package com.itjiandan.servlet; import java.io.IOException; import java.lang.reflect.Method; import java.sql.Timestamp; import java.util.HashMap; import java.util.List; import java.util.Map; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import com.itjiandan.dao.IAuthority_DAO; import com.itjiandan.dao.ICommodityClass_DAO; import com.itjiandan.dao.ICommodity_DAO; import com.itjiandan.dao.IGroup_DAO; import com.itjiandan.dao.IUser_DAO; import com.itjiandan.daoimpl.Authority_DAOImpl; import com.itjiandan.daoimpl.CommodityClass_DAOImpl; import com.itjiandan.daoimpl.Commodity_DAOImpl; import com.itjiandan.daoimpl.Group_DAOImpl; import com.itjiandan.daoimpl.User_DAOImpl; import com.itjiandan.model.Authority_Entity; import com.itjiandan.model.CommodityClass_Entity; import com.itjiandan.model.Commodity_Entity; import com.itjiandan.model.Group_Entity; import com.itjiandan.model.User_Entity; import com.itjiandan.util.DeleteFileUtil; import com.itjiandan.util.FileLoadUtil; import com.itjiandan.util.IPTimeRandom; import com.itjiandan.util.LogUtil; @SuppressWarnings("serial") public class AdminServlet extends HttpServlet { private static AdminServlet adminServlet; private static Map<String,String> myFLMap; private static String myform; private static String method; private static String datename; static { adminServlet = new AdminServlet(); } protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{ doPost(req,resp); } protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{ req.setCharacterEncoding("UTF-8"); resp.setCharacterEncoding("UTF-8"); myform = req.getParameter("myform"); method = req.getParameter("method"); //LogUtil.i(this.getServletContext().getRealPath("/") + "p_w_picpaths\\"); //LogUtil.i(req.getSession().getServletContext().getRealPath("/")); if(method == null && myform == null){ IPTimeRandom itr = new IPTimeRandom(req.getRemoteAddr()); datename = itr.getIPTimeRandom() + "."; LogUtil.i("datename=" + datename + ",ip=" + req.getRemoteAddr()); String path = req.getSession().getServletContext().getRealPath("/") + "p_w_picpaths"+"\\" + datename; myFLMap = FileLoadUtil.fileLoad(req, path); myform = myFLMap.get("myform"); method = myFLMap.get("method"); } LogUtil.i("AdminServlet----->myform="+myform); Method methods; try { methods = AdminServlet.class.getDeclaredMethod(myform, HttpServletRequest.class, HttpServletResponse.class); methods.invoke(adminServlet, req,resp); } catch (Exception e) { e.printStackTrace(); } } /** * 用户登入 * @param req * @param resp * @throws ServletException * @throws IOException */ public void login(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{ String method = req.getParameter("method"); if("login".equals(method)) { String name = req.getParameter("name"); String pass = req.getParameter("pass"); LogUtil.i("login--->name="+name+",password="+pass); IUser_DAO iuser = new User_DAOImpl(); User_Entity user = iuser.getUser(name, pass); if(user != null) { int uid = user.getId(); IGroup_DAO igroup = new Group_DAOImpl(); Group_Entity group = igroup.getGroupByUserId(uid); int gid = group.getId(); List<Authority_Entity> authoritys = igroup.getGroupAuthorityById(gid); Map<String, String> myAMap = new HashMap<String,String>(); for(int i=0; i<authoritys.size(); i++){ Authority_Entity authority = authoritys.get(i); String key = authority.getMyform() +"|" + authority.getMethod(); myAMap.put(key, authority.getPath()); } req.getSession().setAttribute("#myAMap#", myAMap); req.getSession().setAttribute("#user#", user); req.getRequestDispatcher("/WEB-INF/admin/index.jsp").forward(req, resp); }else{ req.setAttribute("false", "false"); req.getRequestDispatcher("/WEB-INF/admin/login.jsp").forward(req, resp); } }else if("showlogin".equals(method)){ req.getRequestDispatcher("/WEB-INF/admin/login.jsp").forward(req, resp); }else if("logout".equals(method)){ String out = req.getParameter("out"); if(out != null)req.getRequestDispatcher("/WEB-INF/admin/login.jsp").forward( req, resp); req.getRequestDispatcher("/WEB-INF/admin/logout.jsp").forward(req,resp); } } /** * 用户列表 * @param req * @param resp * @throws ServletException * @throws IOException */ public void userList(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{ IGroup_DAO igroup = new Group_DAOImpl(); IUser_DAO iuser = new User_DAOImpl(); User_Entity user = null; //Group_Entity group = null; String method = req.getParameter("method"); if("showuserlist".equals(method)) { String p = req.getParameter("page"); String s = req.getParameter("size"); int page = p == null ? 1 : Integer.valueOf(p); int size = s == null ? 5 : Integer.valueOf(s); List<User_Entity> users = iuser.getPageUser(page, size); int count = iuser.getCountUser(); if(users.size() >= 1){ req.setAttribute("users", users); req.setAttribute("page", page); req.setAttribute("size", size); req.setAttribute("count", count); req.getRequestDispatcher("/WEB-INF/admin/userlist.jsp").forward(req, resp); }else{ req.setAttribute("userlist", "no data"); req.getRequestDispatcher("/WEB-INF/admin/userlist.jsp").forward(req, resp); } }else if("adduser".equals(method)) { String name = req.getParameter("name"); String pass = req.getParameter("pass"); String a = req.getParameter("age"); String sex = req.getParameter("sex"); String job = req.getParameter("job"); String mail = req.getParameter("mail"); String g = req.getParameter("gid"); String s = req.getParameter("state"); int age = Integer.valueOf(a); int gid = Integer.valueOf(g); int state = Integer.valueOf(s); Timestamp createdate = new Timestamp(System.currentTimeMillis()); user = new User_Entity(null, name, pass, age, sex, job, mail, createdate, null, state); boolean flse = iuser.addUser(user); if(flse){ iuser.addUserGroup(name, gid); resp.sendRedirect(req.getContextPath()+"/AdminServlet?myform=userList&" + "method=showuserlist"); } }else if("showadduser".equals(method)) { String i = req.getParameter("uid"); if(i != null && i != ""){ LogUtil.i("showadduser---->id="+i); user = iuser.upUserById(Integer.valueOf(i)); req.setAttribute("user", user); } List<Group_Entity> groups = igroup.getPageGroup(1, 50); req.setAttribute("groups", groups); req.getRequestDispatcher("/WEB-INF/admin/userinfo.jsp").forward(req, resp); }else if("upuser".equals(method)) { String u = req.getParameter("uid"); String name = req.getParameter("name"); String a = req.getParameter("age"); String sex = req.getParameter("sex"); String job = req.getParameter("job"); String mail = req.getParameter("mail"); String g = req.getParameter("gid"); String s = req.getParameter("state"); int uid = Integer.valueOf(u); int age = Integer.valueOf(a); int state = Integer.valueOf(s); user = new User_Entity(uid, name, null, age, sex, job, mail, null, null, state); boolean flse = iuser.upUser(user); iuser.delUserGroup(uid); if(flse){ if(g != null && g != ""){ int gid = Integer.valueOf(g); iuser.addUserGroup(name, gid); } resp.sendRedirect(req.getContextPath()+"/AdminServlet?myform=userList&" + "method=showuserlist"); } }else if("deluser".equals(method)) { String[] i = req.getParameterValues("uid"); int k = 1; if(i != null ){ LogUtil.i("deluser------->选中"+i.length+"个"); int[] id = new int[i.length]; for(int j=0; j<i.length; j++){ id[j] = Integer.valueOf(i[j]); k ++; } iuser.delUser(id); if( k == i.length)req.setAttribute("deluser", "succeed"); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=userList&method=showuserlist"); } } /** * 角色列表 * @param req * @param resp * @throws ServletException * @throws IOException */ public void groupList(HttpServletRequest req,HttpServletResponse resp) throws ServletException,IOException{ IGroup_DAO igroup = new Group_DAOImpl(); IAuthority_DAO iauthority = new Authority_DAOImpl(); List<Authority_Entity> authoritys = null; Authority_Entity oneauthority = null; Authority_Entity twoauthority = null; Group_Entity group = null; String method = req.getParameter("method"); LogUtil.i("groupList---->method="+method); if("showgrouplist".equals(method)) { String p = req.getParameter("page"); String s = req.getParameter("size"); int page = p == null ? 1 : Integer.valueOf(p); int size = s == null ? 5 : Integer.valueOf(s); List<Group_Entity> groups = igroup.getPageGroup(page, size); int count = igroup.getCountGroup(); if(groups.size() >= 1){ req.setAttribute("groups", groups); req.setAttribute("page", page); req.setAttribute("size", size); req.setAttribute("count", count); req.getRequestDispatcher("/WEB-INF/admin/grouplist.jsp").forward(req, resp); }else{ req.setAttribute("grouplist", "no data"); req.getRequestDispatcher("/WEB-INF/admin/grouplist.jsp").forward(req, resp); } }else if("addgroup".equals(method)) { String name = req.getParameter("name"); String s = req.getParameter("state"); String[] a = req.getParameterValues("aid"); LogUtil.i("addgroup----->共有"+a.length+"个aid"); int state = Integer.valueOf(s); Timestamp createdate = new Timestamp(System.currentTimeMillis()); group = new Group_Entity(null, name, createdate, null, state); boolean flse = igroup.addGroup(group); if(flse){ int[] aid = new int[a.length]; for(int i=0; i<a.length; i++){ aid[i] = Integer.valueOf(a[i]); } igroup.addGroupAuthority(name, aid); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=groupList&method=showgrouplist"); } else if("showaddgroup".equals(method)) { String g = req.getParameter("gid"); Map<String, String> myMap = new HashMap<String,String>(); if(g != null){ int id = Integer.valueOf(g); authoritys = igroup.getGroupAuthorityById(id); if(authoritys.size() >= 1){ for(int i=0; i<authoritys.size(); i++){ myMap.put(authoritys.get(i).getId()+"", ""); } } group = igroup.upGroupById(id); } List<Authority_Entity> oneauthoritys = iauthority.getPageAuthority(0, 1, 100); for(int o=0; o<oneauthoritys.size(); o++){ oneauthority = oneauthoritys.get(o); List<Authority_Entity> twoauthoritys = iauthority.getPageAuthority(oneauthority.getId(), 1, 100); oneauthority.setList(twoauthoritys); for(int t=0; t<oneauthority.getList().size(); t++){ twoauthority = oneauthority.getList().get(t); List<Authority_Entity> threeauthoritys = iauthority.getPageAuthority(twoauthority.getId(), 1, 100); twoauthority.setList(threeauthoritys); } } req.setAttribute("myMap", myMap); req.setAttribute("group", group); req.setAttribute("oneauthoritys", oneauthoritys); req.getRequestDispatcher("/WEB-INF/admin/groupinfo.jsp").forward(req, resp); } else if("upgroup".equals(method)) { String g = req.getParameter("gid"); String name = req.getParameter("name"); String s = req.getParameter("state"); String[] a = req.getParameterValues("aid"); LogUtil.i("upgroup----->共有"+a.length+"个aid"); int state = Integer.valueOf(s); int id = Integer.valueOf(g); int[] gid = {Integer.valueOf(g)}; Timestamp reworkdate = new Timestamp(System.currentTimeMillis()); group = new Group_Entity(id, name, null, reworkdate, state); igroup.delGroupAuthority(gid); boolean flse = igroup.upGroup(group); if(flse){ int[] aid = new int[a.length]; for(int i=0; i<a.length; i++){ aid[i] = Integer.valueOf(a[i]); } igroup.addGroupAuthority(name, aid); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=groupList&method=showgrouplist"); } else if("delgroup".equals(method)) { String[] g = req.getParameterValues("gid"); if(g != null ){ LogUtil.i("delgroup------->选中"+g.length+"个"); int[] gid = new int[g.length]; for(int j=0; j<g.length; j++){ gid[j] = Integer.valueOf(g[j]); } igroup.delGroup(gid); igroup.delGroupAuthority(gid); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=groupList&method=showgrouplist"); } } /** * 权限列表 * @param req * @param resp * @throws ServletException * @throws IOException */ public void authorityList(HttpServletRequest req,HttpServletResponse resp) throws ServletException,IOException{ IAuthority_DAO iauthority = new Authority_DAOImpl(); Authority_Entity authority = null; Authority_Entity oneauthority = null; Authority_Entity twoauthority = null; String method = req.getParameter("method"); if("showauthoritylist".equals(method)) { String p = req.getParameter("page"); String s = req.getParameter("size"); int page = p == null ? 1 : Integer.valueOf(p); int size = s == null ? 50 : Integer.valueOf(s); List<Authority_Entity> oneauthoritys = iauthority.getPageAuthority(0, page, size); for(int o=0; o<oneauthoritys.size(); o++){ oneauthority = oneauthoritys.get(o); List<Authority_Entity> twoauthoritys = iauthority.getPageAuthority(oneauthority.getId(), 1, 100); oneauthority.setList(twoauthoritys); for(int t=0; t<oneauthority.getList().size(); t++){ twoauthority = oneauthority.getList().get(t); List<Authority_Entity> threeauthoritys = iauthority.getPageAuthority(twoauthority.getId(), 1, 100); twoauthority.setList(threeauthoritys); } } int count = iauthority.getCountAuthority(); if(oneauthoritys.size() >= 1){ req.setAttribute("oneauthoritys", oneauthoritys); req.setAttribute("page", page); req.setAttribute("size", size); req.setAttribute("count", count); req.getRequestDispatcher("/WEB-INF/admin/authoritylist.jsp").forward(req, resp); }else{ req.setAttribute("authoritylist", "no data"); req.getRequestDispatcher("/WEB-INF/admin/authoritylist.jsp").forward(req, resp); } } else if("addauthority".equals(method)) { String name = req.getParameter("name"); String description = req.getParameter("description"); String myforms = req.getParameter("myforms"); String methods = req.getParameter("methods"); String path = req.getParameter("path"); String s = req.getParameter("state"); String p = req.getParameter("pid"); int pid = p == null ? 0 : Integer.valueOf(p); int state = Integer.valueOf(s); Timestamp createdate = new Timestamp(System.currentTimeMillis()); authority = new Authority_Entity(null, name, description, myforms, methods, path, createdate, null, state, pid); iauthority.addAuthority(authority); resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=authorityList&method=showauthoritylist"); } else if("showaddauthority".equals(method)) { String a = req.getParameter("aid"); if(a != null){ int id = Integer.valueOf(a); authority = iauthority.upAuthorityById(id); } List<Authority_Entity> oneauthoritys = iauthority.getPageAuthority(0, 1, 100); for(int o=0; o<oneauthoritys.size(); o++){ oneauthority = oneauthoritys.get(o); List<Authority_Entity> twoauthoritys = iauthority.getPageAuthority(oneauthority.getId(), 1, 100); oneauthority.setList(twoauthoritys); for(int t=0; t<oneauthority.getList().size(); t++){ twoauthority = oneauthority.getList().get(t); List<Authority_Entity> threeauthoritys = iauthority.getPageAuthority(twoauthority.getId(), 1, 100); twoauthority.setList(threeauthoritys); } } req.setAttribute("authority", authority); req.setAttribute("oneauthoritys", oneauthoritys); req.getRequestDispatcher("/WEB-INF/admin/authorityinfo.jsp").forward(req, resp); } else if("upauthority".equals(method)) { String a = req.getParameter("aid"); String name = req.getParameter("name"); String description = req.getParameter("description"); String myforms = req.getParameter("myforms"); String methods = req.getParameter("methods"); String path = req.getParameter("path"); String s = req.getParameter("state"); String p = req.getParameter("pid"); int state = Integer.valueOf(s); int id = Integer.valueOf(a); int pid = Integer.valueOf(p); Timestamp reworkdate = new Timestamp(System.currentTimeMillis()); authority = new Authority_Entity(id, name, description, myforms, methods, path, null, reworkdate, state, pid); iauthority.upAuthority(authority); resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=authorityList&method=showauthoritylist"); } else if("delauthority".equals(method)) { String[] a = req.getParameterValues("aid"); if(a != null ){ LogUtil.i("delauthority------->选中"+a.length+"个"); int[] id = new int[a.length]; for(int j=0; j<a.length; j++){ id[j] = Integer.valueOf(a[j]); } iauthority.delAuthority(id); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=authorityList&method=showauthoritylist"); } } /** * 商品列表 * @param req * @param resp * @throws ServletException * @throws IOException */ @SuppressWarnings("static-access") public void commodityList(HttpServletRequest req,HttpServletResponse resp) throws ServletException,IOException{ ICommodity_DAO icommodity = null; Commodity_Entity commodity = null; List<Commodity_Entity> commoditys = null; Map<String,String> myMap = null; String method = req.getParameter("method"); LogUtil.i("commodityList---->method="+this.method); if("showcommoditylist".equals(method)) { String p = req.getParameter("page"); String s = req.getParameter("size"); int page = p == null ? 1 : Integer.valueOf(p); int size = s == null ? 5 : Integer.valueOf(s); icommodity = new Commodity_DAOImpl(); commoditys = icommodity.getPageCommodity(page, size); int count = icommodity.getCountCommodity(); if(commoditys.size() >= 1){ req.setAttribute("commoditys", commoditys); req.setAttribute("page", page); req.setAttribute("size", size); req.setAttribute("count", count); req.getRequestDispatcher("/WEB-INF/admin/commoditylist.jsp").forward(req, resp); }else{ req.setAttribute("grouplist", "no data"); req.getRequestDispatcher("/WEB-INF/admin/commoditylist.jsp").forward(req, resp); } } else if("showaddcommodity".equals(method)) { String c = req.getParameter("cid"); if(c != null){ int cid = Integer.valueOf(c); icommodity = new Commodity_DAOImpl(); commodity = icommodity.upCommodityById(cid); myMap = new HashMap<String, String>(); CommodityClass_Entity commodityclass = icommodity.getCommodityCommodityClassById(cid); if(commodityclass != null){ myMap.put(commodityclass.getId() +"", ""); } } ICommodityClass_DAO icommodityclass = new CommodityClass_DAOImpl(); List<CommodityClass_Entity> commodityclasss = icommodityclass.getPageCommodityClass(0, 1, 100); for(int o=0; o<commodityclasss.size(); o++){ CommodityClass_Entity onecommodityclass = commodityclasss.get(o); List<CommodityClass_Entity> twocommodityclasss = icommodityclass.getPageCommodityClass(onecommodityclass.getId(), 1, 100); onecommodityclass.setList(twocommodityclasss); for(int t=0; t<onecommodityclass.getList().size(); t++){ CommodityClass_Entity twocommodityclass = onecommodityclass.getList().get(t); List<CommodityClass_Entity> threecommodityclasss = icommodityclass.getPageCommodityClass(twocommodityclass.getId(), 1, 100); twocommodityclass.setList(threecommodityclasss); } } req.setAttribute("myMap", myMap); req.setAttribute("commodity", commodity); req.setAttribute("commodityclasss", commodityclasss); req.getRequestDispatcher("/WEB-INF/admin/commodityinfo.jsp").forward(req, resp); } else if("addcommodity".equals(this.method)) { String num = this.myFLMap.get("number"); String name = this.myFLMap.get("name"); String path = this.myFLMap.get("p_w_picpathname"); if(path != null && !"".equals(path)) path = "/p_w_picpaths/"+this.datename + this.myFLMap.get("p_w_picpathname"); String p = this.myFLMap.get("price"); String link = this.myFLMap.get("link"); String s = this.myFLMap.get("state"); String cc = this.myFLMap.get("ccid"); int number = Integer.valueOf(num); float price = Float.valueOf(p); int state = Integer.valueOf(s); int ccid = Integer.valueOf(cc); LogUtil.i("mumber="+num+"\n name="+name+"\n path="+path+"\n price="+p+"\n link="+link+"\n state="+s+"\n ccid="+cc); Timestamp createdate = new Timestamp(System.currentTimeMillis()); icommodity = new Commodity_DAOImpl(); commodity = new Commodity_Entity(null, number, name, price, path, link, createdate, null, state); boolean flse = icommodity.addCommodity(commodity); if(flse){ icommodity.addCommodityCommodityClass(number, ccid); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=commodityList&method=showcommoditylist"); } else if("upcommodity".equals(this.method)) { String ci = this.myFLMap.get("cid"); String num = this.myFLMap.get("number"); String name = this.myFLMap.get("name"); String path = this.myFLMap.get("p_w_picpathname"); if(path != null )path = "/p_w_picpaths/"+this.datename + this.myFLMap.get("p_w_picpathname"); String p = this.myFLMap.get("price"); String link = this.myFLMap.get("link"); String s = this.myFLMap.get("state"); String cc = this.myFLMap.get("ccid"); int number = Integer.valueOf(num); float price = Float.valueOf(p); int state = Integer.valueOf(s); int cid = Integer.valueOf(ci); int ccid = Integer.valueOf(cc); Timestamp reworkdate = new Timestamp(System.currentTimeMillis()); icommodity = new Commodity_DAOImpl(); commodity = icommodity.upCommodityById(cid); String newpath = req.getSession().getServletContext().getRealPath("/") + commodity.getPath(); DeleteFileUtil.deleteFilePath(newpath, null); commodity = new Commodity_Entity(cid, number, name, price, path, link, null, reworkdate, state); boolean flse = icommodity.upCommodity(commodity); if(flse){ int[] newcid = {cid}; icommodity.delCommodityCommodityClass(newcid); icommodity.addCommodityCommodityClass(number, ccid); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=commodityList&method=showcommoditylist"); } else if("delcommodity".equals(this.method)) { String[] c = req.getParameterValues("cid"); if(c != null){ int[] cid = new int[c.length]; for(int i=0; i<c.length; i++){ cid[i] = Integer.valueOf(c[i]); } icommodity = new Commodity_DAOImpl(); String[] p = icommodity.getCommodityById(cid); String[] paths = new String[p.length]; for(int i=0; i<paths.length; i++){ paths[i] = req.getSession().getServletContext().getRealPath("/") + p[i]; } DeleteFileUtil.deleteFilePath(null, paths); icommodity.delCommodity(cid); icommodity.delCommodityCommodityClass(cid); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=commodityList&method=showcommoditylist"); } } /** * 商品分类列表 * @param req * @param resp * @throws ServletException * @throws IOException * @throws JSONException */ @SuppressWarnings("static-access") public void commodityClassList(HttpServletRequest req,HttpServletResponse resp) throws ServletException,IOException, JSONException{ ICommodityClass_DAO icommodityclass = null; CommodityClass_Entity commodityclass = null; CommodityClass_Entity onecommodityclass = null; CommodityClass_Entity twocommodityclass = null; String method = req.getParameter("method"); if("showcommodityclasslist".equals(method)) { String p = req.getParameter("page"); String s = req.getParameter("size"); int page = p == null ? 1 : Integer.valueOf(p); int size = s == null ? 50 : Integer.valueOf(s); icommodityclass = new CommodityClass_DAOImpl(); List<CommodityClass_Entity> commodityclasss = icommodityclass.getPageCommodityClass(0, page, size); for(int o=0; o<commodityclasss.size(); o++){ onecommodityclass = commodityclasss.get(o); List<CommodityClass_Entity> twocommodityclasss = icommodityclass.getPageCommodityClass(onecommodityclass.getId(), 1, 100); onecommodityclass.setList(twocommodityclasss); for(int t=0; t<onecommodityclass.getList().size(); t++){ twocommodityclass = onecommodityclass.getList().get(t); List<CommodityClass_Entity> threecommodityclasss = icommodityclass.getPageCommodityClass(twocommodityclass.getId(), 1, 100); twocommodityclass.setList(threecommodityclasss); } } int count = icommodityclass.getCountCommodityClass(); if(commodityclasss.size() >= 1){ req.setAttribute("commodityclasss", commodityclasss); req.setAttribute("page", page); req.setAttribute("size", size); req.setAttribute("count", count); req.getRequestDispatcher("/WEB-INF/admin/commodityclasslist.jsp").forward(req, resp); }else{ req.setAttribute("commodityclassslist", "no data"); req.getRequestDispatcher("/WEB-INF/admin/commodityclasslist.jsp").forward(req, resp); } } else if("addcommodityclass".equals(this.method)) { String name = this.myFLMap.get("name"); String path = this.myFLMap.get("p_w_picpathname"); if(path != null && !"".equals(path)) path = "/p_w_picpaths/"+this.datename + this.myFLMap.get("p_w_picpathname"); String s = this.myFLMap.get("state"); String p = this.myFLMap.get("pid"); int pid = p == null ? 0 : Integer.valueOf(p); int state = Integer.valueOf(s); icommodityclass = new CommodityClass_DAOImpl(); Timestamp createdate = new Timestamp(System.currentTimeMillis()); commodityclass = new CommodityClass_Entity(null, name, path, createdate, null, state, pid); icommodityclass.addCommodityClass(commodityclass); resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=commodityClassList&method=showcommodityclasslist"); } else if("showaddcommodityclass".equals(this.method)) { String cc = req.getParameter("ccid"); if(cc != null){ LogUtil.i("showaddcommodityclass ---->ccid="+cc); int id = Integer.valueOf(cc); icommodityclass = new CommodityClass_DAOImpl(); commodityclass = icommodityclass.upCommodityClassById(id); } icommodityclass = new CommodityClass_DAOImpl(); List<CommodityClass_Entity> commodityclasss = icommodityclass.getPageCommodityClass(0, 1, 100); for(int o=0; o<commodityclasss.size(); o++){ onecommodityclass = commodityclasss.get(o); List<CommodityClass_Entity> twocommodityclasss = icommodityclass.getPageCommodityClass(onecommodityclass.getId(), 1, 100); onecommodityclass.setList(twocommodityclasss); for(int t=0; t<onecommodityclass.getList().size(); t++){ twocommodityclass = onecommodityclass.getList().get(t); List<CommodityClass_Entity> threecommodityclasss = icommodityclass.getPageCommodityClass(twocommodityclass.getId(), 1, 100); twocommodityclass.setList(threecommodityclasss); } } req.setAttribute("commodityclass", commodityclass); req.setAttribute("commodityclasss", commodityclasss); req.getRequestDispatcher("/WEB-INF/admin/commodityclassinfo.jsp").forward(req, resp); } else if("upcommodityclass".equals(this.method)) { String cc = this.myFLMap.get("ccid"); String name = this.myFLMap.get("name"); String path = this.myFLMap.get("p_w_picpathname"); if(path != null && !"".equals(path)) path = "/p_w_picpaths/"+this.datename + this.myFLMap.get("p_w_picpathname"); String s = this.myFLMap.get("state"); String p = this.myFLMap.get("pid"); int state = Integer.valueOf(s); int id = Integer.valueOf(cc); int pid = p == null ? 0 : Integer.valueOf(p); icommodityclass = new CommodityClass_DAOImpl(); commodityclass = icommodityclass.upCommodityClassById(id); String newpath = req.getSession().getServletContext().getRealPath("/") + commodityclass.getPath(); DeleteFileUtil.deleteFilePath(newpath,null); Timestamp reworkdate = new Timestamp(System.currentTimeMillis()); commodityclass = new CommodityClass_Entity(id, name, path, null, reworkdate, state, pid); icommodityclass.upCommodityClass(commodityclass); resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=commodityClassList&method=showcommodityclasslist"); } else if("delcommodityclass".equals(this.method)) { String[] a = req.getParameterValues("ccid"); if(a != null ){ LogUtil.i("delcommodityclass------->选中"+a.length+"个"); int[] ccid = new int[a.length]; for(int j=0; j<a.length; j++){ ccid[j] = Integer.valueOf(a[j]); } icommodityclass = new CommodityClass_DAOImpl(); String[] p = icommodityclass.getCommodityClassById(ccid); String[] paths = new String[p.length]; for(int i=0; i<p.length; i++) { paths[i] = req.getSession().getServletContext().getRealPath("/") + p[i]; } DeleteFileUtil.deleteFilePath(null, paths); icommodityclass.delCommodityClass(ccid); icommodityclass.delCommodityCommodityClassById(ccid); } resp.sendRedirect(req.getContextPath() + "/AdminServlet?myform=commodityClassList&method=showcommodityclasslist"); } else if("getcommodityclass".equals(method)) { LogUtil.i("我终于到这里了"); String cc = req.getParameter("ccid"); int ccid = cc == null ? 0 : Integer.valueOf(cc); icommodityclass = new CommodityClass_DAOImpl(); List<CommodityClass_Entity> commodityclasss = icommodityclass.getPageCommodityClass(ccid, 1, 100); JSONArray array = new JSONArray(); for(int i=0; i<commodityclasss.size(); i++) { JSONObject bje = new JSONObject(); commodityclass = commodityclasss.get(i); bje.put("id", commodityclass.getId()); bje.put("name", commodityclass.getName()); array.put(bje); } resp.setContentType("text/json"); resp.getWriter().write(array.toString()); } } }
基于Servlet_MVC模式增删改查_Servlet(4)
原创itjiandan 博主文章分类:Java Web ©著作权
文章标签 Servlet_MVC_Servlet 文章分类 前端开发
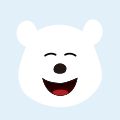
-
springboot增删改查一套Service模板
springboot一套
ide Code ci -
基于Servlet+JSP的增删改查练手项目
我们每写一步,就测试一步,不要等到所有都写好了再测试,如果都写好了再测试,最后出错的话,会很崩溃,代码量大,调试就不容易,话不多说
javaweb servlet jsp 项目 数据 -
Web对数据库的增删改查(servlet+jsp+javaBean增删改查)
Web+Java对数据库增删改查-javaWeb中级1.开始之前的准备(servlet+jsp+javaBean增删改查)2.查询3.删除4.添加5.修改5.1.进入修改
jdbc javabean jsp servlet 新星计划 -
【Java学习】JSP + Servlet + JDBC + Mysql 实现增删改查
新版本(导入eclipse教程...
jsp servlet JDBC Mysql CRUD