/************************************ WZ ASUST 2016 模板实现单向链表 ************************************/ #include"sts.h" template <class T> struct node { public:node(const T &d):next(NULL),data(d){} T data; node<T> *next; }; template <class T> class dlist { private: node<T>* head;node<T>* tail; public: dlist():head(NULL),tail(NULL){}; ~dlist(){node<T>*cur=head;while(cur){node<T>*del=cur;cur=cur->next;delete del;}} void print(){node<T>*cur=head;while(cur){cout<<cur->data<< " ";cur=cur->next;}cout<<"ok"<<endl;} dlist & operator=(const dlist <T> & dl) { if(this!=&dl) { head=dl.head; tail=dl.tail; node<T>*dlcur=dl.head; node<T>*cur=dlcur; int xx=length()-1; while(xx--) { dlcur=dlcur->next; dlcur=cur; cur=cur->next; } } } int length() { int ret=0; if(head) { node<T>*cur=head; while(cur) { ret++; cur=cur->next; } return ret; } else return 0; } void pushback(const T &d) { if(head==NULL) { head=new node<T>(d); tail=head; } else { tail->next=new node<T>(d); tail=tail->next; } } T popback() { if(head==NULL) { return 0; } else { return tail->data; } } void pushfront(const T &d) { if(head==NULL) { head=new node<T>(d); tail=head; } else { node<T> *add=new node<T>(d); add->next=head; head=add; } } T popfront() { if(head==NULL) { return 0; } else { return head->data; } } void insert(int x, const T d) { if(x<0||x<length()) {x=x-1; node<T> *add=new node<T>(d); node<T> *cur=head; if(head) { while(x--) {cur=cur->next;} add->next=cur->next; cur->next=add; } } } void reverse() { int len=length(); node<T> *tmp=NULL; if(head) { node<T>* p = head; node<T>* q = head; node<T>* th=NULL; while(len--) { q=p; p=p->next; q->next=th; th=q; } head=th; } else return; } void paixu() { int len=length(); int state=1; T tmp; //len>1; while(len--&&state) { node<T>* p = head; node<T>* q=p->next; while(q) { if(p->data >= q->data){ tmp=q->data;q->data=p->data;p->data=tmp; } else state=1; p=p->next; q=q->next; } } } }; void test() { dlist<int> int_dlist; cout<<"** 1 : ***************"<<endl; int_dlist.pushback(11); int_dlist.pushback(22); int_dlist.pushback(33); int_dlist.pushback(44); int_dlist.print(); cout<<"*** 2 :**************"<<endl; int_dlist.pushfront(55); int_dlist.pushfront(66); int_dlist.pushfront(77); int_dlist.print(); cout<<"*** 3 :**************"<<endl; cout<<int_dlist.popfront()<<endl; cout<<int_dlist.popback()<<endl; cout<<"*** 4 :**************"<<endl; dlist<int> int_dlist2; int_dlist2=int_dlist; int_dlist.print(); cout<<"** 5 : ***************"<<endl; cout<<int_dlist.length()<<endl; } int main() { // test(); dlist<int> int_dlist; int_dlist.pushback(11); int_dlist.pushback(22); int_dlist.pushback(44); int_dlist.pushback(33); int_dlist.print(); cout<<"** 6 : ***************"<<endl; int_dlist.reverse(); int_dlist.print(); cout<<"** 7 : ***************"<<endl; int_dlist.insert(3,99); int_dlist.paixu(); int_dlist.print(); return 0; }
模板实现单向链表
原创
©著作权归作者所有:来自51CTO博客作者文洲的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:c++ 模板实现顺序表
下一篇: 模板实现双向链表
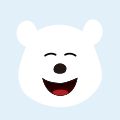
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
简单的尾插法实现单链表
尾插法建立单链表
结点 头结点 链表 -
4.带头节点的双链表的实现(C语言)
双链表基本运算的代码实现,前插、后插操作
双链表 头结点 插入操作 删除操作 -
Python实现单向链表
Python实现单向链表关于链表的介绍,请参
Python实现单向链表 单向链表