import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.*;
public class LocalTimeTest {
private static Map<String, String> timeZoneIds = Map.of("NRT", "Asia/Tokyo", "BKK", "Asia/Bangkok", "ICN", "Asia/Seoul", "HNL", "Pacific/Honolulu", "SIN", "Asia/Singapore", "TPE", "Asia/Taipei", "LAX", "America/Los_Angeles");
public static void main(String[] args) throws ParseException {
System.out.println(getLocalTime("2022-11-05T17:30:00.000Z"));
System.out.println(getLocalTime("2022-11-06T17:30:00.000Z"));
}
private static String getLocalTime(String gmtTime) throws ParseException {
final DateFormat formatter = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss.SSS'Z'");
formatter.setTimeZone(TimeZone.getTimeZone("GMT"));
final Date timezone = formatter.parse(gmtTime);
formatter.setTimeZone(TimeZone.getTimeZone(timeZoneIds.get("LAX")));
return formatter.format(timezone);
}
}
java:GMT转本地时间
原创
©著作权归作者所有:来自51CTO博客作者呜哈哈666888的原创作品,请联系作者获取转载授权,否则将追究法律责任
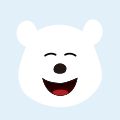
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章