//2016-08-26 /** * Check that the given file is a valid file instance. * * @param mixed $file * @return bool */ protected function isValidFile($file) { return $file instanceof SplFileInfo && $file->getPath() != ''; // if both about this is ok than it is ok }//Check that the given file is a valid file instance. /** * Retrieve a header from the request. * * @param string $key * @param string|array|null $default * @return string|array */ public function header($key = null, $default = null) { return $this->retrieveItem('headers', $key, $default); }//Retrieve a header from the request. // retrieve like search // get the request header // use a wrap function to set this options to get the value /** * Retrieve a server variable from the request. * * @param string $key * @param string|array|null $default * @return string|array */ public function server($key = null, $default = null) { return $this->retrieveItem('server', $key, $default); }// like brother /** * Retrieve an old input item. * * @param string $key * @param string|array|null $default * @return string|array */ public function old($key = null, $default = null) { return $this->session()->getOldInput($key, $default); }// this is amazing method to get you old boy friend // like get you history /** * Flash the input for the current request to the session. * * @param string $filter * @param array $keys * @return void */ public function flash($filter = null, $keys = []) { $flash = (! is_null($filter)) ? $this->$filter($keys) : $this->input(); $this->session()->flashInput($flash);// use a flash method }// flash the input for the current request to the session /** * Flash only some of the input to the session. * * @param array|mixed $keys * @return void */ public function flashOnly($keys) { $keys = is_array($keys) ? $keys : func_get_args();// get the keys return $this->flash('only', $keys);// return this flash }// flash only some of the input to the session /** * Flash only some of the input to the session. * * @param array|mixed $keys * @return void */ public function flashExcept($keys) { $keys = is_array($keys) ? $keys : func_get_args();// get this right key. return $this->flash('except', $keys);// use a key to store this value }//flash only some of the input to the session. /** * Flush all of the old input from the session. * * @return void */ public function flush() { $this->session()->flashInput([]); }// if this values is null flash all value. /** * Retrieve a parameter item from a given source. * * @param string $source * @param string $key * @param string|array|null $default * @return string|array */ protected function retrieveItem($source, $key, $default) { if (is_null($key)) { return $this->$source->all(); }// is_null return $this->$source->get($key, $default);// get the key }//this is the retrieve method ,like a search function. /** * Merge new input into the current request's input array. * * @param array $input * @return void */ public function merge(array $input) { $this->getInputSource()->add($input);// to get the Source }//get the merge /** * Replace the input for the current request. * * @param array $input * @return void */ public function replace(array $input) { $this->getInputSource()->replace($input); }//replace the input for the current request. /** * Get the JSON payload for the request. * * @param string $key * @param mixed $default * @return mixed */ public function json($key = null, $default = null) { if (! isset($this->json)) { $this->json = new ParameterBag((array) json_decode($this->getContent(), true)); }//set this json if (is_null($key)) { return $this->json; }// return all return data_get($this->json->all(), $key, $default);// get the key from this store array }//get the JSON payload fro the request. /** * Get the input source for the request. * * @return \Symfony\Component\HttpFoundation\ParameterBag */ protected function getInputSource() { if ($this->isJson()) { return $this->json(); }// if it is a Json return $this->getMethod() == 'GET' ? $this->query : $this->request;// get this GET }//Get the input source for the request /** * Determine if the given content types match. * * @param string $actual * @param string $type * @return bool */ public static function matchesType($actual, $type) {// Determine if the given content types match. if ($actual === $type) { return true; }// determine this two type is ok $split = explode('/', $actual);//get this value if (isset($split[1]) && preg_match('/'.$split[0].'\/.+\+'.$split[1].'/', $type)) { return true; }//has this check return false; }// default it is wrong. /** * Determine if the request is sending JSON. * * @return bool */ public function isJson() { return Str::contains($this->header('CONTENT_TYPE'), ['/json', '+json']); // Str:: contains // to set this header }//Determine if the request is sending JSON /** * Determine if the current request is asking for JSON in return. * * @return bool */ public function wantsJson() { $acceptable = $this->getAcceptableContentTypes();// use a magic function return isset($acceptable[0]) && Str::contains($acceptable[0], ['/json', '+json']); }// check this request method to return by json type /** * Determines whether the current requests accepts a given content type. * * @param string|array $contentTypes * @return bool */ public function accepts($contentTypes) {//Determines whether the current requests accepts a given content type. $accepts = $this->getAcceptableContentTypes();// get this accepts if (count($accepts) === 0) { return true; }// if count is zero will return true. $types = (array) $contentTypes;// get this type foreach ($accepts as $accept) {// loop accepts as accept if ($accept === '*/*' || $accept === '*') { return true; }// if it is all ,will return good foreach ($types as $type) {// loop this can be accept types ,and match it if ($this->matchesType($accept, $type) || $accept === strtok($type, '/').'/*') { return true; }// if has it ,just return true. } } return false; } /** * Return the most suitable content type from the given array based on content negotiation. * * @param string|array $contentTypes * @return string|null */ public function prefers($contentTypes) {//return the most suitable content type from the given array based on content negotiation. $accepts = $this->getAcceptableContentTypes();// get this accept type $contentTypes = (array) $contentTypes;// change this best type. foreach ($accepts as $accept) {// loop again , i can see the after if (in_array($accept, ['*/*', '*'])) { return $contentTypes[0]; }// in_array foreach ($contentTypes as $contentType) {// loop this contentTypes $type = $contentType;// type if (! is_null($mimeType = $this->getMimeType($contentType))) { $type = $mimeType; }// get this mimeType; if ($this->matchesType($type, $accept) || $accept === strtok($type, '/').'/*') { return $contentType; }// matches Type } } } /** * Determines whether a request accepts JSON. * * @return bool */ public function acceptsJson() { return $this->accepts('application/json'); }// a wrap about json /** * Determines whether a request accepts HTML. * * @return bool */ public function acceptsHtml() { return $this->accepts('text/html'); }// a wrap about html /** * Get the data format expected in the response. * * @param string $default * @return string */ public function format($default = 'html') { foreach ($this->getAcceptableContentTypes() as $type) { if ($format = $this->getFormat($type)) { return $format; } }// loop for every thing return $default; }// i like this function to format every thing /** * Get the bearer token from the request headers. * * @return string|null */ public function bearerToken() { $header = $this->header('Authorization', ''); if (Str::startsWith($header, 'Bearer ')) { return Str::substr($header, 7); }// get the Str:: }// bearer Token /** * Create an Illuminate request from a Symfony instance. * * @param \Symfony\Component\HttpFoundation\Request $request * @return \Illuminate\Http\Request */ public static function createFromBase(SymfonyRequest $request) {//Create an Illuminate request from a Symfony instance. if ($request instanceof static) { return $request; }// request instanceof static $content = $request->content;// get this content $request = (new static)->duplicate( $request->query->all(), $request->request->all(), $request->attributes->all(), $request->cookies->all(), $request->files->all(), $request->server->all() );// get request $request->content = $content; $request->request = $request->getInputSource(); return $request; }// a wrap function /** * {@inheritdoc} */ public function duplicate(array $query = null, array $request = null, array $attributes = null, array $cookies = null, array $files = null, array $server = null) { return parent::duplicate($query, $request, $attributes, $cookies, array_filter((array) $files), $server); }// duplicate is a good function /** * Get the session associated with the request. * * @return \Illuminate\Session\Store * * @throws \RuntimeException */ public function session() { if (! $this->hasSession()) { throw new RuntimeException('Session store not set on request.'); }// has Session return $this->getSession();// get Session }//Get the session associated withe the request. /** * Get the user making the request. * * @param string|null $guard * @return mixed */ public function user($guard = null) { return call_user_func($this->getUserResolver(), $guard);// just call user fucntion }//Get the user making the request. /** * Get the route handling the request. * * @param string|null $param * * @return \Illuminate\Routing\Route|object|string */ public function route($param = null) {//Get the route handling the request. $route = call_user_func($this->getRouteResolver());// a function to get route if (is_null($route) || is_null($param)) { return $route;// get this route } else { return $route->parameter($param);// get the parameter } } /** * Get a unique fingerprint for the request / route / IP address. * * @return string * * @throws \RuntimeException */ public function fingerprint() {// get the unique fingerprint for the request /route /IP address if (! $this->route()) { throw new RuntimeException('Unable to generate fingerprint. Route unavailable.'); }// if throw new Runtime Exception return sha1( implode('|', $this->route()->methods()). '|'.$this->route()->domain(). '|'.$this->route()->uri(). '|'.$this->ip() );// a sha1 } /** * Get the user resolver callback. * * @return \Closure */ public function getUserResolver() { return $this->userResolver ?: function () { // }; }//Get the user resolver callback /** * Set the user resolver callback. * * @param \Closure $callback * @return $this */ public function setUserResolver(Closure $callback) { $this->userResolver = $callback; return $this;//a function }// Set the user resolver callback /** * Get the route resolver callback. * * @return \Closure */ public function getRouteResolver() { return $this->routeResolver ?: function () { // };// a null function }//Get the route resolver callback. /** * Set the route resolver callback. * * @param \Closure $callback * @return $this */ public function setRouteResolver(Closure $callback) { $this->routeResolver = $callback; return $this; }//Set the route resolver callback. /** * Get all of the input and files for the request. * * @return array */ public function toArray() { return $this->all();// return all }//Get all of the input and files for the request. /** * Determine if the given offset exists. * * @param string $offset * @return bool */ public function offsetExists($offset) { return array_key_exists($offset, $this->all()); }//array_key_exists /** * Get the value at the given offset. * * @param string $offset * @return mixed */ public function offsetGet($offset) { return data_get($this->all(), $offset); }//get the offset Get /** * Set the value at the given offset. * * @param string $offset * @param mixed $value * @return void */ public function offsetSet($offset, $value) { return $this->getInputSource()->set($offset, $value); }// offset Set /** * Remove the value at the given offset. * * @param string $offset * @return void */ public function offsetUnset($offset) { return $this->getInputSource()->remove($offset); }// Unset /** * Check if an input element is set on the request. * * @param string $key * @return bool */ public function __isset($key) { return ! is_null($this->__get($key)); }// isset method /** * Get an input element from the request. * * @param string $key * @return mixed */ public function __get($key) { $all = $this->all(); if (array_key_exists($key, $all)) { return $all[$key]; } else { return $this->route($key); } }// a magic get }
[李景山php]每天laravel-20161022|Request.php-3
原创
©著作权归作者所有:来自51CTO博客作者lijingsan1的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:[李景山php]每天laravel-20161021|Request.php-2
下一篇:[李景山php]每天laravel-20161026|TranslationServiceProvider.php
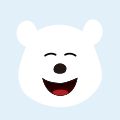
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章