- class TestDelegate
- {
- public delegate void EventHanding();
- public event EventHanding OnFireEvnet;
- protected void RegistEvent()
- {
- Delegate d1 = new EventHanding(test1);
- Delegate d2 = new EventHanding(test2);
- Delegate d3 = new EventHanding(test3);
- Delegate d4 = new EventHanding(test4);
- Delegate newEvent1 = Delegate.Combine(d1, d2);
- Delegate newEvent2 = Delegate.Combine(d3, d4);
- //新合并的委托是一个新的对象
- if (Object.ReferenceEquals(d2, newEvent1))
- {
- string str = string.Empty;
- }
- if (d2.Method.ToString().CompareTo(newEvent1.Method.ToString())==0)
- {
- string str = string.Empty;
- }
- EventHanding newEvent = (EventHanding)Delegate.Combine(newEvent1, newEvent2);
- OnFireEvnet += newEvent;
- }
- protected void FireEvent()
- {
- //单波
- MulticastDelegate del = (MulticastDelegate)OnFireEvnet;
- del.Method.Invoke(this, null);
- //多波
- OnFireEvnet();
- }
- int i = 0;
- public void test1()
- {
- i++;
- }
- public void test2()
- {
- i++;
- }
- public void test3()
- {
- i++;
- }
- public void test4()
- {
- i++;
- }
- public static void Test()
- {
- TestDelegate test = new TestDelegate();
- test.RegistEvent();
- test.FireEvent();
- }
- }
Delegate 与 MulticastDelegate 委托的单波与多波模式
原创
©著作权归作者所有:来自51CTO博客作者skydxd的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:连接字符串配置
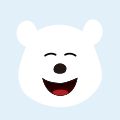
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
委托delegate编程语言 编程
-
C#多播委托(Multicast Delegate)
委托可以包含多个方法,这种委托称为多播委托。如果调用多播委托,就可以按顺序连续调用多个方法。为此,委托的签名必
c# 多播委托 方法调用 运算符 调用方法