keys.next();
String value = map.get(key);
System.out.println("key = "+ key + ";value = "+ value);
}
}
}
/**
Output
key = 001;value = 宝马
key = 002;value = 奔驰
key = 003;value = 奥迪
===
key = 001;value = 宝马
key = 002;value = 奔驰
key = 003;value = 奥迪
===
key = 001;value = 宝马
key = 002;value = 奔驰
key = 003;value = 奥迪*/
HashMap集合的3种迭代方式
原创
©著作权归作者所有:来自51CTO博客作者wx5925899fdb5f1的原创作品,请联系作者获取转载授权,否则将追究法律责任
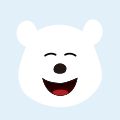
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
java遍历map集合那种方式最快
Java中遍历Map集合常用的几种方法。
System 键值对 java -
HashMap集合遍历的四种方式
对于Map来说,遍历的方式都是一样的,大概都是有四种形式直接遍历返回keySet()返回Value
HashMap 集合遍历 java 增强for循环 集合类型 -
java 遍历集合的三种方式 for循环 加强for 迭代器
在java中,有三种方式遍历集合,分别是for循环 加强for 迭代器。
java jvm 开发语言 System List -
HashMap的四种遍历方式
使用junit来测试HashMap的四种遍历方式
Java HashMap 遍历 -
HashMap 的 7 种遍历方式+性能分析!
随着 JDK 1.8 Streams API 的发布,使得 HashMap 拥有了更多的遍历的方式,但应该选择那种遍历方式?反而成了一个问题。
JDK 1.8 Streams AP HashMap 遍历方式 -
ios ui 交互卡住
近来在看<IPhone开发基础教程>,看到第一个提到交互性的例子,说是其它交互性程序的基础,故也不可小看它,按书的意思做,不懂就多看几遍. 刚开始的时候我就急着想动手写一些代码,但是效果不怎么好,其中难免会出点错.到整个讲完的时候会有不少错误发生.不过呢,没关系,我爱实践是没有错的,我
ios ui 交互卡住 iphone button hierarchy initialization