1.创建项目
spring092601
2.引入spring jar包
commons-logging.jar
junit-4.4.jar
log4j.jar
spring-beans-3.2.0.RELEASE.jar
spring-context-3.2.0.RELEASE.jar
spring-core-3.2.0.RELEASE.jar
spring-expression-3.2.0.RELEASE.jar
3.添加配置文件
在conf下添加spring的核心配置文件applicationContext.xml,配置如下:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd">
</beans>
4.创建业务bean
1).在src目录下创建业务Bean
包名:cn.jbit.spring092601.domain
package cn.jbit.spring092601.domain;
import java.io.Serializable;
/**
* 教师类
* @author Administrator
*
*/
public class Teacher implements Serializable {
private String name;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
2).在src目录下创建工厂Bean
包名:cn.jbit.spring092601.factory
package cn.jbit.spring092601.factory;
import cn.jbit.spring092601.domain.Teacher;
/**
* 教师工厂类
* @author Administrator
*
*/
public class TeacherFactory {
/**
* 获得教师对象
* @return 教师对象
*/
public Teacher getTeacher(){
return new Teacher();
}
}
5.在配置文件中编写bean的配置,并注入属性值。
配置如下:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd">
<!--
实现工厂方法方式装配bean
-->
<!-- 教师工厂Bean -->
<bean id="teacherFactory" class="cn.jbit.spring092601.factory.TeacherFactory"></bean>
<!-- 教师bean -->
<bean id="teacher" factory-bean="teacherFactory" factory-method="getTeacher">
<property name="name">
<value>邓</value>
</property>
</bean>
</beans>
6.测试bean的属性注入
在test目录下测试
包名:cn.jbit.spring092601.domain
public class HelloSpringTest {
/**
* 实现工厂方法方式装配Bean
*/
@Test
public void testFactory2(){
/*
*
* 对象由spring创建,调用工厂中的静态方法来创建Bean
*/
BeanFactory beanFactory = new XmlBeanFactory(new ClassPathResource("applicationContext.xml"));
Teacher teacher = (Teacher) beanFactory.getBean("teacher");
//teacher.setName("ff");
System.out.println(teacher.getName());
}
}
Spring-装配Bean的3种方式-实现工厂方法方式装配Bean
原创
©著作权归作者所有:来自51CTO博客作者素颜猪的原创作品,请联系作者获取转载授权,否则将追究法律责任
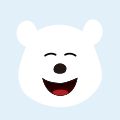
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章