package util; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.support.ui.ExpectedCondition; import org.openqa.selenium.support.ui.WebDriverWait; /** * * @author yedeng *等待时间的工具类 */ public class WaitTime { /** * 通过页面元素来判定等待时间 * @param driver * @param element * @param timeOut */ public static void waitForLoad(WebDriver driver,final WebElement element,int timeOut){ WebDriverWait wait=new WebDriverWait(driver, timeOut); wait.until(new ExpectedCondition<Boolean>() { public Boolean apply() { boolean loadcomplete = element.isDisplayed(); return loadcomplete; } @Override public Boolean apply(WebDriver arg0) { // TODO Auto-generated method stub return null; } }); } /** * 通过By定位元素来定义等待时间 * @param driver * @param by * @param timeOut */ public static void waitForLoad(WebDriver driver,final By by,int timeOut){ WebDriverWait wait=new WebDriverWait(driver, timeOut); wait.until(new ExpectedCondition<Boolean>() { @Override public Boolean apply(WebDriver d) { boolean loadcomplete = d.findElement(by).isDisplayed(); return loadcomplete; } }); } }
webdriver 等待时间工具类
原创
©著作权归作者所有:来自51CTO博客作者yunjians的原创作品,请联系作者获取转载授权,否则将追究法律责任
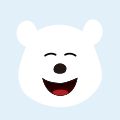
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章