namespace Illuminate\Filesystem; use ErrorException; use FilesystemIterator; use Symfony\Component\Finder\Finder; use Illuminate\Support\Traits\Macroable; use Illuminate\Contracts\Filesystem\FileNotFoundException; // long time ago ,never see namespace so better, now I will use it more class Filesystem { use Macroable;// this is a trait class like a real class /** * Determine if a file or directory exists. * * @param string $path * @return bool */ public function exists($path) { return file_exists($path);// a method wrap }// Determine if a file or directory exists. /** * Get the contents of a file. * * @param string $path * @return string * * @throws \Illuminate\Contracts\Filesystem\FileNotFoundException */ public function get($path) { if ($this->isFile($path)) {// Determine is a file return file_get_contents($path);//get file contents } throw new FileNotFoundException("File does not exist at path {$path}"); }// get file contents
每天laravel-20160910|Filesystem-1
原创
©著作权归作者所有:来自51CTO博客作者lijingsan1的原创作品,请联系作者获取转载授权,否则将追究法律责任
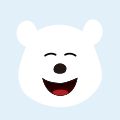
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
laravel实现高并发限流解决方案之令牌桶算法
限流 laravel限流 高并发
php App redis