package com.extop.appday;
import android.content.Context;
import android.util.Log;
import android.widget.Toast;
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.OutputStream;
import java.io.InputStream;
import java.util.Map;
import java.io.IOException;
import java.net.URLEncoder;
import java.io.ByteArrayOutputStream;
public class HttpUtils {
public static String post(String httpUrl, String strData)
{
try{
byte[] data = strData.getBytes();//获得请求体
URL url = new URL(httpUrl);
HttpURLConnection httpURLConnection = (HttpURLConnection)url.openConnection();
httpURLConnection.setConnectTimeout(5000); //设置连接超时时间
httpURLConnection.setDoInput(true); //打开输入流,以便从服务器获取数据
httpURLConnection.setDoOutput(true); //打开输出流,以便向服务器提交数据
httpURLConnection.setRequestMethod("POST"); //设置以Post方式提交数据
httpURLConnection.setUseCaches(false); //使用Post方式不能使用缓存
//设置请求体的类型是文本类型
httpURLConnection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
//设置请求体的长度
httpURLConnection.setRequestProperty("Content-Length", String.valueOf(data.length));
//获得输出流,向服务器写入数据
OutputStream outputStream = httpURLConnection.getOutputStream();
outputStream.write(data);
int response = httpURLConnection.getResponseCode(); //获得服务器的响应码
if(response == HttpURLConnection.HTTP_OK) {
InputStream inptStream = httpURLConnection.getInputStream();
return dealResponseResult(inptStream); //处理服务器的响应结果
}
}
catch (Exception ex)
{
Log.d("AppDay", ex.toString());
}
return "ok";
}
public static String dealResponseResult(InputStream inputStream) {
String resultData = null; //存储处理结果
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
byte[] data = new byte[1024];
int len = 0;
try {
while((len = inputStream.read(data)) != -1) {
byteArrayOutputStream.write(data, 0, len);
}
} catch (IOException e) {
// e.printStackTrace();
Log.d("AppDay", e.toString());
}
resultData = new String(byteArrayOutputStream.toByteArray());
return resultData;
}
}
安卓http post
原创
©著作权归作者所有:来自51CTO博客作者friendan的原创作品,请联系作者获取转载授权,否则将追究法律责任
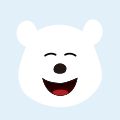
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
安卓逆向 -- POST数据解密
上节课内容安卓逆向 -- JEB3.7安装使用安卓逆向 -- Jeb动态调试一、通过上几节课我们知道要找的加密数据如
POST解密 安卓逆向 d3 动态调试 -
HTTP POST 请求学习