package com.teny.utils.p_w_picpath; import java.awt.Color; import java.awt.Font; import java.awt.Graphics2D; import java.awt.RenderingHints; import java.awt.p_w_picpath.BufferedImage; import java.io.File; import java.io.FileOutputStream; import javax.servlet.http.HttpServletRequest; import org.apache.commons.lang3.StringUtils; import org.apache.struts2.ServletActionContext; import com.sun.p_w_picpath.codec.jpeg.JPEGCodec; import com.sun.p_w_picpath.codec.jpeg.JPEGEncodeParam; import com.sun.p_w_picpath.codec.jpeg.JPEGImageEncoder; import com.teny.utils.string.Constants; /** * 功能描述:根据提供的文字生成jpg图片 * * @author : Teny_lu 刘鹰 * @ProjectName : b2b_new * @FileName : CreateImageUtils.java * @E_Mail : liuying5590@163.com * @CreatedTime : 2015年2月10日-上午11:24:55 */ public class CreateImageUtils { /** * 功能描述:根据提供的文字生成jpg图片 * * @author : Teny_lu 刘鹰 * @E_Mail : liuying5590@163.com * @CreatedTime : 2015年2月10日-上午10:19:50 * @param text * String 文字 * @param high * int 每个字的宽度和高度是一样的 * @param bgcolor * Color 背景色 * @param fontcolor * Color 字色 * @param fontPath * String 字体文件 * @param jpgname * String jpg图片名 * @return */ private String createJpgByFont(String text, int high, Color bgcolor, Color fontcolor, String fontPath, String jpgname) { try { // 宽度 高度 int width = text.length() * high; BufferedImage bp_w_picpath = new BufferedImage(width / 2, high, BufferedImage.TYPE_INT_RGB); Graphics2D g = bp_w_picpath.createGraphics(); g.setColor(bgcolor); // 背景色 g.fillRect(0, 0, width, high); // 画一个矩形 System.out.println("文本长度:" + text.length() + " ,高度:" + high); g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); // 去除锯齿(当设置的字体过大的时候,会出现锯齿) g.setColor(fontcolor); // 字的颜色 File file = new File(fontPath); // 字体文件 Font font = Font.createFont(Font.TRUETYPE_FONT, file); // 根据字体文件所在位置,创建新的字体对象(此语句在jdk1.5以上才支持) // g.setFont(font.deriveFont((float) smallWidth)); // font.deriveFont(float f)复制当前 Font 对象并应用新设置字体的大小 g.setFont(new Font("Times New Roman", Font.PLAIN, Integer.valueOf(StringUtils.substringBeforeLast(String.valueOf(high * 0.66), ".")))); int sort = text.length(); if (sort >= 1 && sort <= 9) { g.drawString(text, Integer.valueOf(StringUtils.substringBeforeLast(String.valueOf(high * 0.1 * sort), ".")), Integer.valueOf(StringUtils.substringBeforeLast(String.valueOf(high * 0.75), ".")));// 控制文字的坐标 (文字,x轴,y轴) } else if (text.length() > 9 && text.length() < 16) { g.drawString(text, high, Integer.valueOf(StringUtils.substringBeforeLast(String.valueOf(high * 0.75), ".")));// 控制文字的坐标 (文字,x轴,y轴) } else { g.drawString(text, Integer.valueOf(StringUtils.substringBeforeLast(String.valueOf(high * 1.2), ".")), Integer.valueOf(StringUtils.substringBeforeLast(String.valueOf(high * 0.75), ".")));// 控制文字的坐标 (文字,x轴,y轴 } // 在指定坐标除添加文字 g.dispose(); FileOutputStream out = new FileOutputStream(jpgname); // 指定输出文件 JPEGImageEncoder encoder = JPEGCodec.createJPEGEncoder(out); JPEGEncodeParam param = encoder.getDefaultJPEGEncodeParam(bp_w_picpath); param.setQuality(50f, true); encoder.encode(bp_w_picpath, param); // 存盘 out.flush(); out.close(); } catch (Exception e) { System.out.println("创建文字图片jpg失败!"); e.printStackTrace(); } return "made in Teny_Lu"; } public String getRandomNumber(Integer val) {// 取随机数字符串 double num = 0; switch (val) { case 1: num = Math.random() * 10; break; case 2: num = Math.random() * 100; break; case 3: num = Math.random() * 1000; break; case 4: num = Math.random() * 10000; break; case 5: num = Math.random() * 100000; break; case 6: num = Math.random() * 1000000; break; case 7: num = Math.random() * 10000000; break; case 8: num = Math.random() * 100000000; break; case 9: num = Math.random() * 1000000000; break; } long t = Math.round(num); String st = new Long(t).toString(); return st; } protected HttpServletRequest getRequest() { return ServletActionContext.getRequest(); } /** * 功能描述:创建一张数字图片(固定文件为:.ttf后缀的文字说明) * * @author : Teny_lu 刘鹰 * @E_Mail : liuying5590@163.com * @CreatedTime : 2015年2月10日-上午11:38:04 * @param val * 几位数(1-9) * @param bgColor * 背景色 * @param textColor * 文字颜色 * @return * @return */ public static String create(Integer val, Color bgColor, Color textColor) { CreateImageUtils tt = new CreateImageUtils(); String num = tt.getRandomNumber(val); tt.createJpgByFont(num, 120, bgColor, textColor, "D:/times.ttf", "D:/" + Constants.getSimpleDateFormat2() + "_" + num + ".jpg"); return num; } public static String create(String text, Color bgColor, Color textColor) { CreateImageUtils tt = new CreateImageUtils(); tt.createJpgByFont(text, 120, bgColor, textColor, "D:/times.ttf", "D:/" + Constants.getSimpleDateFormat2() + "_" + text + ".jpg"); return text; } /** * @author : Teny_lu 刘鹰 * @E_Mail : liuying5590@163.com * @CreatedTime : 2015年2月10日-下午2:58:39 * @param val * 几位数(1-9) * @param bgColor * 背景色 * @param textColor * 文字颜色 * @param high * 高度 * @return * @return */ public static String create(Integer val, Color bgColor, Color textColor, int high) { CreateImageUtils tt = new CreateImageUtils(); String num = tt.getRandomNumber(val); tt.createJpgByFont(num, high, bgColor, textColor, "D:/times.ttf", "D:/" + Constants.getSimpleDateFormat2() + "_" + num + ".jpg"); return num; } public static String create(String text, Color bgColor, Color textColor, int high) { CreateImageUtils tt = new CreateImageUtils(); tt.createJpgByFont(text, high, bgColor, textColor, "D:/times.ttf", "D:/" + Constants.getSimpleDateFormat2() + "_" + text + ".jpg"); return text; } }
二选一模式:根据提供的文字或数值生成jpg图片
精选 原创
©著作权归作者所有:来自51CTO博客作者a7272706的原创作品,请联系作者获取转载授权,否则将追究法律责任
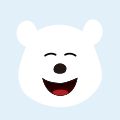
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
根据Excel生成Insert或Update语句
根据Excel生成SQL
EXCEL SQL -
java根据图片和文字生成自定义图片
java根据图片和文字生成自定义图片
java 2d 字体颜色 提示框 生成图片