// jsp页面
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%><!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<form action="${pageContext.request.contextPath}/Test" enctype="multipart/form-data" method="post">
上传用户:<input type="text" name="username"/><br/>
上传文件1:<input type="file" name="file1"/><br/>
上传文件2:<input type="file" name="file2" /><br/>
<input type="submit" value="提交"/>
</form>
</body>
</html>
//servlet
package com.kero99.ygc.test;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.List;import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.FileUploadException;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;public class Test extends HttpServlet {
/**
* Constructor of the object.
*/
public Test() {
super();
} /**
* Destruction of the servlet. <br>
*/
public void destroy() {
super.destroy(); // Just puts "destroy" string in log
// Put your code here
} /**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
} /**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to post.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
DiskFileItemFactory diskFileItemFactory=new DiskFileItemFactory();
ServletFileUpload fileUpload=new ServletFileUpload(diskFileItemFactory);
try {
List<FileItem> list=fileUpload.parseRequest(request);
for(FileItem item : list){
System.out.println("-------------------------");
String origname=item.getName();//原始文件名
String context=item.getString("UTF-8");//获得文本项的内容
String value1=new String (context.getBytes("iso8859-1"),"UTF-8");
String fieldName=item.getFieldName();//获得文本域的名字
String contentType=item.getContentType();//获得文本域内容的类型
long size=item.getSize();//获取文件大小
boolean isFieldFrom=item.isFormField();//判断是不是文本域
System.out.println("原始文件名getName---"+origname);
System.out.println("获得文本项的内容context---"+context);
System.out.println("文本项的内容getString---"+value1);
System.out.println("文本域的名字FieldName---"+fieldName);
System.out.println("文本域内容的类型contentType---"+contentType);
System.out.println("文件大小---"+size);
System.out.println("是不是文本域isFrom---"+isFieldFrom);
System.out.println("-------------------------");
}
} catch (FileUploadException e) {
e.printStackTrace();
}
} /**
* Initialization of the servlet. <br>
*
* @throws ServletException if an error occurs
*/
public void init() throws ServletException {
// Put your code here
}}
//web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<display-name></display-name>
<servlet>
<servlet-name>Test</servlet-name>
<servlet-class>com.kero99.ygc.test.Test</servlet-class>
</servlet> <servlet-mapping>
<servlet-name>Test</servlet-name>
<url-pattern>/Test</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
上传文件常用api
原创
©著作权归作者所有:来自51CTO博客作者南归北隐的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:java文件上传功能
下一篇:java实现简单的文件下载
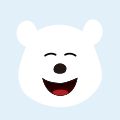
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
C# Web Api 上传文件
一、 使用默认方法上传文件: 1、Action: /// <sum
ide 后缀名 html -
文件上传api——MultipartFile
MultipartFile 方法总结 byte[] getBytes() 返回文件的内容作为一个字节数组。 String getContentType() 返回文件的内容类型。 InputStream getInputStream()
java经验集锦 通用实践 Spring SpringMVC 文件系统