package mztest;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStreamReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import com.kingdee.bos.BOSException;
import com.kingdee.eas.common.EASBizException;
import com.kingdee.eas.fm.common.FMIsqlFacadeFactory;
import com.kingdee.eas.fm.common.IFMIsqlFacade;
import edu.emory.mathcs.backport.java.util.Collections;
public class mz {
private static final String DRIVER_CLASS = "oracle.jdbc.driver.OracleDriver";
private static String DATABASE_URL = "jdbc:oracle:thin:@192.168.0.8:1521:eas";;
private static String DATABASE_USER = "*********";
private static String DATABASE_PASSWORD = "***********";
// private static String DATABASE_URL = "jdbc:oracle:thin:@10.123.69.161:1521:KDDB1";;
// private static String DATABASE_USER = "kingdee";
// private static String DATABASE_PASSWORD = "kingdee";
public static Connection getConn() {
try {
Class.forName(DRIVER_CLASS);
Connection dbConnection = DriverManager.getConnection(
DATABASE_URL, DATABASE_USER, DATABASE_PASSWORD);
return dbConnection;
} catch (SQLException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return null;
}
public static void closeAll(Connection conn, PreparedStatement pstmt,
ResultSet rs) {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null)
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void main(String[] args) throws FileNotFoundException, SQLException {
String materialTypeNumber="08.00";
ArrayList numberList= new ArrayList();
ArrayList numberstrList= new ArrayList();
Connection conn = null;
PreparedStatement ps = null;
conn = getConn();
String sql=" select FNUMBER from T_BD_MATERIAL where FMATERIALGROUPID= ( select fid from T_BD_MATERIALGROUP where FNUMBER='"+materialTypeNumber+"' ) order by FNUMBER ";
ps=conn.prepareStatement(sql);
ResultSet executeSQL = ps.executeQuery();
String number="";
while (executeSQL.next() ) {
number= executeSQL.getString(1);
int lastIndexOf = number.lastIndexOf(".");
String strc= number.substring(lastIndexOf+1,number.length() ) ;
Integer c= Integer.valueOf(number.substring(lastIndexOf+1,number.length() ) );
numberList.add(c);
numberstrList.add(strc);
}
if(numberList.size()<=0){
numberList.add(1);
numberstrList.add("001");
}
Collections.sort(numberList);
String bigNumber=numberList.get(numberList.size() - 1).toString();
System.out.println("输出numberList================================");
System.out.println(numberList);
String c=materialTypeNumber+".";
String augend= getMaxLength( numberstrList );
//被加数前面有0,前面的0会消失, 进行补0
String format = String.format("%0"+augend.length()+"d", Long.parseLong(bigNumber)+1);
number=c+format;
System.out.println("输出================================");
System.out.println(number);
}
public static String getMaxLength(ArrayList numberstrList ){
int maxLengthIndex=0;
for (int i = 0; i < numberstrList.size(); i++) {
if(numberstrList.get(i).toString().length()>numberstrList.get(maxLengthIndex).toString().length()){
maxLengthIndex=i;
}
}
return numberstrList.get(maxLengthIndex).toString();
}
}
客户物料编码获取测试
原创
©著作权归作者所有:来自51CTO博客作者晴天MZ的原创作品,请联系作者获取转载授权,否则将追究法律责任
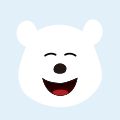
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
JS选择图片获取base64编码预览图片
通过将图片转为data url的base64格式编码,实现直接预览图片
图片预览 base64 dataurl 图片转base64 -
浅谈ERP系统中的物料编码
物料编码-企业信息化的源头
物料编码 如何用勤哲服务器来进行编码管理 企业信息化的源头 -
获取物料主数据的特性值
获取物料主数据的特性值
字段 码农 微信