文件切割合并器 3 合并类Merge
原创
©著作权归作者所有:来自51CTO博客作者明明如月学长的原创作品,请联系作者获取转载授权,否则将追究法律责任
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.GridBagLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.SequenceInputStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Enumeration;
import java.util.Properties;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
//用于切割后的文件合并
public class Merge extends InitFrame{
private static final long serialVersionUID = 1L;
public Merge()
{
super("文件合并","file_split.png",440,200);
initGUI();
this.setDefaultCloseOperation
(JFrame.DISPOSE_ON_CLOSE);
this.setVisible(true);
}
//初始化界面
public void initGUI() {
// 设置布局管理器
this.setLayout(new BorderLayout());
this.setResizable(false);
// 设置面板
northPanel = new JPanel();
southPanel = new JPanel();
// 设置面板布局
northPanel.setLayout(new GridBagLayout());
southPanel.setLayout(new FlowLayout());
// 将面板添加进窗体
this.add(northPanel,BorderLayout.CENTER);
this.add(southPanel,BorderLayout.SOUTH);
// 设置北部面板
label_1 = new JLabel("切割文件所在目录:");
label_2 = new JLabel("请选择目标文件夹:");
field_1 = new JTextField(20);
field_2 = new JTextField(20);
button_1 = new JButton("选择",new ImageIcon(Merge.class.getResource("/resources/open.png")));
button_1.addActionListener(listener);
button_2 = new JButton("选择",new ImageIcon(Merge.class.getResource("/resources/open.png")));
button_2.addActionListener(listener);
northPanel.add(label_1,new GBC(0,0).setInsets(1));
northPanel.add(field_1,new GBC(1,0).setInsets(1));
northPanel.add(button_1,new GBC(2,0).setInsets(1));
northPanel.add(label_2,new GBC(0,1).setInsets(1));
northPanel.add(field_2,new GBC(1,1).setInsets(1));
northPanel.add(button_2,new GBC(2,1).setInsets(1));
// 设置南部面板
button_Merge= new JButton("
合 并
",new ImageIcon(Merge.class.getResource("/resources/merge.png")));
button_Merge.addActionListener(listener);
button_open = new JButton("打开目标文件夹",new ImageIcon(Merge.class.getResource("/resources/open_dir.png")));
button_open.addActionListener(listener);
southPanel.add(button_Merge);
southPanel.add(button_open);
this.setVisible(true);
}
private class ChoiceListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
if(e.getSource().equals(button_1))
{
JFileChooser chooser = new JFileChooser();
//
只许选择文件夹
chooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
//
不允许同时选中多个文件夹
chooser.setMultiSelectionEnabled
(false);
int returnVal = chooser.showOpenDialog(Merge.this);
if(returnVal == JFileChooser.APPROVE_OPTION) {
//
获取选择的文件
dir_source=
chooser.getSelectedFile();
//
获取的文件名
dirName = dir_source.getAbsolutePath();
//
将获取的源文件名显示
field_1.setText(dirName);
}
}else if(e.getSource().equals(button_2))
{
JFileChooser chooser = new JFileChooser();
//
只许选择文件夹
chooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
//
不允许同时选中多个文件夹
chooser.setMultiSelectionEnabled
(false);
int returnVal = chooser.showOpenDialog(Merge.this);
if(returnVal == JFileChooser.APPROVE_OPTION) {
//
获取选择的目标文件夹
dir_des=
chooser.getSelectedFile();
//
将获取的目标文件名显示
field_2.setText(dir_des.getAbsolutePath());
}
}else if(e.getSource().equals(button_Merge))
{
MergeFiles(dir_source,dir_des);
}else if(e.getSource().equals(button_open))
{
//
获取目标文件目录路径
String dir_path = dir_des.getAbsolutePath();
//
打开目标文件目录
Runtime r = Runtime.getRuntime();
try {
r.exec("cmd.exe /c start "+dir_path);
} catch (IOException e1) {
//
打开存放切割目录失败是给予提示
JLabel label_result = new JLabel("打开目标目录失败!!");
JOptionPane.showConfirmDialog(Merge.this, label_result,"文件合并-打开目录", JOptionPane.INFORMATION_MESSAGE,JOptionPane.OK_OPTION, new ImageIcon(Merge.class.getResource("/resources/null.png")));
}
}
}
private void MergeFiles(File dir_source, File dir_des)
{
File [] fileinfo = dir_source.listFiles(new SuffixFilter(".properties"));
// 当配置信息为0个或者两个或者两个以上时,无法进行判断,给出提示信息
if(fileinfo.length!=1)
{
JLabel label_result = new JLabel("无配置信息,或者配置信息不唯一,无法合并文件!!");
JOptionPane.showConfirmDialog(Merge.this, label_result,"文件合并-错误提示", JOptionPane.INFORMATION_MESSAGE,JOptionPane.OK_OPTION, new ImageIcon(Merge.class.getResource("/resources/null.png")));
}
else{
//
获取配置信息
Properties prop = new Properties();
try {
FileInputStream fis = new FileInputStream(fileinfo[0]);
// 将配置信息加载到流中
prop.load(fis);
// 获取文件名和截取片段个数
filename = prop.getProperty("filename");
int count = Integer.parseInt(prop.getProperty("splitcount"));
File []
files = dir_source.listFiles(new SuffixFilter(".split"));
System.out.println(count-1);
System.out.println(files.length);
if(files.length!=(count-1))
{
JLabel label_result = new JLabel("截取片段数目无效,应该是"+(count-1)+"个!!");
JOptionPane.showConfirmDialog(Merge.this, label_result,"文件合并-错误提示", JOptionPane.INFORMATION_MESSAGE,JOptionPane.OK_OPTION, new ImageIcon(Merge.class.getResource("/resources/null.png")));
}else{
// 定义泛型集合 将切割后的文件和刘相关联并存储到几个里
ArrayList<FileInputStream > arraylist = new ArrayList<FileInputStream >();
for(int i= 0;i< files.length;i++)
{
arraylist.add(new FileInputStream(files[i]));
}
//
将数个流合并成一个流
Enumeration< FileInputStream> enumer = Collections.enumeration(arraylist);
SequenceInputStream sis = new SequenceInputStream(enumer);
// 定义输出流
FileOutputStream fos = new FileOutputStream(new File(dir_des,filename));
byte [] buf = new byte[1024*1024];
int len =0;
// 按每次1M写入文件
while((len= sis.read(buf))!=-1)
{
fos.write(buf, 0, len);
}
// 关闭流
fos.close();
sis.close();
JLabel label_result = new JLabel("已经成功将切割文件合并到目标文件夹!!");
JOptionPane.showConfirmDialog(Merge.this, label_result,"文件合并-成功提示", JOptionPane.INFORMATION_MESSAGE,JOptionPane.OK_OPTION, new ImageIcon(Merge.class.getResource("/resources/succes.png")));
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
private
ChoiceListener listener = new ChoiceListener();
private JPanel northPanel;
private JPanel southPanel;
private JLabel label_1;
private JLabel label_2;
private JTextField field_1;
private JTextField field_2;
private JButton button_1;
private JButton button_2;
private JButton button_Merge;
private JButton button_open;
private File dir_source=null;
private File dir_des=null;
private String dirName= "";
private
String filename;
}
上一篇:文件切割合并器 收获感悟
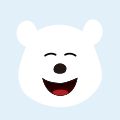
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
有关树JTree的类和接口JTree
有关树JTree的类和接口JTree 显示树的核心基本类。TreeMode
职场 接口 休闲 JTree -
&nb
初学Linux
Linux 博客 操作系统