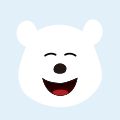
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
使用ref和$refs获取dom和组件
使用ref和$refs获取dom,获取组件
前端 javascript 开发语言 获取数据 App -
【React】243- 在 React 组件中使用 Refs 指南
通过例子来了解
输入框 html 构造函数 -
在 React 组件中使用 Refs 指南
我什么时候应该使用 Refs ? 我们建议在以下情况下使用 refs: 与第三方 DOM 库集成 触发命令式动画 管理焦点,文本选
输入框 html 构造函数 赋值 参数传递 -
react js 组件传参(转发)
assed into child components from parents (if we think
React.js sed html ide javascript -
react refs All In One
react refs All In One
refs React refs React js html