/*---------------------------------------------------------
Title: Link Stack(链栈) 链栈-链式存储结构的栈
请先阅读教材67页, 2.3.2,2.3.4节, 栈的定义及基本运算
(注意以下程序为简化后的,仅供入门学习之用)
----------------------------------------------------------*/
#include<stdio.h>
#include<stdlib.h>
//定义栈的结点结构
struct stacknode
{
int data;//存放数据元素
struct stacknode * next;//指针,指向下一个结点
};
//初始化栈
struct stacknode * InitialStack()
{
struct stacknode * head;
head=(struct stacknode *)malloc(sizeof(struct stacknode ));
head->next=NULL;//
return head;
}
//入栈
void PushIntoStack(struct stacknode * head, int value)
{
struct stacknode * p;
p=(struct stacknode *)malloc(sizeof(struct stacknode ));
p->data=value;
p->next=head->next;
head->next=p;
}
//出栈
void PopFromStack(struct stacknode * head)
{
struct stacknode * p;
if(head->next==NULL)
printf("Pop Failed \n");
else
{
p=head->next;
head->next=p->next;
free(p);
}
}
//显示栈中所有元素
void ShowStack(struct stacknode * head)
{
struct stacknode * p;
p=head->next;
printf("\nShow all elements in stack:\n");
while(p != NULL)
{
printf(" %d ",p->data);
p=p->next;
}
}
void main()
{
struct stacknode * head1;
head1=InitialStack();
ShowStack(head1);
PushIntoStack(head1,11);
ShowStack(head1);
PushIntoStack(head1,22);
ShowStack(head1);
PopFromStack(head1);
ShowStack(head1);
}
2008秋-链栈-链式存储结构的栈
原创
©著作权归作者所有:来自51CTO博客作者emanlee的原创作品,请联系作者获取转载授权,否则将追究法律责任
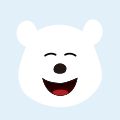
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
一场关于栈的面试----最小栈的实现
一场关于栈的面试----最小栈的实现
最小值 出栈 入栈 -
【数据结构】C语言实现顺序栈
【数据结构】第三章——栈、队列和数组详细介绍通过C语言实现顺序栈
数据结构 C语言 顺序栈 -
链栈-----栈的链式存储结构及其实现
栈的链式存储
数据结构 栈 链式 -
栈的链式存储java 栈的链式存储定义
链栈结构的定义:链栈即栈的链式存储,这里用带头结点的单链表实现链栈
栈的链式存储java 数据结构 出栈 结点 初始化