public class Binarytreedept { /* * 输出二叉树最小深度 * 核心思想:根节点到达最近的叶子节点的路径长度。 * 1、当根为空时,输出0。 * 2、当左子树为空时,输出右子树深度+1。 * 3、当右子树为空时,输出左子树深度+1。 * 4、以上条件都不满足时,输出min(左子树深度,右子树深度)+1。 * * 输出二叉树最大深度 * 核心思想:根节点到达最远的叶子节点的路径长度。 * 1、如果二叉树为空,则深度为0; * 2、如果不为空,分别求左子树的深度和右子树的深度,取较大值加1,因为根节点深度是1。 */ public static int minDepth(BiTreeNode root) { //如果二叉树为空,返回0; if(root == null) return 0; //如果二叉树左子树为空,返回右子树深度; if(root.lchild == null) return minDepth(root.rchild) + 1; //如果二叉树右子树为空,返回左子树深度; if(root.rchild == null) return minDepth(root.lchild) + 1; //如果二叉树左右子树均不为为空,返回左右子树深度的较小值; int leftDepth = minDepth(root.lchild); int rightDepth = minDepth(root.rchild); return leftDepth < rightDepth ? (leftDepth + 1) : (rightDepth + 1); } public static int maxDepth(BiTreeNode root) { //如果二叉树为空,返回0; if(root == null) return 0; //否则返回二叉树左右子树深度较大值; int leftDepth = maxDepth(root.lchild); int rightDepth = maxDepth(root.rchild); return leftDepth > rightDepth ? (leftDepth + 1) : (rightDepth + 1); } }
递归求取二叉树最小深度和最大深度
原创
©著作权归作者所有:来自51CTO博客作者递归梦想的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:经典汉诺塔递归实现
下一篇:M个数里面找出最大的N个数
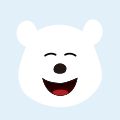
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】二叉树的存储结构
【数据结构】第五章——树与二叉树详细介绍二叉树的存储结构……
二叉树 数据结构 C语言 -
输出二叉树中的所有叶子节点
关键:叶子节点的左右子树都为空
树 子树 子节点 -
【二叉树】输出二叉树
题目在一个 m*n 的二维字符串数组中输出二叉树行数 m 应当等于给定二叉树的 高度列数 n 应当总是 奇数根节点 的值(以字符串格式
swift 算法 深度优先 二叉树 子树 -
【二叉树】删除给定值的叶子节点
题目给你一棵以 root 为根的二叉树和一个整数 target请你删除所有值为 target 的 叶子
swift 二叉树 算法 子节点 五笔 -
找到二叉树全部叶子节点的路径
算法笔记
Data 递归 数组 -
【二叉树】好叶子节点对的数量
题目给你二叉树的根节点 root 和一个整数 distance如果二叉树中两个 叶 节点之间的 最短路
swift 算法 二叉树 子节点 子树 -
【二叉树】层数最深叶子节点的和
题目给你一棵二叉树的根节点 root请你返回 层数最深 的叶子节点的和
swift 二叉树 算法 子节点 小程序 -
二叉树删除叶子节点代码Python 二叉树 删除节点
前面写过二叉树的节点插入与查找关键数据项以及最值的数据项。二叉树的删除与遍历是另外一项重要的操作。特别是二叉树的人删除比较复杂,分为无子节点的节点删除,只有一个子节点的节点删除和有两个子节点的节点删除三种情况。1. 删除没有子节点的节点 这种情况是三种节点删除中最简单
二叉树删除叶子节点代码Python 数据结构预算法 java 二叉树删除 子节点