#!/bin/bash # ------------------------------------------------------------------------------- # filename: lamp_install.sh # version: centos6.5_32;apache2.2;mysql5.1;php5.4 # date: 2016/03/18 # author: ecithy # mail: 598779784@qq.com # website: www.xiaoleizi.com # weixingonghao 红叶IT # pre_operate: yum install -y wget gcc lrzsz epel-release unix2dos dos2unix # copyright: free # ------------------------------------------------------------------------------- check() { if [ $(id -u) != '0' ];then echo "you aren't root,stop install..."; exit 1; else echo "you are root,continue..."; fi if [ `arch` != 'i686' ];then echo "you aren't 32 system,stop install..." exit 1; else echo "you are 32 system,continue..."; fi } init() { #shutdown SELINUX setenforce 0 #remove iptables iptables -F && service iptables save test -d /usr/local/src || mkdir -p /usr/local/src } mysql_install() { cd /usr/local/src/ wget http://mirrors.sohu.com/mysql/MySQL-5.1/mysql-5.1.73-linux-i686-glibc23.tar.gz tar zxvf mysql-5.1.73-linux-i686-glibc23.tar.gz mv mysql-5.1.73-linux-i686-glibc23 /usr/local/mysql useradd -s /sbin/nologin mysql mkdir -p /data/mysql; chown -R mysql /data/mysql cd /usr/local/mysql/ ./scripts/mysql_install_db --user=mysql --datadir=/data/mysql if [ $? -ne 0 ];then echo "MySQL install fail, stop..." exit 1 fi cp /usr/local/mysql/support-files/my-large.cnf /etc/my.cnf cp /usr/local/mysql/support-files/mysql.server /etc/init.d/mysqld chmod 755 /etc/init.d/mysqld sed -ir 's#basedir=#basedir=/usr/local/mysql#g' /etc/init.d/mysqld sed -ir 's#datadir=#datadir=/data/mysql#g' /etc/init.d/mysqld chkconfig --add mysqld;chkconfig mysqld on;/etc/init.d/mysqld start if [ $? -ne 0 ];then echo "MySQL install fail, stop..." exit 1 fi } apache_install() { cd /usr/local/src/ yum install -y pcre pcre-devel apr apr-devel zlib-devel wget http://mirrors.cnnic.cn/apache/httpd/httpd-2.2.31.tar.gz tar zxvf httpd-2.2.31.tar.gz cd httpd-2.2.31 ./configure \ --prefix=/usr/local/apache \ --with-included-apr \ --enable-so \ --enable-deflate=shared \ --enable-expires=shared \ --enable-rewrite=shared \ --with-pcre if [ $? -ne 0 ];then echo "apache configure fail , stop... " exit 1 fi make && make install if [ $? -ne 0 ];then echo "apache make fail , stop... " exit 1 fi sed -i 's#\#ServerName www.example.com:80#ServerName localhost:80#g' /usr/local/apache/conf/httpd.conf #check apache syntax /usr/local/apache/bin/apachectl -t /usr/local/apache/bin/apachectl restart #check apache install succeed or not curl localhost if [ $? -eq 0 ];then echo "apache started OK,continue... " else echo "apachec started fail,top..." exit 1 fi } php_install() { cd /usr/local/src/ yum install -y epel-release libjpeg libjpeg-devel libpng libpng-devel freetype freetype-devel yum install -y bzip2 bzip2-devel openssl openssl-devel libmcrypt-devel libxml2-devel wget http://cn2.php.net/get/php-5.4.45.tar.bz2/from/this/mirror mv mirror php-5.4.45.tar.bz2 tar jxvf php-5.4.45.tar.bz2 cd php-5.4.45 ./configure \ --prefix=/usr/local/php \ --with-apxs2=/usr/local/apache/bin/apxs \ --with-config-file-path=/usr/local/php/etc \ --with-mysql=/usr/local/mysql \ --with-libxml-dir \ --with-gd \ --with-jpeg-dir \ --with-png-dir \ --with-freetype-dir \ --with-iconv-dir \ --with-zlib-dir \ --with-bz2 \ --with-openssl \ --with-mcrypt \ --enable-soap \ --enable-gd-native-ttf \ --enable-mbstring \ --enable-sockets \ --enable-exif \ --disable-ipv6 if [ $? -ne 0 ];then echo "php configure fail , stop... " exit 1 fi make && make install if [ $? -ne 0 ];then echo "php make fail , stop... " exit 1 fi test -d /usr/local/php/etc/ || mkdir /usr/local/php/etc/ cp php.ini-production /usr/local/php/etc/php.ini } apacche_resolve_php() { #support resilve php sed -i -e 's#AddType application/x-gzip .gz .tgz#&\n AddType application/x-httpd-php .php#g' /usr/local/apache/conf/httpd.conf sed -i 's#DirectoryIndex index.html#& index.htm index.php#g' /usr/local/apache/conf/httpd.conf #set apachectl as global variable echo -e 'PATH=$PATH:/usr/local/mysql/bin:/usr/local/apache/bin\nexport PATH' >/etc/profile.d/path.sh; source /etc/profile apachectl -t apachectl restart cat > /usr/local/apache/htdocs/index.php<<EOF <?php phpinfo(); ?> EOF curl localhost && echo "php resolve OK" || echo "php resolve fail" } lamp_install() { check init mysql_install apache_install php_install apacche_resolve_php } lamp_install 2>&1 | tee ~/install.log
LAMP一键安装脚本(编译方式)
精选 原创
©著作权归作者所有:来自51CTO博客作者ecithy的原创作品,请联系作者获取转载授权,否则将追究法律责任
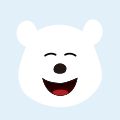
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章