import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MovingHeart extends JFrame {
private int x = 0;
private int y = 100;
private int size = 50;
private Color color = Color.RED;
public MovingHeart() {
setTitle("动态爱心");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setResizable(false);
Timer timer = new Timer(50, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
x += 5;
if (x > getWidth()) {
x = -size;
}
repaint();
}
});
timer.start();
}
@Override
public void paint(Graphics g) {
super.paint(g);
Graphics2D g2d = (Graphics2D) g;
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setColor(color);
g2d.fillOval(x, y, size, size);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new MovingHeart().setVisible(true);
}
});
}
}
Java爱心代码
原创
©著作权归作者所有:来自51CTO博客作者lizhao1688的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:Java“不死”神兔
下一篇:浅谈Java
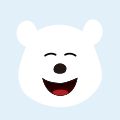
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
java跳动爱心代码
Java跳动爱心代码示例。
Java java 2d -
Java爱心空心 java爱心代码
相信对于打印三角形都没什么难度,只需要利用for循环嵌套使用就行但是对于打印圆形和三角形不同因为到圆心距离相等的点一般不会横坐标和纵坐标都为整数
Java爱心空心 java心形代码 热门搜索词java实现代码 输出以下图案菱形7行 i++ -
javacv 提高人脸准确的
技术栈:Spring boot 2.x腾讯java版本1.8注意事项:本文内的“**.**”需要自己替换为自己的路径。常量内的“**”需要自己定义自己内容。业务中认证图片,上传至阿里云OSS上话不多说,直接上代码1、pom文件:<!-- apache httpclient组件 --> <dependency> <groupId>org.apache.h
javacv 提高人脸准确的 java java AI 对接 java腾讯AI对接 java人脸对比