/** * Given two date/time strings, check that one is after the other. * * @param string $format * @param string $before * @param string $after * @return bool */ protected function checkDateTimeOrder($format, $before, $after) { $before = $this->getDateTimeWithOptionalFormat($format, $before);// format it $after = $this->getDateTimeWithOptionalFormat($format, $after);// format after return ($before && $after) && ($after > $before);// a big logic }// get two date/time strings, check that one is after the other. /** * Get a DateTime instance from a string. * * @param string $format * @param string $value * @return \DateTime|null */ protected function getDateTimeWithOptionalFormat($format, $value) {//Get a DateTime instance from a sting. $date = DateTime::createFromFormat($format, $value);//Date::createFromFormat if ($date) {// if date return $date; } try {// return new DateTime return new DateTime($value); } catch (Exception $e) { // do nothing } } /** * Validate that an attribute is a valid timezone. * * @param string $attribute * @param mixed $value * @return bool */ protected function validateTimezone($attribute, $value) { try { new DateTimeZone($value); } catch (Exception $e) { return false; } return true; }//validate timezone /** * Get the date format for an attribute if it has one. * * @param string $attribute * @return string|null */ protected function getDateFormat($attribute) { if ($result = $this->getRule($attribute, 'DateFormat')) { return $result[1][0]; } }// Get the date format from an attribute if it has one. /** * Get the validation message for an attribute and rule. * * @param string $attribute * @param string $rule * @return string */ protected function getMessage($attribute, $rule) { $lowerRule = Str::snake($rule);// use snake method to get this you want $inlineMessage = $this->getInlineMessage($attribute, $lowerRule);// get message // First we will retrieve the custom message for the validation rule if one // exists. If a custom validation message is being used we'll return the // custom message, otherwise we'll keep searching for a valid message. if (! is_null($inlineMessage)) { return $inlineMessage; }// if it is a good message $customKey = "validation.custom.{$attribute}.{$lowerRule}";// this can get a good key $customMessage = $this->getCustomMessageFromTranslator($customKey); // First we check for a custom defined validation message for the attribute // and rule. This allows the developer to specify specific messages for // only some attributes and rules that need to get specially formed. if ($customMessage !== $customKey) { return $customMessage; }// return the custom message // If the rule being validated is a "size" rule, we will need to gather the // specific error message for the type of attribute being validated such // as a number, file or string which all have different message types. elseif (in_array($rule, $this->sizeRules)) { return $this->getSizeMessage($attribute, $rule); }// specific error // Finally, if no developer specified messages have been set, and no other // special messages apply for this rule, we will just pull the default // messages out of the translator service for this validation rule. $key = "validation.{$lowerRule}"; if ($key != ($value = $this->translator->trans($key))) { return $value; }// just message return $this->getInlineMessage( $attribute, $lowerRule, $this->fallbackMessages ) ?: $key;// return key }
[李景山php]每天laravel-20161011|Validator.php-11
原创
©著作权归作者所有:来自51CTO博客作者lijingsan1的原创作品,请联系作者获取转载授权,否则将追究法律责任
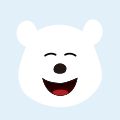
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
[李景山php]每天laravel-20161004|Validator.php-4
/** * Determine if it's a necessary presence validation. * * This&nbs
comparison determine necessary -
[李景山php]每天laravel-20161013|Validator.php-13
/** * Get the displayable name of the attribute. *
return developer function