/* * You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a linked list. You may assume the two numbers do not contain any leading zero, except the number 0 itself. Input: (2 -> 4 -> 3) + (5 -> 6 -> 4) Output: 7 -> 0 -> 8 具体的意思 大概就是:有一个类型 是链表类型 结构如类ListNode 把两个给出的链表的val值相加 合并成一个链表(有进位的haunted加到next链表上 比如2+9=11 也就是相加结果为1 进位为1 在下一次相加的时候要多加1 ) 如果不是很懂 就直接看代码 思路应该很清晰 */ public class Solution { /* * 方法1 */ public static ListNode addTwoNumbers(ListNode l1,ListNode l2) { //如果都为空 直接返回不为空的一个参数 如果都未空 则返回空 if(l1 == null || l2 == null){ return l1 == null ?(l2 == null ?null:l2):l1; } //返回值 ListNode ret = new ListNode(0); ListNode temp = ret; int flag = 0;//进位标示 while(true){ int sum = l1.val + l2.val+flag; //取商 进位 flag = sum/10; //取余 最后实际值(去除进位) temp.val =sum%10; // l1 l2 指向下一个节点 ret 新建下一个节点 l1 = l1.next; l2 = l2.next; //如果有一个为空就结束循环 if(l1 == null || l2 == null){ break; } temp.next = new ListNode(0); temp = temp.next; } //如果l1 l2同时遍历完(也就是同时为链尾) 查看是否最后有进位 如果有的话 需要新建节点 if(l1 == null && l2 == null){ if(flag == 1 ){ temp.next = new ListNode(flag); return ret; }else{ return ret; } }else{//两个不是同样长 其中一个遍历完 另一个还有节点没有遍历 ListNode tmpNode =l1 == null ? l2 :l1; temp.next = new ListNode(0); temp = temp.next; while(true){ int sum = tmpNode.val +flag; //取商 进位 flag = sum/10; //取余 最后实际值(去除进位) temp.val =sum%10; //下一个节点 tmpNode = tmpNode.next; if(tmpNode == null){ break; } temp.next = new ListNode(0); temp =temp.next; } } //最后的进位 if(flag == 1 ){ temp.next =new ListNode(flag); }else{ temp = null; } return ret; } /* * 方法2 */ public static ListNode addTwoNumbers2(ListNode l1, ListNode l2) { ListNode c1 = l1; ListNode c2 = l2; //返回值 ListNode ret = new ListNode(0); //d指向链表头 最后返回的时候 是ret.next 也就是从链表的第二个节点开始返回(第一个作为头 不返回) //这里其实就是一个小技巧了 可以看方法一 赋值都是从第一个节点开始的 而方法二赋值都是从next开始的( d.next) //从next开始赋值 就可以把代码统一一下 赋值都是: d.next = new ListNode(sum % 10); //如果不是从第二个节点开始 第一个赋值要:temp.val =sum%10; 第二个赋值要temp = temp.next //这样说没什么感觉 亲自动手写一写 你就明白了 ListNode d = ret; int sum = 0; while (c1 != null || c2 != null) { sum /= 10; //加和 指向下一个节点 if (c1 != null) { sum += c1.val; c1 = c1.next; } if (c2 != null) { sum += c2.val; c2 = c2.next; } //设置下一个节点赋值(从第二个开始 第一个就当做头结点了) d.next = new ListNode(sum % 10); d = d.next; } //最后是否有进位 如果有添加节点 如果没有就直接返回 if (sum / 10 == 1) d.next = new ListNode(1); // return ret.next; } public static void main(String[] args) { ListNode l1 = new ListNode(5); // l1.next = new ListNode(4); // l1.next.next = new ListNode(3); // l1.next.next.next = new ListNode(7); ListNode l2 = new ListNode(5); // l2.next = new ListNode(6); // l2.next.next = new ListNode(4); ListNode ret= Solution.addTwoNumbers(l1,l2); System.out.println(ret.toString()); } } /* * 链表实体类 */ class ListNode { int val; ListNode next; ListNode(int x) { val = x; } @Override public String toString() { String ret = val+""; while(next != null){ ret=ret+"---"+next.val; next = next.next; } return ret; } }
leetcode Add Two Numbers 解决思路
原创
©著作权归作者所有:来自51CTO博客作者木子的昼夜的原创作品,请联系作者获取转载授权,否则将追究法律责任
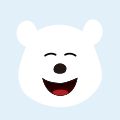
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
GitHub two-factor authentication开启教程
GitHub two-factor authentication开启教程
GitHub 2FA 登录验证 two-factor -
【LeetCode】39. 组合总和
【LeetCode】39. 组合总和
leetcode 数据结构与算法 C++ -
【LeetCode】705. 设计哈希集合
【LeetCode】705. 设计哈希集合
leetcode 数据结构与算法 C++ -
[LeetCode]Add Two Numbers
第二题
边界条件 git 流程图 -
Leetcode: Add Two Numbers II
The key of this problem is to think of using Stack
Leetcode Stack Linkedlist git 编程题目 -
重新启动resourcmanager
1、Windows Mobile手机的记忆体容量概念 与电脑相仿,Windows Mobile手机自身的记忆体从用途可以分为“程序运行空间”和“储存空间”。“程序运行空间”主要是运行程序使用;“储存空间”主要用来安装程序,保存文件档案等。由于Windows mobile手机自身的“储存空间”不大,一般不会超过2
重新启动resourcmanager 操作系统 Windows Mobile 程序运行