//////////////////////////////////////////////////////////////////////// /////////////////////泛型编程之动态顺序表的模板///////////////////////// //////////////////////////////////////////////////////////////////////// #include<iostream> #include<string> using namespace std; template<typename Type> //建立动态顺序表类模板 class Vector { public: Vector() :_head(NULL), _size(0),_capacity(0) {} Vector(const Vector<Type>& vector) :_head(NULL), _size(0), _capacity(0) { _head = new Type[vector._size]; //memcpy(_head, vector._head, sizeof(Type)*vector._size); (错误的写法) //当Type 是自定义类型时,可能会造成的浅拷贝,从而造成程序崩溃 for (int i = 0; i < vector._size; i++) { _head[i] = vector._head[i]; } _size = vector._size; _capacity = vector._capacity; } Vector<Type>& operator = (const Vector<Type>& vector) { if (this != &vector) { if (_head != NULL) { delete[] _head; _size = 0; _capacity = 0; } _head = new Type[vector._size]; for (int i = 0; i < vector._size; i++) { _head[i] = vector._head[i]; } _size = vector._size; _capacity = vector._capacity; } return *this; } ~Vector() { if (_head != NULL) { delete[] _head; } _size = 0; _capacity = 0; } public: void PushBack(Type x) { _CheckCapacity(); _head[_size++] = x; } void Print() { cout << "顺序表:" << endl; for (int i = 0; i < _size; i++) { cout << _head[i] << " "; } cout << endl << endl; } protected: void _CheckCapacity() { if (_size >= _capacity) { Type *tmp = _head; _head = new Type[2 * _capacity + 3]; //memcpy(_head, vector._head, sizeof(Type)*vector._size); (错误的写法) //当Type 是自定义类型时,可能会造成的浅拷贝,从而造成野指针问题,析构时就会出现问题 for (int i = 0; i < _size; i++) { _head[i] = tmp[i]; } delete[] tmp; _capacity = 2 * _capacity + 3; } } protected: Type *_head; size_t _size; size_t _capacity; }; void Test_string() { Vector<string> vector; vector.PushBack("aaaaa"); vector.PushBack("bbbbb"); vector.PushBack("ccccc"); vector.PushBack("ddddd"); vector.PushBack("eeeee"); Vector<string> vector_2(vector); Vector<string> vector_3; vector_3 = vector_2; vector_3.Print(); } void Test_double() { Vector<double> vector; vector.PushBack(1.1); vector.PushBack(2.2); vector.PushBack(3.3); vector.PushBack(4.4); vector.PushBack(5.5); Vector<double> vector_2(vector); Vector<double> vector_3; vector_3 = vector_2; vector_3.Print(); } void Test_char() { Vector<char> vector; vector.PushBack('a'); vector.PushBack('b'); vector.PushBack('c'); vector.PushBack('d'); vector.PushBack('e'); Vector<char> vector_2(vector); Vector<char> vector_3; vector_3 = vector_2; vector_3.Print(); } void main() { Test_string(); Test_double(); Test_char(); } ////////////////////////////////////////////////////////////////////////// ///////////////////////泛型编程之动态双向链表的模板/////////////////////// ////////////////////////////////////////////////////////////////////////// #include<iostream> #include<string> using namespace std; template<typename Type> struct ListNode { ListNode(Type x = 0) :_date(x), _next(NULL), _prev(NULL) { } Type _date; ListNode<Type> *_next; ListNode<Type> *_prev; }; template<typename Type> class List { public: List() :_head(NULL), _tail(NULL) {} List(const List<Type>& list) :_head(NULL), _tail(NULL) { ListNode<Type> *tmp = list._head; while (tmp) { PushBack(tmp->_date); tmp = tmp->_next; } } List<Type> & operator = (List<Type> list) { if (this != &list) { swap(_head, list._head); swap(_tail, list._tail); } return *this; } ~List() { if (_head != NULL) { while (_head) { ListNode<Type> *tmp = _head; _head = _head->_next; delete tmp; } } _head = NULL; _tail = NULL; } public: void PushBack(Type x) { ListNode<Type> *tmp = new ListNode<Type>(x); if (_head == NULL) { _head = _tail = tmp; } else { _tail->_next = tmp; tmp->_prev = _tail; _tail = _tail->_next; } } void Print() { cout << "单链表为:" << endl; ListNode<Type> *tmp = _head; while (tmp) { cout << tmp->_date << "->"; tmp = tmp->_next; } cout << "NULL\n" <<endl; } protected: ListNode<Type> *_head; ListNode<Type> *_tail; }; void Test_int() { List<int> list; list.PushBack(1); list.PushBack(2); list.PushBack(3); list.PushBack(4); list.PushBack(5); list.PushBack(6); list.Print(); } void Test_char() { List<char> list; list.PushBack('a'); list.PushBack('b'); list.PushBack('c'); list.PushBack('d'); list.PushBack('e'); list.PushBack('f'); list.Print(); } void Test_string() { List<string> list; list.PushBack("aaaaa"); list.PushBack("bbbbb"); list.PushBack("ccccc"); list.PushBack("dddddd"); list.PushBack("eeeeee); list.PushBack("ffffff"); list.Print(); } void Test_1() { List<string> list; list.PushBack("aaaaa"); list.PushBack("bbbbbb"); list.PushBack("cccccc"); List<string> list_2(list); List<string> list_3; list_3 = list_2; list.Print(); list_2.Print(); list_3.Print(); } void main() { Test_int(); Test_char(); Test_string(); Test_1(); }
动态顺序表 与 双向链表的模板类
原创icandothis 博主文章分类:c++ ©著作权
文章标签 动态顺序表 双向链表 & 文章分类 数据结构与算法 人工智能
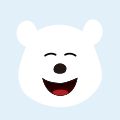
-
顺序表的动态分配(C语言版)
顺序表的动态分配代码实录
顺序表 动态分配 C语言 -
1.顺序表的实现——C语言
顺序表各种操作的代码实现
顺序表 冒泡排序 算法 随机数 -
线性表--顺序表--双向链表(六)
双向链表也叫双链表,是链表的一种,它的每个数据结点中都有两个指针,分别指向直接后继和直接
数据结构 链表 指针 c语言 结点