/*
* 123.c
*
* Created on: 2013-6-3
* Author: Administrator
*/
#include<reg52.h>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
typedef bit BOOL;
sbit DQ = P3 ^ 7;
uchar Temp_Value[] = { 0x00, 0x00 };
uchar Display_Digit[] = { 0, 0, 0, 0 };
sbit LCD_RW = P2 ^ 5; //写数据?
sbit LCD_RS = P2 ^ 6;
sbit LCD_EP = P2 ^ 7; //使能?
uchar num;
int temper = 0;
int CurrentT = 0;
bit flag;
void delay(uchar t) {
while (t--)
;
}
/*
void delay2(uint z)
{
uint x,y;
for(x=z;x>0;x--)
for(y=110;y>0;y--){;}
}
*/
void init_ds18b20() {
// uchar n;
DQ = 1;
_nop_();
DQ = 0;
delay(80);
_nop_();
DQ = 1;
delay(14);
_nop_();
_nop_();
_nop_();
if (DQ == 0)
flag = 1; //detect 1820 success!
else
flag = 0; //detect 1820 fail!
delay(20); //20
_nop_();
_nop_();
DQ = 1;
}
void write_byte(uchar dat) {
uchar i;
for (i = 0; i < 8; i++) {
DQ = 0;
_nop_();
DQ = dat & 0x01; //从最低位开始写
delay(3);
_nop_();
_nop_();
DQ = 1;
dat >>= 1;
}
// delay(4);
}
uchar read_byte() {
uchar i, value = 0;
for (i = 0; i < 8; i++) {
DQ = 0;
value >>= 1;
DQ = 1;
if (DQ)
value |= 0x80;
delay(2);
_nop_();
}
return value;
}
int readtemperature() {
uchar templ, temph;
// int temp;
init_ds18b20();
write_byte(0xcc); //跳过ROM
write_byte(0x44); //启动温度测量
delay(300);
init_ds18b20();
write_byte(0xcc);
write_byte(0xbe);
// Temp_Value[0] = ReadOneByte();
// Temp_Value[1] = ReadOneByte();
templ = read_byte();
temph = read_byte();
//CurrentT = ((templ&0xf0)>>4) | ((temph&0x07)<<4);
CurrentT = temph*256 +templ;
CurrentT = CurrentT * 62.5;
return CurrentT;
}
/****************侧忙函数************************/
BOOL lcd_bz() {
BOOL result;
LCD_RS = 0;
LCD_RW = 1;
LCD_EP = 1;
_nop_();
_nop_();
_nop_();
_nop_();
result = (BOOL) (P0 & 0x80); //检测P0最高位是否为1
LCD_EP = 0;
return result;
}
/****************写命令函数************************/
void write_com(uchar cmd) {
while (lcd_bz())
;
LCD_RS = 0;
LCD_RW = 0;
// LCD_EP = 0;
_nop_();
_nop_();
P0 = cmd;
_nop_();
_nop_();
_nop_();
_nop_();
LCD_EP = 1;
_nop_();
_nop_();
_nop_();
_nop_();
LCD_EP = 0;
}
void lcd_pos(uchar pos) {
write_com(pos | 0x80);
}
/****************写数据函数************************/
void write_data(uchar dat) { //写入字符显示数据到LCD
while (lcd_bz())
;
LCD_RS = 1;
LCD_RW = 0;
// LCD_EP = 0;
_nop_();
_nop_();
P0 = dat;
_nop_();
_nop_();
_nop_();
_nop_();
LCD_EP = 1;
_nop_();
_nop_();
_nop_();
_nop_();
LCD_EP = 0;
}
void init() {
// dula=0;
// wela=0;
LCD_EP = 0;
write_com(0x38); //16*2显示
write_com(0x0e); //开显示;显示光标,光标不闪烁
write_com(0x06); //光标自动增加111
write_com(0x01); //显示清屏
// write_com(0x80+0x10);
}
void main() {
init();
while (1) {
temper = readtemperature();
lcd_pos(0);
if (temper < 0) {
write_data('-');
temper = 0 - temper;
} else
write_data('+');
lcd_pos(1);
write_data((temper) / 10000 + 0x30);
lcd_pos(2);
write_data(((temper) % 10000 )/ 1000 + 0x30);
lcd_pos(3);
write_data('.');
lcd_pos(4);
write_data(((temper) % 10000 % 1000 )/ 100 + 0x30);
lcd_pos(5);
write_data(((temper) %10000 %1000 % 100 )/10 + 0x30);
lcd_pos(6);
write_data((temper) %10000 %1000 % 100 % 10 + 0x30);
lcd_pos(7);
write_data(0xdf);
lcd_pos(8);
write_data('C');
}
}
温度计(LCD显示)
转载上一篇:SQL语句快速介绍
下一篇:基于单片机的生日快乐歌
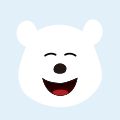
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
用arduinoUNO做温度计
用arduinoUNO测量电烙铁的温度。
Arduino 电路