// opencv2.cpp : 定义控制台应用程序的入口点。
//
#include "stdafx.h"
#include <opencv2/opencv.hpp>
#include "opencv2/highgui/highgui.hpp"
#include "opencv2/imgproc/imgproc.hpp"
#include "opencv2/features2d/features2d.hpp"
#include "cv.h"
#include "highgui.h"
#include <cxcore.h>
using namespace cv;
using namespace std;
int main(int argc, char **argv)
{
CvPoint2D32f srcTri[4], dstTri[4];
CvMat* warp_mat = cvCreateMat(3, 3, CV_32FC1);
IplImage* src = NULL;
IplImage* dst = NULL;
src = cvLoadImage("test.jpg", 1);
dst = cvCloneImage(src);
dst->origin = src->origin;
cvZero(dst);
srcTri[0].x = 0;
srcTri[0].y = 0;
srcTri[1].x = src->width - 1;
srcTri[1].y = 0;
srcTri[2].x = 0;
srcTri[2].y = src->height - 1;
srcTri[3].x = src->width - 1;
srcTri[3].y = src->height - 1;
dstTri[0].x = src->width * 0.05;
dstTri[0].y = src->height * 0.33;
dstTri[1].x = src->width * 0.9;
dstTri[1].y = src->height * 0.25;
dstTri[2].x = src->width * 0.2;
dstTri[2].y = src->height * 0.7;
dstTri[3].x = src->width * 0.8;
dstTri[3].y = src->height * 0.9;
cvGetPerspectiveTransform(srcTri, dstTri, warp_mat);
cvWarpPerspective(src, dst, warp_mat);
cvNamedWindow("src", 1);
cvShowImage("src", src);
cvNamedWindow("Affine_Transform", 1);
cvShowImage("Affine_Transform", dst);
cvWaitKey(0);
cvReleaseImage(&src);
cvReleaseImage(&dst);
cvReleaseMat(&warp_mat);
return 0;
}
opencv之WarpPerspective透视变化
原创
©著作权归作者所有:来自51CTO博客作者303103757q的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:qt屏幕漫天雪花飘落
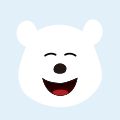
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Python OpenCV #2 - OpenCV中的GUI功能
本文介绍了OpenCV的基本方发,包括图像读取、显示和写入。
python ide OpenCV -
opencv warpPerspective 反变换 opencv hough变换
霍夫变换是图像处理中从图像中识别几何形状的基本方法之一,应用很广泛,也有很多改进算法。主要用来从图像中分离出具有某种相同特征的几何形状(如,直线,圆等)。最基本的霍夫变换是从黑白图像中检测直线(线段)。霍夫空间霍夫变换的关键是霍夫空间。  
opencv HoughLine HoughCircles 霍夫(Hough)变换 霍夫变换