#include "stdio.h"
#include "pthread.h"
#include "stdlib.h"
#include "string.h"
struct test
{
int num;
struct test *next;
};
struct test *head;
pthread_cond_t ok=PTHREAD_COND_INITIALIZER;
pthread_mutex_t lock=PTHREAD_MUTEX_INITIALIZER;
void consume()
{
struct test *mp;
for(;;)
{
pthread_mutex_lock(&lock);
if(head == NULL)
{
printf("Consum is waiting...\n");
pthread_cond_wait(&ok,&lock);
printf("Consum leave waiting %d\n",head->num);
}
printf("Consum get the number %d\n",head->num);
mp=head;
head=head->next;
free(mp);
pthread_mutex_unlock(&lock);
sleep(rand()%5);
}
}
void produce()
{
struct test *tmp;
for(;;)
{
tmp=(struct test *)malloc(sizeof(struct test));
tmp->num=rand()%1000+1;
pthread_mutex_lock(&lock);
tmp->next=head;
head=tmp;
printf("Produce %d\n",tmp->num);
pthread_mutex_unlock(&lock);
pthread_cond_signal(&ok);
sleep(rand()%5);
}
}
int main()
{
pthread_t pid,cid;
pthread_create(&pid,NULL,produce,NULL);
pthread_create(&cid,NULL,consume,NULL);
pthread_join(pid,NULL);
pthread_join(cid,NULL);
return 0;
}
C-pthread 生产者,消费者
原创
©著作权归作者所有:来自51CTO博客作者bio_tt的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:C-sys/time
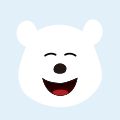
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
chan实现生产者消费者模型
一个简单的例子让你更好的理解golang chan的使用
斐波拉契数列 斐波那契数列 Group -
生产者消费者java实现 java 生产者和消费者
什么是生产者消费者模式?生产者消费者模式是程序设计中非常常见的一种设计模式,被广泛运用在解耦、消息队列等场景。在现实世界中,我们把生产商品的一方称为生产者,把消费商品的一方称为消费者,有时生产者的生产速度特别快,但消费者的消费速度跟不上,俗称“产能过剩”,又或是多个生产者对应多个消费者时,大家可能会手忙脚乱。在生产者和消费者之间就需要一个中介来进行调度,于是便诞生了生产者消费者模式使用生产者消费者
生产者消费者java实现 生产者消费者模式 BlockingQueue实现 Condition实现 wait/notify实现