标签类:
package cn.bugu_tag.page;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.tagext.TagSupport;/**
*
* @author Xiaoping Huang (hxpwangyi@163.com)
*/
public class BuguPageTag extends TagSupport {
private int pageNum = 1;
private int pages;
private String url;@Override
public int doStartTag() throws JspException {HttpServletRequest request = (HttpServletRequest) pageContext.getRequest();
request.setAttribute("pageNum", pageNum);
request.setAttribute("pages", pages);
request.setAttribute("url", url);
try {
pageContext.include("/WEB-INF/template/page.jsp");
} catch (ServletException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return SKIP_BODY;
} public String getUrl() {
return url;
}public void setUrl(String url) {
this.url = url;
}public int getPageNum() {
return pageNum;
} public void setPageNum(int pageNum) {
this.pageNum = pageNum;
} public int getPages() {
return pages;
} public void setPages(int pages) {
this.pages = pages;
}}
jsp页面:
<%@ page language="java" pageEncoding="UTF-8"%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<style>
@charset utf-8;
.pagination{height:30px;vertical-align:middle;margin:5px 10px 0;}
.pagination a{display:inline-block;margin-right:5px;width:25px;height:25px;line-height:25px;border:1px solid #ccc;text-align:center;text-decoration:none;color:#555;font-size:14px;overflow:hidden}
.pagination a:hover{background-color:#FFEDE1;text-decoration:none;font-weight:700;border-color:#FD6D01}
.pagination .current{display:inline-block;margin-right:5px;width:25px;height:25px;line-height:25px;border:1px solid #FD6D01;text-align:center;color:#555;font-size:14px;overflow:hidden;background-color:#FFEDE1;font-weight:700}
.pagination a.pagenum{width:52px;height:25px}
.pagination span{margin-right:5px;width:25px;height:25px;line-height:25px;display:inline-block;font-family:Tahoma,SimSun,Arial;font-size:12px;text-align:center;vertical-align:top;}
.pagination .prev,.pagination .next{display:inline-block;margin-right:5px;width:50px;height:25px;line-height:25px;border:1px solid #ccc;text-align:center;color:#999;font-size:14px;overflow:hidden}
.pagination .pagejump{width:auto;color:#666;vertical-align:top;padding:0 5px;}
.pagination .pagejump input{width:30px;height:20px;text-align:center;font-family:Tahoma,SimSun,Arial;color:#000;border:1px solid #999;margin:0 5px}
.pagination .pagejump button{width:50px;height:25px;cursor:pointer;border:1px solid #ccc;margin:0 0 0 5px}
</style>
<script type="text/javascript" src="/bugu_ui/jquery/jquery.min.js"></script>
<script type="text/javascript">
function beforeSubmit(){
var re = /^[1-9][0-9]*$/;
if(!re.test($("#pageNum1").val())){
$("#pageNum1").val(1);
}
if($("#pageNum1").val()>${pages}){
$("#pageNum1").val(${pages});
}
return true;
}
</script>
<c:if test="${pages>0}">
<div id="pagination" class="pagination">
<form action="${url}" method="post" onSubmit="beforeSubmit()">
<c:if test="${fn:indexOf(url,'?')==-1}">
<c:choose>
<c:when test="${pageNum==1}">
<span class="prev">首页</span>
<span class="prev">上一页</span>
</c:when>
<c:otherwise>
<a href="${url}?pageNum=1" class="pagenum" >首页</a>
<a href="${url}?pageNum=${pageNum-1}" class="pagenum" >上一页</a>
</c:otherwise>
</c:choose>
<c:if test="${pageNum-3>0}">
<a href="${url}?pageNum=${pageNum-3}" >${pageNum-3}</a>
</c:if>
<c:if test="${pageNum-2>0}">
<a href="${url}?pageNum=${pageNum-2}" >${pageNum-2}</a>
</c:if>
<c:if test="${pageNum-1>0}">
<a href="${url}?pageNum=${pageNum-1}" >${pageNum-1}</a>
</c:if>
<span class="current">${pageNum}</span>
<c:if test="${pageNum+1<=pages}">
<a href="${url}?pageNum=${pageNum+1}" >${pageNum+1}</a>
</c:if>
<c:if test="${pageNum+2<=pages}">
<a href="${url}?pageNum=${pageNum+2}" >${pageNum+2}</a>
</c:if>
<c:if test="${pageNum+3<=pages}">
<a href="${url}?pageNum=${pageNum+3}" >${pageNum+3}</a>
</c:if>
<c:choose>
<c:when test="${pageNum==pages}">
<span class="next">下一页</span>
<span class="next">末页</span>
</c:when>
<c:otherwise>
<a href="${url}?pageNum=${pageNum+1}" class="pagenum">下一页</a>
<a href="${url}?pageNum=${pages}" class="pagenum" >末页</a>
</c:otherwise>
</c:choose>
<span class="pagejump">共${count}条 跳到第<input type="text" value="${pageNum}" size="3" name="pageNum" id="pageNum1">页<button type="submit">确定</button></span>
</c:if>
<c:if test="${fn:indexOf(url,'?')!=-1}">
<c:choose>
<c:when test="${pageNum==1}">
<span class="prev">首页</span>
<span class="prev">上一页</span>
</c:when>
<c:otherwise>
<a href="${url}&pageNum=1" class="pagenum" >首页</a>
<a href="${url}&pageNum=${pageNum-1}" class="pagenum" >上一页</a>
</c:otherwise>
</c:choose>
<c:if test="${pageNum-3>0}">
<a href="${url}&pageNum=${pageNum-3}" >${pageNum-3}</a>
</c:if>
<c:if test="${pageNum-2>0}">
<a href="${url}&pageNum=${pageNum-2}" >${pageNum-2}</a>
</c:if>
<c:if test="${pageNum-1>0}">
<a href="${url}&pageNum=${pageNum-1}" >${pageNum-1}</a>
</c:if>
<span class="current">${pageNum}</span>
<c:if test="${pageNum+1<=pages}">
<a href="${url}&pageNum=${pageNum+1}" >${pageNum+1}</a>
</c:if>
<c:if test="${pageNum+2<=pages}">
<a href="${url}&pageNum=${pageNum+2}" >${pageNum+2}</a>
</c:if>
<c:if test="${pageNum+3<=pages}">
<a href="${url}&pageNum=${pageNum+3}" >${pageNum+3}</a>
</c:if>
<c:choose>
<c:when test="${pageNum==pages}">
<span class="next">下一页</span>
<span class="next">末页</span>
</c:when>
<c:otherwise>
<a href="${url}&pageNum=${pageNum+1}" class="pagenum">下一页</a>
<a href="${url}&pageNum=${pages}" class="pagenum" >末页</a>
</c:otherwise>
</c:choose>
<span class="pagejump">共${count}条 跳到第<input type="text" value="${pageNum}" size="3" name="pageNum" id="pageNum1">页<button type="submit">确定</button></span>
</c:if>
</form>
</div>
</c:if>
<c:if test="${pages==0}">
没有数据
</c:if>tld文件
<?xml version="1.0" encoding="utf-8"?>
<taglib xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee web-jsptaglibrary_2_0.xsd"
version="2.0">
<tlib-version>1.0</tlib-version>
<short-name>pageTag</short-name>
<!-- 定义该标签库的URI -->
<uri>http://www.bugu_tag.cn/page</uri>
<!-- 定义第一个标签 -->
<tag>
<!-- 定义标签名 -->
<name>page</name>
<!-- 定义标签处理类 -->
<tag-class>cn.bugu_tag.page.BuguPageTag</tag-class>
<!-- 定义标签体为空 -->
<body-content>jsp</body-content>
<attribute>
<name>url</name>
<required>false</required>
<rtexprvalue>true</rtexprvalue>
</attribute>
<attribute>
<name>pageNum</name>
<required>false</required>
<rtexprvalue>true</rtexprvalue>
</attribute>
<attribute>
<name>pages</name>
<required>false</required>
<rtexprvalue>true</rtexprvalue>
</attribute>
</tag>
</taglib>
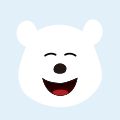
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
JSTL 标签库
【代码】JSTL 标签库。
java 前端 servlet 标签库 属性设置 -
JSTL标签引入
ticle/details/3960973
apache struts java -
java jstl c if标签 jstl c:if
使用jstl标签中的c:if标签进行字符串比较时,可以使用eq/ne进行比较。(not eq/!eq会报错)
java jstl c if标签 jstl c:if 字符串比较 jstl标签 -
jstl标签经典
1.<c:out>库:Core(核心库)URI:http://java.sun.com/jsp/jstl/core前缀:c描述
java 迭代 默认值 html 作用域