import os
import os.path
import time
#简单读取
# f = open('file.txt','r',encoding='utf-8')
#
# txt = f.read()
#
# print(txt)
#
# f.close()
#
# print(txt)
#防止异常读取
# f = ''
# try:
# f = open('file.txt', 'r', encoding='utf-8')
#
# print(f.read())
#
# finally:
# print('>>>>>>finally')
# if f:
# f.close()
#获取当前文件路径
# nowPath = os.getcwd()
# print(nowPath)
#获取当前文件的父路径 结果:这里返回的是根目录
# fatherPath = os.path.abspath('../../../')
# print(fatherPath)
#返回绝对路径根目录 结果:c:\
# p = os.path.dirname('c:\csv\test.csv')
# print(p)
#返回绝对路径的文件名 结果:test.csv
# p = os.path.basename('c:\csv\\test.csv')
# print(p)
#判断文件是否存在 我的有这个文件返回的是:true
# path = os.getcwd()
# p = os.path.exists(path + '\\file.txt')
#判断目录是否存在,如果路径最后面有 \ 则是 false :true
# path = os.getcwd()
# dir = os.path.isdir(path)
#拼接路径,第一个绝对路径之前的参数将被忽略 结果:F:\python\file.txt
# path = os.path.join('F:\\','python','file.txt')
# print(path)
#格式化路径 F:/css\mian.css 结果:F:\css\mian.css
# path = os.path.normpath('F:/css\mian.css')
# print(path)
#分离文件和文件名 默认返回的是元祖 结果:('F:\\css\\mian', '.css')
# p = os.path.splitext('F:\css\mian.css')
# print(p)
#获取文件大小 结果:41
# p = os.path.getsize(os.getcwd() + '\\file.txt')
# print(p)
#获取最后存取时间
p = os.path.getatime(os.getcwd() + '\\file.txt')
print(p)
#获取最后修改时间并将时间转化为指定格式
# p = os.path.getmtime(os.getcwd() + '\\file.txt')
#
# times = time.localtime(p)
#
# format_time = time.strftime("%Y-%m-%d %H:%M:%S",times)
#
# print(format_time)
python常用文件操作方法
原创
©著作权归作者所有:来自51CTO博客作者小何博客的原创作品,请联系作者获取转载授权,否则将追究法律责任
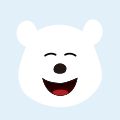
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
canvas常用操作方法
canvas常用操作方法
Data github NPM -
Python 集合(set)常用操作方法
集合是0个或多个对象引用的无序组合,这些对
对象引用 python 常用操作 -
Asp.Net常用文件操作方法
常用文件操作方法
asp net 文件操作 -
.net xml常用操作方法
using System;using System.Xml;using System.Xml.Xsl;using System.Xml.XPath;usin
xml .net string list class