/** * Resolve a non-class hinted dependency. * * @param \ReflectionParameter $parameter * @return mixed * * @throws \Illuminate\Contracts\Container\BindingResolutionException */ protected function resolveNonClass(ReflectionParameter $parameter) {// the parameter is a RefectionParameter $parameter if (! is_null($concrete = $this->getContextualConcrete('$'.$parameter->name))) { // if can get the concrete if ($concrete instanceof Closure) {// if the concrete is a instance of Closure return call_user_func($concrete, $this);// return the call_user_func } else { return $concrete;// return concrete } } if ($parameter->isDefaultValueAvailable()) {// if has return $parameter->getDefaultValue();// return it } // other ,set the bad message and return it // some time the message is very good use double " ,yeah $message = "Unresolvable dependency resolving [$parameter] in class {$parameter->getDeclaringClass()->getName()}"; throw new BindingResolutionException($message);// throw bad message } /** * Resolve a class based dependency from the container. * * @param \ReflectionParameter $parameter * @return mixed * * @throws \Illuminate\Contracts\Container\BindingResolutionException */ protected function resolveClass(ReflectionParameter $parameter) {// the class is based dependency from the container. try { return $this->make($parameter->getClass()->name); }// try check this class name whether can be make // If we can not resolve the class instance, we will check to see if the value // is optional, and if it is we will return the optional parameter value as // the value of the dependency, similarly to how we do this with scalars. catch (BindingResolutionException $e) {// catch the exception if ($parameter->isOptional()) {// if we has the Optional return $parameter->getDefaultValue(); } throw $e;// throw $e } }
每天laravel-20160815| Container -18
原创
©著作权归作者所有:来自51CTO博客作者lijingsan1的原创作品,请联系作者获取转载授权,否则将追究法律责任
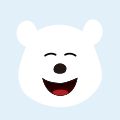
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
51c视觉~合集18视觉
-
每天laravel-20160807| Container -10
/** * Alias a type to a different name. * * @param string&n
different function definition