c primer plus(第五版)读书笔计 第七章(4)
原创IT业民工 博主文章分类:c primer plus ©著作权
文章标签 c primer plus(第五版)读书 文章分类 后端开发
©著作权归作者所有:来自51CTO博客作者IT业民工的原创作品,请联系作者获取转载授权,否则将追究法律责任
一个统计字数的程序
//7-7.c -- 统计字符,单词和行
#include <stdio.h>
#include <ctype.h>
#define STOP '|'
int main (void)
{
char c;
char prev;
long n_chars = 0l;
int n_lines = 0;
int n_words = 0;
int p_lines = 0;
int inword = 0;
printf ("Enter text to be analyzed (| to terminate ):\n");
prev = '\n';
while ((c = getchar ()) != STOP)
{
n_chars ++;
if (c == '\n')
n_lines ++;
if (!isspace (c) && !inword)
{
inword = 1 ;
n_words++;
}
if (isspace (c) && !inword)
inword = 0;
prev = c;
}
if (prev != '\n')
p_lines = 1;
printf ("charcters = %ld,words = %d,lines = %d,",n_chars,n_words,n_lines);
printf("partial lins = %d\n",p_lines);
return 0 ;
}
条件运算符?
C提供一种简写的方式来表示if else 语句的一种形式,这被称为条件表达式,并使用条件运算符(?:)。这是个有三个运算符分两部分的运算符。它的一般形式:
expression1 ? expression2 : expression3
如果expression1为真,整个条件表达式的值和expression2的值相同。如果expression1为假整个表达式的值等于expression3的值。
//7-8.c -- 使用条件运算符
#include <stdio.h>
#define COVERAGE 200
int main (void)
{
int sq_feet;
int cans;
printf ("Enter number of square feet to be painted:\n");
while (scanf("%d",&sq_feet ) == 1)
{
cans = sq_feet / COVERAGE;
//printf ("%.f",cans);
cans += ((sq_feet % COVERAGE == 0)) ? 0 : 1;
printf ("You need %d %s of paint .\n",cans,cans == 1 ? "can":"cans");
printf ("Enter next value (q to quit):\n");
}
return 0 ;
}
因为程序使用了int 类型所以除法被截断,也就是说215/200的结果为1。因此cans 被四舍五入为整数部分,如果sq_feet % COVERAGE == 0那么cans值不变否则有余数所以加上1
cans += ((sq_feet % COVERAGE == 0)) ? 0 : 1;
循环辅助手段:continue 和break
这两个语句可以根据循环体内进行判断结果来忽略部分循环甚至终止它
//7-9.c 使用continue 跳过部分循环
#include <stdio.h>
int main (void)
{
const float MIN = 0.0f;
const float MAX = 100.0f;
float score ;
float total = 0.0f;
int n = 0;
float min = MIN;
float max = MAX;
printf ("Enter the first score(q to quit):");
while (scanf ("%f",&score) == 1)
{
if (score < MIN || score > MAX)
{
printf ("%0.1f is an invalid value .Try again:",score);
continue;
}
printf ("Accepting %0.1f :\n",score);
min = (score < min) ? score : min;
max = (score > max) ? score : max;
total += score;
n++;
printf ("Enter next score (q to quit ):");
}
if (n > 0)
{
printf ("Average of %d score is %0.1f.\n",n ,total / n);
printf ("Low = %0.1f,high = %0.1f\n",min,max);
}
else
printf ("NO valid scores were entered.\n");
return 0 ;
}
程序说明:while 循环读取输入内容,直到输入一个非数字的数据,循环里的if 筛选出无效的分数值,当输入的值不在0-100之间,那么他printf ("%0.1f is an invalid value .Try again:",score); 然后continue 语句导致程序跳过循环其余的用于处理有效输入的部分,程序进入下一个循环周期,试图读取下一个输入值。
在这种情况下使用continue 的好处为:可以增加程序的可读性,另个一个好处是作为占位符用。在什么地方继续循环:在while 和do while循环中continue 语句之后发生的动作为求循环判断表达式的值。而对于for则首先更新表达式的值然后再求循环判断表达式的值。
Break语句
循环中break语句导致程序终止包含它的循环,并进行程序的下一阶段,它同continue语句相同的地方是,它们在嵌套循环里,都只影响包含它最里层的循环。它们的区别:
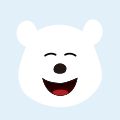
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章